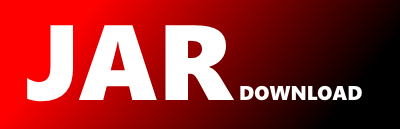
cc.protea.foundation.providers.JerseyConfiguration Maven / Gradle / Ivy
package cc.protea.foundation.providers;
import java.lang.reflect.Method;
import java.text.SimpleDateFormat;
import java.util.Date;
import javax.ws.rs.core.SecurityContext;
import org.apache.commons.lang3.StringUtils;
import org.glassfish.jersey.server.monitoring.ApplicationEvent;
import org.glassfish.jersey.server.monitoring.ApplicationEventListener;
import org.glassfish.jersey.server.monitoring.RequestEvent;
import org.glassfish.jersey.server.monitoring.RequestEventListener;
import org.slf4j.LoggerFactory;
import cc.protea.foundation.model.ProteaException;
public class JerseyConfiguration implements ApplicationEventListener {
public void onEvent(final ApplicationEvent applicationEvent) {
switch (applicationEvent.getType()) {
case INITIALIZATION_FINISHED:
LoggerFactory.getLogger(JerseyConfiguration.class).info("Jersey application started");
break;
default:
break;
}
}
public RequestEventListener onRequest(final RequestEvent requestEvent) {
return new MyRequestEventListener();
}
public static class MyRequestEventListener implements RequestEventListener {
static boolean cacheServers = false;
private volatile long methodStartTime;
public void onEvent(final RequestEvent requestEvent) {
switch (requestEvent.getType()) {
case RESOURCE_METHOD_START:
methodStartTime = System.currentTimeMillis();
break;
case FINISHED:
final String methodExecution = methodStartTime == 0 ? "" : String.format("%,6dms", System.currentTimeMillis() - methodStartTime);
final String path = requestEvent.getUriInfo().getPath();
final String verb = StringUtils.rightPad(requestEvent.getContainerRequest().getMethod(), 7);
final Method method = requestEvent.getUriInfo().getMatchedResourceMethod() == null ? null : requestEvent.getUriInfo().getMatchedResourceMethod().getInvocable().getHandlingMethod();
String methodName = method == null ? " (method not found)" : " (" + method.getDeclaringClass().getSimpleName() + "." + method.getName() + ")";
if ("OPTIONS".equals(verb)) {
methodName = "";
}
final int status = requestEvent.getContainerResponse() == null ? 0 : requestEvent.getContainerResponse().getStatus();
if (status != 0 && StringUtils.equalsIgnoreCase(verb, "OPTIONS")) {
methodName = "Access-Control-Allow-Origin: " + requestEvent.getContainerResponse().getHeaderString("Access-Control-Allow-Origin");
}
String cacheServer = " ";
String rawIp = requestEvent.getContainerRequest().getHeaderString("X-Forwarded-For");
if (StringUtils.contains(rawIp, ",")) {
rawIp = StringUtils.substringAfterLast(rawIp, ",").trim();
cacheServers = true;
cacheServer = "CACHED";
}
if (requestEvent.getContainerRequest().getHeaders().containsKey("Fastly-Client-IP")) {
cacheServers = true;
cacheServer = "FASTLY";
}
if (requestEvent.getContainerRequest().getHeaders().containsKey("CloudFront-Forwarded-Proto")) {
cacheServers = true;
cacheServer = "CLOUDF";
}
final String ipAddress = StringUtils.rightPad(StringUtils.trimToEmpty(rawIp != null ? rawIp : new SimpleDateFormat("MM/dd HH:mm:ss").format(new Date())), 15);
final String cacheInfo = cacheServers ? "" : (" " + cacheServer);
String exception = "";
if (requestEvent.getException() != null) {
Throwable cause = requestEvent.getException().getCause();
while(cause != null) { // Check all the causes for a ProteaException
if (cause instanceof ProteaException) {
exception = " - " + ((ProteaException)cause).logMessage; // If found use that log message
break;
}
cause = cause.getCause();
}
// If we don't find a protea exception use whatever message we do find
if(StringUtils.isBlank(exception)) {
exception = " - " + requestEvent.getException().getMessage();
}
}
SecurityContext securityContext = requestEvent.getContainerRequest().getSecurityContext();
String userInfo = "";
if (securityContext != null && securityContext.getUserPrincipal() != null) {
userInfo = " user:" + securityContext.getUserPrincipal().getName();
}
String referer = StringUtils.trimToEmpty(requestEvent.getContainerRequest().getHeaderString("Referer"));
if (StringUtils.isNotBlank(referer)) {
referer = " - from " + referer;
}
String s = (requestEvent.isSuccess() ? "API Success " : "API Failure ") + status + " [" + ipAddress + cacheInfo + "] " +
methodExecution + " " +
verb + " " + path + methodName +
userInfo + exception + referer;
if (requestEvent.isSuccess()) {
System.out.println(s);
} else {
System.err.println(s);
}
break;
default:
break;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy