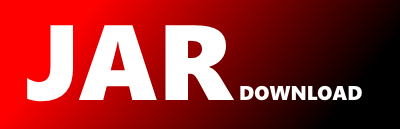
cc.protea.foundation.util.ImageUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of foundation Show documentation
Show all versions of foundation Show documentation
Integrate compatible versions of many external systems to create a REST/HTTP server foundation
The newest version!
package cc.protea.foundation.util;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.color.CMMException;
import java.awt.image.BufferedImage;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
public class ImageUtil {
static Logger log = LoggerFactory.getLogger(ImageUtil.class);
public static enum Type { PNG, JPEG }
public static BufferedImage getImage(InputStream is) throws IOException {
try {
return ImageIO.read(is);
} catch (CMMException e) {
log.error("Error loading image", e);
return null;
}
}
public static BufferedImage getImage(byte[] bytes) {
if (bytes == null || bytes.length == 0) {
return null;
}
try {
return getImage(new ByteArrayInputStream(bytes));
} catch (IOException e) {
log.error("Error loading image", e);
return null;
}
}
public static byte[] convert(byte[] inputImageBytes, Type type) {
if (type == null) {
return inputImageBytes;
}
BufferedImage image = getImage(inputImageBytes);
return convert(image, type);
}
public static byte[] convert(BufferedImage image, Type type) {
if (image == null || type == null) {
return null;
}
image = removeTransparency(image);
ByteArrayOutputStream os = new ByteArrayOutputStream();
try {
ImageIO.write(image, type.name(), os);
os.flush();
return os.toByteArray();
} catch (IOException e) {
log.error("Could not convert image to {0}", type, e);
return null;
}
}
public static BufferedImage removeTransparency(BufferedImage in) {
return removeTransparency(in, Color.WHITE);
}
public static BufferedImage removeTransparency(BufferedImage in, Color color) {
if (in == null) {
return null;
}
BufferedImage image = new BufferedImage(in.getWidth(null), in.getHeight(null), BufferedImage.TYPE_INT_RGB);
Graphics2D g = image.createGraphics();
g.setColor(color);
g.fillRect(0, 0, in.getWidth(null), in.getHeight(null));
g.drawRenderedImage(in, null);
g.dispose();
return image;
}
public static BufferedImage crop(BufferedImage in, int width, int height) {
if (in == null) {
return null;
}
int top = (in.getHeight() - height) / 2;
int bottom = top + height;
int left = (in.getWidth() - width) / 2;
int right = left + width;
return crop(in, left, top, right, bottom);
}
public static BufferedImage crop(BufferedImage img, int left, int top, int right, int bottom) {
if (img == null) {
return null;
}
int type = img.getColorModel().hasAlpha() ? BufferedImage.TYPE_4BYTE_ABGR : BufferedImage.TYPE_3BYTE_BGR;
BufferedImage out = new BufferedImage(right - left, bottom - top, type);
Graphics2D g = out.createGraphics();
g.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
g.drawImage(img, 0, 0, right - left, bottom - top, left, top, right, bottom, null);
g.dispose();
return out;
}
public static BufferedImage limit(BufferedImage img, Integer maxW, Integer maxH) {
if (img == null || (maxW == null && maxH == null)) {
return img;
}
int w = img.getWidth();
int h = img.getHeight();
if (maxW == null) {
maxW = w;
}
if (maxH == null) {
maxH = h;
}
double scaleFactor = getScaleFactor(w, h, maxW, maxH);
int newWidth = (int) Math.round(scaleFactor * w);
int newHeight = (int) Math.round(scaleFactor * h);
if (w != newWidth || h != newHeight) {
return resize(img, newWidth, newHeight);
}
return img;
}
public static BufferedImage pad(BufferedImage img, Integer width, Integer height) {
if (img == null || width == null || height == null) {
return img;
}
img = limit(img, width, height);
if (img.getWidth() == width && img.getHeight() == height) {
return img;
}
int type = img.getColorModel().hasAlpha() ? BufferedImage.TYPE_4BYTE_ABGR : BufferedImage.TYPE_3BYTE_BGR;
BufferedImage dimg = new BufferedImage(width, height, type);
Graphics2D g = dimg.createGraphics();
g.setBackground(Color.BLACK);
g.setColor(Color.BLACK);
g.fillRect(0, 0, width, height);
g.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
int left = (width - img.getWidth()) / 2;
int top = (height - img.getHeight()) / 2;
g.drawImage(img, left, top, left + img.getWidth(), top + img.getHeight(), 0, 0, img.getWidth(), img.getHeight(), null);
g.dispose();
return dimg;
}
public static BufferedImage resize(BufferedImage img, int newW, int newH) {
if (img == null) {
return null;
}
int w = img.getWidth();
int h = img.getHeight();
int type = img.getColorModel().hasAlpha() ? BufferedImage.TYPE_4BYTE_ABGR : BufferedImage.TYPE_3BYTE_BGR;
BufferedImage dimg = new BufferedImage(newW, newH, type);
Graphics2D g = dimg.createGraphics();
g.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
g.drawImage(img, 0, 0, newW, newH, 0, 0, w, h, null);
g.dispose();
return dimg;
}
static double getScaleFactor(double imageWidth, double imageHeight, double maxWidth, double maxHeight) {
double widthScale = maxWidth / (double) Math.max(1, imageWidth);
double heightScale = maxHeight / (double) Math.max(1, imageHeight);
double factor = Math.min(widthScale, heightScale);
if (factor >= 1) {
return 1;
}
return factor;
}
public static boolean isSquareish(BufferedImage img) {
if (img == null) {
return false;
}
if (img.getHeight() > (img.getWidth() * 1.1)) {
return false;
}
if (img.getHeight() < (img.getWidth() * 0.9)) {
return false;
}
return true;
}
public static boolean isPortrait(BufferedImage img) {
return (img != null && img.getWidth() < img.getHeight());
}
public static boolean isLandscape(BufferedImage img) {
return (img != null && img.getWidth() > img.getHeight());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy