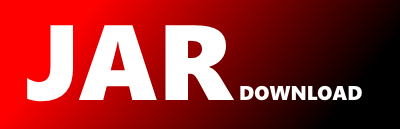
cc.redberry.combinatorics.CombinatorialIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of combinatorics Show documentation
Show all versions of combinatorics Show documentation
A number of combinatorial algorithms for enumerating (without in-memory storing) of different types of
combinations.
The newest version!
package cc.redberry.combinatorics;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
/**
* Combinatorial iterator.
*
* @author Dmitry Bolotin
* @author Stanislav Poslavsky
* @since 1.0
*/
public interface CombinatorialIterator
extends Iterator, Iterable, Serializable {
/**
* Resets the iteration
*/
void reset();
/**
* Returns the reference on the current iteration element.
*
* @return the reference on the current iteration element
*/
T current();
@Override
default Iterator iterator() {
return this;
}
/**
* Return a stream of combinations
*/
default Stream stream() { return StreamSupport.stream(this.spliterator(), false);}
/**
* Write all elements of this iterable to list
*/
default List toList() {
List list = new ArrayList<>();
for (T t : this)
list.add(t);
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy