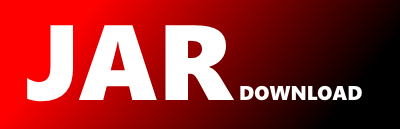
cc.redberry.graph.ContractionsGraphDrawer Maven / Gradle / Ivy
/*
* Redberry: symbolic tensor computations.
*
* Copyright (c) 2010-2012:
* Stanislav Poslavsky
* Bolotin Dmitriy
*
* This file is part of Redberry.
*
* Redberry is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Redberry is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Redberry. If not, see .
*/
package cc.redberry.graph;
import java.io.*;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
import cc.redberry.core.context.CC;
import cc.redberry.core.context.ToStringMode;
import cc.redberry.core.indices.IndicesUtils;
import cc.redberry.core.tensor.FullContractionsStructure;
import cc.redberry.core.tensor.Product;
import cc.redberry.core.tensor.Tensor;
public class ContractionsGraphDrawer {
public static final String BIN_DIR = "/usr/bin/";
public static String draw(Product product, ToStringMode mode) {
int i;
FullContractionsStructure fcs = product.getContent().getFullContractionStructure();
Tensor[] data = product.getContent().getDataCopy();
long[][] contractions = fcs.contractions;
//Same algorithm as used now in ProductsBijectionPort
Set seeds = new HashSet<>();
int[] seeds_ = new int[fcs.componentCount];
Arrays.fill(seeds_, -1);
for (i = 0; i < fcs.components.length; ++i)
if (seeds_[fcs.components[i]] == -1)
seeds.add(seeds_[fcs.components[i]] = i);
//Buiding graph
StringBuilder builder = new StringBuilder();
int freeCounter = 0;
builder.append("digraph G {");
for (i = 0; i < fcs.freeContractions.length; ++i)
builder.append("F").append(i).append(" [label=\"-\" style=\"filled\" fillcolor=\"#CCFFFF\"];");
for (i = 0; i < data.length; ++i)
builder.append("T").append(i).append(" [label=\"[").append(i).append("] ").append(data[i].toString(mode)).append("\"").append(seeds.contains(i) ? " style=\"filled\" fillcolor=\"#B6FA50\"" : "").append("];");
long contraction;
for (i = 0; i < contractions.length; ++i) {
Tensor from = data[i];
for (int j = 0; j < contractions[i].length; ++j) {
contraction = contractions[i][j];
int toIndex = FullContractionsStructure.getToTensorIndex(contraction);
//Tensor to = null;
//if (toIndex != -1)
// to = data[toIndex];
int index = from.getIndices().get(j);
String sIndex = CC.getIndexConverterManager().getSymbol(IndicesUtils.getNameWithType(index), ToStringMode.LaTeX);
if (toIndex != -1 && IndicesUtils.getState(index))
builder.append("T").append(i).append("->T").append(toIndex).append(" [label=\"").append(sIndex).append("\"]");
if (toIndex == -1)
if (IndicesUtils.getState(index))
builder.append("T").append(i).append("->F").append(freeCounter++).append(" [label=\"").append(sIndex).append("\"]");
else
builder.append("F").append(freeCounter++).append("->T").append(i).append(" [label=\"").append(sIndex).append("\"]");
}
}
builder.append("}");
return builder.toString();
}
public static void drawToFile(Product product, ToStringMode mode, String format, String address, String name) {
try {
String code = ContractionsGraphDrawer.draw(product, mode);
ProcessBuilder pb = new ProcessBuilder(BIN_DIR + "dot", "-T" + format);//("/bin/sh", "-c", "dot -Tpng");
pb.redirectErrorStream(true);
pb.redirectOutput(new File(address + name + "." + format));
Process process = pb.start();
PrintStream ps = new PrintStream(process.getOutputStream());
ps.println(code);
ps.close();
process.waitFor();
} catch (IOException | InterruptedException e) {
}
}
public static void drawToPngFile(Product product, String address, String name) {
drawToFile(product, ToStringMode.REDBERRY, "png", address, name);
}
public static void createTexFile(Product product, String address, String fileName) {
System.out.println();
System.out.println("Executing dot2tex:");
//creating tex file
try {
String code = ContractionsGraphDrawer.draw(product, ToStringMode.LaTeX);
ProcessBuilder pb = new ProcessBuilder(BIN_DIR + "dot2tex", "-tmath", "--autosize", "--nominsize");
pb.redirectErrorStream(true);
pb.redirectOutput(new File(address + fileName + ".tex"));
Process process = pb.start();
PrintStream ps = new PrintStream(process.getOutputStream());
ps.println(code);
ps.close();
process.waitFor();
System.out.println("Done.");
} catch (IOException | InterruptedException e) {
}
System.out.println();
System.out.println("Executing LaTeX:");
//latex
Process p;
try {
p = Runtime.getRuntime().exec(BIN_DIR + "latex -output-directory " + address + " " + address + fileName);
BufferedReader bri = new BufferedReader(new InputStreamReader(p.getInputStream()));
BufferedReader bre = new BufferedReader(new InputStreamReader(p.getErrorStream()));
String line;
while ((line = bri.readLine()) != null)
System.out.println(line);
bri.close();
while ((line = bre.readLine()) != null)
System.out.println(line);
bre.close();
p.waitFor();
System.out.println("Done.");
} catch (IOException | InterruptedException ex) {
System.out.println(ex);
}
System.out.println();
System.out.println("PS:");
//ps
try {
p = Runtime.getRuntime().exec(BIN_DIR + "dvips -o " + address + fileName + ".ps " + address + fileName);
BufferedReader bri = new BufferedReader(new InputStreamReader(p.getInputStream()));
BufferedReader bre = new BufferedReader(new InputStreamReader(p.getErrorStream()));
String line;
while ((line = bri.readLine()) != null)
System.out.println(line);
bri.close();
while ((line = bre.readLine()) != null)
System.out.println(line);
bre.close();
p.waitFor();
System.out.println("Done.");
} catch (IOException | InterruptedException ex) {
System.out.println(ex);
}
System.out.println();
System.out.println("PDF:");
//pdf
try {
p = Runtime.getRuntime().exec(BIN_DIR + "ps2pdf " + address + fileName + ".ps " + address + fileName + ".pdf");
BufferedReader bri = new BufferedReader(new InputStreamReader(p.getInputStream()));
BufferedReader bre = new BufferedReader(new InputStreamReader(p.getErrorStream()));
String line;
while ((line = bri.readLine()) != null)
System.out.println(line);
bri.close();
while ((line = bre.readLine()) != null)
System.out.println(line);
bre.close();
p.waitFor();
System.out.println("Done.");
} catch (IOException | InterruptedException ex) {
System.out.println(ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy