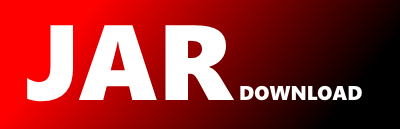
cc.shacocloud.mirage.web.bind.WebDataBinder Maven / Gradle / Ivy
package cc.shacocloud.mirage.web.bind;
import org.springframework.core.CollectionFactory;
import org.jetbrains.annotations.Nullable;
import org.springframework.validation.DataBinder;
import java.lang.reflect.Array;
import java.util.Collection;
import java.util.Map;
/**
* 用于从web请求参数到JavaBean对象的数据绑定的特殊 {@link DataBinder}
*/
public class WebDataBinder extends DataBinder {
/**
* 创建一个新的WebDataBinder实例,使用默认的对象名称。
*
* @param target 要绑定到的目标对象(如果绑定器只是用于转换普通参数值,则为{@code null})
* @see #DEFAULT_OBJECT_NAME
*/
public WebDataBinder(@Nullable Object target) {
super(target);
}
/**
* 创建一个新的WebDataBinder实例。
*
* @param target 要绑定到的目标对象(如果绑定器只是用于转换普通参数值,则为{@code null})
* @param objectName 目标对象的名称
*/
public WebDataBinder(@Nullable Object target, String objectName) {
super(target, objectName);
}
/**
* 确定指定字段的空值
*
* 如果字段类型已知,则默认实现将委托给{@link #getEmptyValue(Class)},否则将返回到{@code null}。
*
* @param field 字段的名称
* @param fieldType 字段的类型
*/
@Nullable
protected Object getEmptyValue(String field, @Nullable Class> fieldType) {
return (fieldType != null ? getEmptyValue(fieldType) : null);
}
/**
* 确定指定字段的空值
*
* 默认实现返回:
*
* - {@code Boolean} 布尔值返回 {@code FALSE}
*
- 用于数组类型的空数组
*
- 集合类型的集合实现
*
- 映射类型的映射实现
*
- 否则,使用{@code null}作为默认值
*
*
* @param fieldType 字段的类型
*/
@Nullable
public Object getEmptyValue(Class> fieldType) {
try {
if (boolean.class == fieldType || Boolean.class == fieldType) {
// 布尔属性的特殊处理。
return Boolean.FALSE;
} else if (fieldType.isArray()) {
// 数组属性的特殊处理。
return Array.newInstance(fieldType.getComponentType(), 0);
} else if (Collection.class.isAssignableFrom(fieldType)) {
return CollectionFactory.createCollection(fieldType, 0);
} else if (Map.class.isAssignableFrom(fieldType)) {
return CollectionFactory.createMap(fieldType, 0);
}
} catch (IllegalArgumentException ex) {
if (logger.isDebugEnabled()) {
logger.debug("未能创建默认值—返回null: " + ex.getMessage());
}
}
// 默认值:空
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy