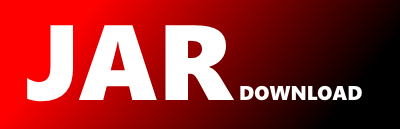
ch.acanda.maven.coan.AggregateMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of code-analysis-maven-plugin Show documentation
Show all versions of code-analysis-maven-plugin Show documentation
The Code Analysis Maven Plugin runs several static analysis tools to check
your code for bugs, design and formatting problems.
package ch.acanda.maven.coan;
import ch.acanda.maven.coan.checkstyle.CheckstyleInspector;
import ch.acanda.maven.coan.pmd.PmdInspector;
import ch.acanda.maven.coan.report.LogReport;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.RejectedExecutionException;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.stream.Stream;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
@Mojo(name = "aggregate", aggregator = true, threadSafe = true)
public class AggregateMojo extends AbstractCoanMojo {
@Parameter(property = "reactorProjects", readonly = true, required = true)
private List reactorProjects;
@Override
protected void analyseCode() throws MojoFailureException {
logReactorProjects();
final List> analysers = reactorProjects.stream()
.flatMap(reactorProject -> Stream.>of(
() -> new PmdInspector(assemblePmdConfig(reactorProject)).inspect(),
() -> new CheckstyleInspector(assembleCheckstyleConfig(reactorProject)).inspect()
))
.collect(toList());
final ExecutorService executorService = Executors.newFixedThreadPool(analysers.size());
try {
final List> runningAnalyses = executorService.invokeAll(analysers, 1, TimeUnit.HOURS);
final List analyses = runningAnalyses.stream()
.map(AggregateMojo::waitUntilFinished)
.collect(toList());
analyses.forEach(analysis -> LogReport.report(analysis, getProject().getBasedir().toPath(), getLog()));
createReports(analyses.toArray(Inspection[]::new));
failOnIssues(analyses);
} catch (final RejectedExecutionException e) {
throw new MojoFailureException(e.getMessage(), e);
} catch (final InterruptedException e) {
Thread.currentThread().interrupt();
throw new MojoFailureException(e.getMessage(), e);
} catch (final AnalysisExecutionException e) {
final Throwable cause = e.getCause();
throw new MojoFailureException(cause.getMessage(), cause); //NOPMD
} finally {
executorService.shutdown();
}
}
private void failOnIssues(final List analyses) throws MojoFailureException {
final boolean foundIssues = analyses.stream().anyMatch(Inspection::foundIssues);
if (isFailOnIssues() && foundIssues) {
final long sum = analyses.stream().mapToInt(Inspection::getNumberOfIssues).sum();
final String issues = sum == 1 ? " issue" : " issues";
throw new MojoFailureException("Code analysis found " + sum + issues + ".");
}
}
private void logReactorProjects() {
if (getLog().isDebugEnabled()) {
final String projects = reactorProjects.stream()
.map(MavenProject::toString)
.collect(joining("\n ", "Projects:\n ", ""));
getLog().debug(projects);
}
}
private static Inspection waitUntilFinished(final Future runningAnalysis) {
try {
return runningAnalysis.get(1, TimeUnit.HOURS);
} catch (final InterruptedException e) {
Thread.currentThread().interrupt();
throw new AnalysisExecutionException(e);
} catch (final ExecutionException | TimeoutException e) {
throw new AnalysisExecutionException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy