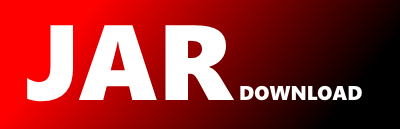
ch.acanda.maven.coan.report.HtmlReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of code-analysis-maven-plugin Show documentation
Show all versions of code-analysis-maven-plugin Show documentation
The Code Analysis Maven Plugin runs several static analysis tools to check
your code for bugs, design and formatting problems.
package ch.acanda.maven.coan.report;
import ch.acanda.maven.coan.Inspection;
import ch.acanda.maven.coan.Issue;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.Writer;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Arrays;
import java.util.List;
import java.util.LongSummaryStatistics;
import java.util.Map;
import static java.util.Comparator.comparing;
import static java.util.stream.Collectors.groupingBy;
import static java.util.stream.Collectors.summarizingLong;
import static org.apache.commons.text.StringEscapeUtils.escapeHtml4;
@SuppressWarnings("java:S1192" /* duplicated strings: creating constants for html tags makes the code less readable. */)
public class HtmlReport {
private final MavenProject project;
private final Path baseDir;
private final List analyses;
public HtmlReport(final MavenProject project, final Path baseDir, final Inspection... analyses) {
this.project = project;
this.baseDir = baseDir;
this.analyses = Arrays.asList(analyses);
}
public void writeTo(final Path file) throws MojoFailureException {
try (Writer out = Files.newBufferedWriter(file)) {
writeTo(out);
} catch (final IOException e) {
throw new MojoFailureException("Failed to write HTML report to file " + file + ".", e);
}
}
private void writeTo(final Writer out) throws IOException {
final PrintWriter html = new PrintWriter(out);
html.println("");
html.println("");
writeHead(html);
writeBody(html);
html.println("");
html.flush();
if (html.checkError()) {
throw new IOException("Failed to create HTML report.");
}
}
private void writeBody(final PrintWriter html) {
html.println("");
html.print("Code Analysis for ");
html.print(escapeHtml4(getProjectName(project)));
final String version = project.getVersion();
if (version != null) {
html.print(" ");
html.print(version);
}
html.println("
");
writeSummary(html);
writeAnalyses(html);
html.println("");
}
private void writeSummary(final PrintWriter html) {
final boolean foundIssues = analyses.stream().anyMatch(Inspection::foundIssues);
html.println("");
if (foundIssues) {
html.println("Summary
");
analyses.stream()
.collect(groupingBy(Inspection::toolName, summarizingLong(Inspection::getNumberOfIssues)))
.entrySet()
.stream()
.map(entry -> escapeHtml4(entry.getKey()) + " found " + numberOfIssues(entry.getValue()) + ".")
.sorted()
.forEachOrdered(toolSummary -> {
html.print("");
html.print(toolSummary);
html.println("
");
});
} else {
html.println("Congratulations!
");
html.println("The code analysers did not find any issues.
");
}
html.println(" ");
}
private void writeAnalyses(final PrintWriter html) {
final Map> analysesByProject = analyses.stream()
.filter(Inspection::foundIssues)
.collect(groupingBy(Inspection::project));
final boolean includeProjectName = analysesByProject.size() > 1;
analysesByProject
.entrySet()
.stream()
.sorted(comparing(e -> getProjectName(e.getKey())))
.forEachOrdered(entry -> writeAnalyses(entry.getKey(), entry.getValue(), includeProjectName, html));
}
private void writeAnalyses(final MavenProject project, final List analyses,
final boolean includeProjectName, final PrintWriter html) {
if (includeProjectName) {
html.println("");
html.println("");
html.print("");
html.print(escapeHtml4(getProjectName(project)));
html.println("
");
}
analyses.forEach(inspection -> writeAnalysis(inspection, html));
if (includeProjectName) {
html.println("");
html.println(" ");
}
}
private void writeAnalysis(final Inspection inspection, final PrintWriter html) {
html.println("");
html.print("");
html.print(escapeHtml4(inspection.toolName()));
html.println(" Report
");
inspection.issues()
.stream()
.collect(groupingBy(Issue::file))
.forEach((file, issues) -> writeIssues(file, issues, html));
html.println(" ");
}
private void writeIssues(final Path file, final List extends Issue> issues, final PrintWriter html) {
html.print("");
html.print(escapeHtml4(baseDir.relativize(file).toString().replace('\\', '/')));
html.println("
");
html.println("");
issues.stream()
.sorted(comparing(Issue::severity)
.thenComparing(Issue::name)
.thenComparing(Issue::line)
.thenComparing(Issue::column))
.forEachOrdered(issue -> writeIssue(issue, html));
html.println("
");
}
private static String getProjectName(final MavenProject project) {
final String name = project.getName();
return name == null ? project.getArtifactId() : name;
}
private static String numberOfIssues(final LongSummaryStatistics summary) {
final long sum = summary.getSum();
final String noun = sum == 1 ? " issue" : " issues";
return sum + noun;
}
private static void writeHead(final PrintWriter html) {
html.println("""
Code Analysis Report
""");
}
private static void writeIssue(final Issue issue, final PrintWriter html) {
html.print("");
html.print(escapeHtml4(issue.name()));
html.print(" ");
html.print(escapeHtml4(issue.description()));
html.print(" (");
html.print(issue.line());
html.print(':');
html.print(issue.column());
html.println(") ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy