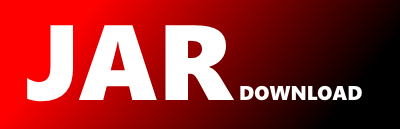
ch.agent.crnickl.mongodb.AccessMethodsForAny Maven / Gradle / Ivy
Show all versions of crnickl-mongodb Show documentation
/*
* Copyright 2012-2013 Hauser Olsson GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package ch.agent.crnickl.mongodb;
import ch.agent.crnickl.T2DBException;
import ch.agent.crnickl.api.Series;
import ch.agent.crnickl.api.UpdatableSeries;
import ch.agent.crnickl.api.ValueType;
import ch.agent.crnickl.impl.ChronicleUpdatePolicy;
import ch.agent.crnickl.impl.ValueAccessMethods;
import ch.agent.t2.time.Range;
import ch.agent.t2.time.TimeIndex;
import ch.agent.t2.timeseries.Observation;
import ch.agent.t2.timeseries.TimeAddressable;
/**
* An implementation of {@link ValueAccessMethods} which
* stores values as strings. It supports
* any type supported by a {@link ValueType}.
*
* Methods are only stubs. Actually using them throws exceptions.
*
* @author Jean-Paul Vetterli
* @param the data type of values
*/
public class AccessMethodsForAny extends MongoDatabaseMethods implements ValueAccessMethods {
private ValueType valueType;
/**
* Construct an access method object.
*/
public AccessMethodsForAny() {
}
/**
* Set the value type.
*
* @param valueType a value type
*/
public void setValueType(ValueType valueType) {
this.valueType = valueType;
}
private RuntimeException ouch() {
return new RuntimeException("ACCESS METHODS NOT IMPLEMENTED: " + valueType.getName());
}
@Override
public Range getRange(Series series) throws T2DBException {
throw ouch();
}
@Override
public long getValues(Series series, Range range, TimeAddressable ts) throws T2DBException {
throw ouch();
}
@Override
public Observation getFirst(Series series, TimeIndex time) throws T2DBException {
throw ouch();
}
@Override
public Observation getLast(Series series, TimeIndex time) throws T2DBException {
throw ouch();
}
@Override
public boolean deleteValue(UpdatableSeries series, TimeIndex t, ChronicleUpdatePolicy policy) throws T2DBException {
throw ouch();
}
@Override
public boolean updateSeries(UpdatableSeries series, Range range, ChronicleUpdatePolicy policy) throws T2DBException {
throw ouch();
}
@Override
public long updateValues(UpdatableSeries series, TimeAddressable values, ChronicleUpdatePolicy policy) throws T2DBException {
throw ouch();
}
}