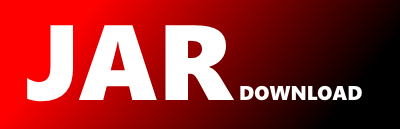
net.datastream.schemas.mp_entities.casemanagement_001.TrackingDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eam-wshub-proxyclient Show documentation
Show all versions of eam-wshub-proxyclient Show documentation
JAX-WS aisws aisws generated from Infor EAM Web Services Toolkit WSDLs.
package net.datastream.schemas.mp_entities.casemanagement_001;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import net.datastream.schemas.mp_fields.CONTACTRECORDID_Type;
import net.datastream.schemas.mp_fields.Employee_Type;
import net.datastream.schemas.mp_fields.PERSONID_Type;
import net.datastream.schemas.mp_fields.STATUS_Type;
import net.datastream.schemas.mp_fields.TYPE_Type;
import net.datastream.schemas.mp_fields.USERID_Type;
import org.openapplications.oagis_segments.DATETIME;
/**
* Classe Java pour anonymous complex type.
*
*
Le fragment de schéma suivant indique le contenu attendu figurant dans cette classe.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://schemas.datastream.net/MP_fields}REQUESTEDBY" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}DATEREQUESTED" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}PERSONRESPONSIBLE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}EMAIL" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}PREPAREDBY" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}PREPAREDBYEMAIL" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}ASSIGNEDTO" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}ASSIGNEDTOEMAIL" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}SCHEDULEDSTARTDATE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}SCHEDULEDENDDATE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}REQUESTEDSTART" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}REQUESTEDEND" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}STARTDATE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}COMPLETEDDATE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CONTACTRECORDID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CONTACTRECORDSTATUS" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}SOURCETYPE" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"requestedby",
"daterequested",
"personresponsible",
"email",
"preparedby",
"preparedbyemail",
"assignedto",
"assignedtoemail",
"scheduledstartdate",
"scheduledenddate",
"requestedstart",
"requestedend",
"startdate",
"completeddate",
"contactrecordid",
"contactrecordstatus",
"sourcetype"
})
@XmlRootElement(name = "TrackingDetails")
public class TrackingDetails {
@XmlElement(name = "REQUESTEDBY", namespace = "http://schemas.datastream.net/MP_fields")
protected PERSONID_Type requestedby;
@XmlElement(name = "DATEREQUESTED", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME daterequested;
@XmlElement(name = "PERSONRESPONSIBLE", namespace = "http://schemas.datastream.net/MP_fields")
protected Employee_Type personresponsible;
@XmlElement(name = "EMAIL", namespace = "http://schemas.datastream.net/MP_fields")
protected String email;
@XmlElement(name = "PREPAREDBY", namespace = "http://schemas.datastream.net/MP_fields")
protected USERID_Type preparedby;
@XmlElement(name = "PREPAREDBYEMAIL", namespace = "http://schemas.datastream.net/MP_fields")
protected String preparedbyemail;
@XmlElement(name = "ASSIGNEDTO", namespace = "http://schemas.datastream.net/MP_fields")
protected PERSONID_Type assignedto;
@XmlElement(name = "ASSIGNEDTOEMAIL", namespace = "http://schemas.datastream.net/MP_fields")
protected String assignedtoemail;
@XmlElement(name = "SCHEDULEDSTARTDATE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME scheduledstartdate;
@XmlElement(name = "SCHEDULEDENDDATE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME scheduledenddate;
@XmlElement(name = "REQUESTEDSTART", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME requestedstart;
@XmlElement(name = "REQUESTEDEND", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME requestedend;
@XmlElement(name = "STARTDATE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME startdate;
@XmlElement(name = "COMPLETEDDATE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME completeddate;
@XmlElement(name = "CONTACTRECORDID", namespace = "http://schemas.datastream.net/MP_fields")
protected CONTACTRECORDID_Type contactrecordid;
@XmlElement(name = "CONTACTRECORDSTATUS", namespace = "http://schemas.datastream.net/MP_fields")
protected STATUS_Type contactrecordstatus;
@XmlElement(name = "SOURCETYPE", namespace = "http://schemas.datastream.net/MP_fields")
protected TYPE_Type sourcetype;
/**
* Obtient la valeur de la propriété requestedby.
*
* @return
* possible object is
* {@link PERSONID_Type }
*
*/
public PERSONID_Type getREQUESTEDBY() {
return requestedby;
}
/**
* Définit la valeur de la propriété requestedby.
*
* @param value
* allowed object is
* {@link PERSONID_Type }
*
*/
public void setREQUESTEDBY(PERSONID_Type value) {
this.requestedby = value;
}
/**
* Obtient la valeur de la propriété daterequested.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getDATEREQUESTED() {
return daterequested;
}
/**
* Définit la valeur de la propriété daterequested.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setDATEREQUESTED(DATETIME value) {
this.daterequested = value;
}
/**
* Obtient la valeur de la propriété personresponsible.
*
* @return
* possible object is
* {@link Employee_Type }
*
*/
public Employee_Type getPERSONRESPONSIBLE() {
return personresponsible;
}
/**
* Définit la valeur de la propriété personresponsible.
*
* @param value
* allowed object is
* {@link Employee_Type }
*
*/
public void setPERSONRESPONSIBLE(Employee_Type value) {
this.personresponsible = value;
}
/**
* Obtient la valeur de la propriété email.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEMAIL() {
return email;
}
/**
* Définit la valeur de la propriété email.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEMAIL(String value) {
this.email = value;
}
/**
* Obtient la valeur de la propriété preparedby.
*
* @return
* possible object is
* {@link USERID_Type }
*
*/
public USERID_Type getPREPAREDBY() {
return preparedby;
}
/**
* Définit la valeur de la propriété preparedby.
*
* @param value
* allowed object is
* {@link USERID_Type }
*
*/
public void setPREPAREDBY(USERID_Type value) {
this.preparedby = value;
}
/**
* Obtient la valeur de la propriété preparedbyemail.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPREPAREDBYEMAIL() {
return preparedbyemail;
}
/**
* Définit la valeur de la propriété preparedbyemail.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPREPAREDBYEMAIL(String value) {
this.preparedbyemail = value;
}
/**
* Obtient la valeur de la propriété assignedto.
*
* @return
* possible object is
* {@link PERSONID_Type }
*
*/
public PERSONID_Type getASSIGNEDTO() {
return assignedto;
}
/**
* Définit la valeur de la propriété assignedto.
*
* @param value
* allowed object is
* {@link PERSONID_Type }
*
*/
public void setASSIGNEDTO(PERSONID_Type value) {
this.assignedto = value;
}
/**
* Obtient la valeur de la propriété assignedtoemail.
*
* @return
* possible object is
* {@link String }
*
*/
public String getASSIGNEDTOEMAIL() {
return assignedtoemail;
}
/**
* Définit la valeur de la propriété assignedtoemail.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setASSIGNEDTOEMAIL(String value) {
this.assignedtoemail = value;
}
/**
* Obtient la valeur de la propriété scheduledstartdate.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getSCHEDULEDSTARTDATE() {
return scheduledstartdate;
}
/**
* Définit la valeur de la propriété scheduledstartdate.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setSCHEDULEDSTARTDATE(DATETIME value) {
this.scheduledstartdate = value;
}
/**
* Obtient la valeur de la propriété scheduledenddate.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getSCHEDULEDENDDATE() {
return scheduledenddate;
}
/**
* Définit la valeur de la propriété scheduledenddate.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setSCHEDULEDENDDATE(DATETIME value) {
this.scheduledenddate = value;
}
/**
* Obtient la valeur de la propriété requestedstart.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getREQUESTEDSTART() {
return requestedstart;
}
/**
* Définit la valeur de la propriété requestedstart.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setREQUESTEDSTART(DATETIME value) {
this.requestedstart = value;
}
/**
* Obtient la valeur de la propriété requestedend.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getREQUESTEDEND() {
return requestedend;
}
/**
* Définit la valeur de la propriété requestedend.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setREQUESTEDEND(DATETIME value) {
this.requestedend = value;
}
/**
* Obtient la valeur de la propriété startdate.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getSTARTDATE() {
return startdate;
}
/**
* Définit la valeur de la propriété startdate.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setSTARTDATE(DATETIME value) {
this.startdate = value;
}
/**
* Obtient la valeur de la propriété completeddate.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getCOMPLETEDDATE() {
return completeddate;
}
/**
* Définit la valeur de la propriété completeddate.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setCOMPLETEDDATE(DATETIME value) {
this.completeddate = value;
}
/**
* Obtient la valeur de la propriété contactrecordid.
*
* @return
* possible object is
* {@link CONTACTRECORDID_Type }
*
*/
public CONTACTRECORDID_Type getCONTACTRECORDID() {
return contactrecordid;
}
/**
* Définit la valeur de la propriété contactrecordid.
*
* @param value
* allowed object is
* {@link CONTACTRECORDID_Type }
*
*/
public void setCONTACTRECORDID(CONTACTRECORDID_Type value) {
this.contactrecordid = value;
}
/**
* Obtient la valeur de la propriété contactrecordstatus.
*
* @return
* possible object is
* {@link STATUS_Type }
*
*/
public STATUS_Type getCONTACTRECORDSTATUS() {
return contactrecordstatus;
}
/**
* Définit la valeur de la propriété contactrecordstatus.
*
* @param value
* allowed object is
* {@link STATUS_Type }
*
*/
public void setCONTACTRECORDSTATUS(STATUS_Type value) {
this.contactrecordstatus = value;
}
/**
* Obtient la valeur de la propriété sourcetype.
*
* @return
* possible object is
* {@link TYPE_Type }
*
*/
public TYPE_Type getSOURCETYPE() {
return sourcetype;
}
/**
* Définit la valeur de la propriété sourcetype.
*
* @param value
* allowed object is
* {@link TYPE_Type }
*
*/
public void setSOURCETYPE(TYPE_Type value) {
this.sourcetype = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy