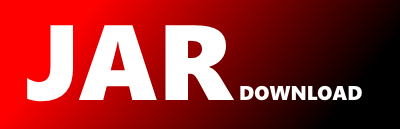
net.datastream.schemas.mp_functions.gridresult.METADATA Maven / Gradle / Ivy
package net.datastream.schemas.mp_functions.gridresult;
import java.math.BigInteger;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Classe Java pour anonymous complex type.
*
*
Le fragment de schéma suivant indique le contenu attendu figurant dans cette classe.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="REQUEST_TYPE_META" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* </restriction>
* </simpleType>
* </element>
* <element name="MULTIORGCOLUMN" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* </restriction>
* </simpleType>
* </element>
* <element name="EDITABLE" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="+"/>
* <enumeration value="-"/>
* </restriction>
* </simpleType>
* </element>
* <element name="MORERECORDPRESENT" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="+"/>
* <enumeration value="-"/>
* </restriction>
* </simpleType>
* </element>
* <element name="GRIDNAME" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* </restriction>
* </simpleType>
* </element>
* <element name="GRIDLABEL" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* </restriction>
* </simpleType>
* </element>
* <element name="RECORDS" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}integer">
* </restriction>
* </simpleType>
* </element>
* <element name="CURRENTCURSORPOSITION" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}integer">
* </restriction>
* </simpleType>
* </element>
* <element name="CLIENTROWS" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}integer">
* </restriction>
* </simpleType>
* </element>
* <element name="GRIDID" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}integer">
* </restriction>
* </simpleType>
* </element>
* <element name="DATASPYID" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}integer">
* </restriction>
* </simpleType>
* </element>
* <element name="SERVERTIMESTAMP" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}integer">
* </restriction>
* </simpleType>
* </element>
* <element name="TRANSTYPE" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="TARGETPROMPTSEQ" type="{http://www.w3.org/2001/XMLSchema}integer" minOccurs="0"/>
* <element name="SRCPROMPTSEQ" type="{http://www.w3.org/2001/XMLSchema}integer" minOccurs="0"/>
* <element name="GRIDTYPE" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"request_TYPE_META",
"multiorgcolumn",
"editable",
"morerecordpresent",
"gridname",
"gridlabel",
"records",
"currentcursorposition",
"clientrows",
"gridid",
"dataspyid",
"servertimestamp",
"transtype",
"targetpromptseq",
"srcpromptseq",
"gridtype"
})
@XmlRootElement(name = "METADATA")
public class METADATA {
@XmlElement(name = "REQUEST_TYPE_META")
protected String request_TYPE_META;
@XmlElement(name = "MULTIORGCOLUMN")
protected String multiorgcolumn;
@XmlElement(name = "EDITABLE")
protected String editable;
@XmlElement(name = "MORERECORDPRESENT")
protected String morerecordpresent;
@XmlElement(name = "GRIDNAME")
protected String gridname;
@XmlElement(name = "GRIDLABEL")
protected String gridlabel;
@XmlElement(name = "RECORDS")
protected BigInteger records;
@XmlElement(name = "CURRENTCURSORPOSITION")
protected BigInteger currentcursorposition;
@XmlElement(name = "CLIENTROWS")
protected BigInteger clientrows;
@XmlElement(name = "GRIDID")
protected BigInteger gridid;
@XmlElement(name = "DATASPYID")
protected BigInteger dataspyid;
@XmlElement(name = "SERVERTIMESTAMP")
protected BigInteger servertimestamp;
@XmlElement(name = "TRANSTYPE")
protected String transtype;
@XmlElement(name = "TARGETPROMPTSEQ")
protected BigInteger targetpromptseq;
@XmlElement(name = "SRCPROMPTSEQ")
protected BigInteger srcpromptseq;
@XmlElement(name = "GRIDTYPE")
protected String gridtype;
/**
* Obtient la valeur de la propriété request_TYPE_META.
*
* @return
* possible object is
* {@link String }
*
*/
public String getREQUEST_TYPE_META() {
return request_TYPE_META;
}
/**
* Définit la valeur de la propriété request_TYPE_META.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setREQUEST_TYPE_META(String value) {
this.request_TYPE_META = value;
}
/**
* Obtient la valeur de la propriété multiorgcolumn.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMULTIORGCOLUMN() {
return multiorgcolumn;
}
/**
* Définit la valeur de la propriété multiorgcolumn.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMULTIORGCOLUMN(String value) {
this.multiorgcolumn = value;
}
/**
* Obtient la valeur de la propriété editable.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEDITABLE() {
return editable;
}
/**
* Définit la valeur de la propriété editable.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEDITABLE(String value) {
this.editable = value;
}
/**
* Obtient la valeur de la propriété morerecordpresent.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMORERECORDPRESENT() {
return morerecordpresent;
}
/**
* Définit la valeur de la propriété morerecordpresent.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMORERECORDPRESENT(String value) {
this.morerecordpresent = value;
}
/**
* Obtient la valeur de la propriété gridname.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGRIDNAME() {
return gridname;
}
/**
* Définit la valeur de la propriété gridname.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGRIDNAME(String value) {
this.gridname = value;
}
/**
* Obtient la valeur de la propriété gridlabel.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGRIDLABEL() {
return gridlabel;
}
/**
* Définit la valeur de la propriété gridlabel.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGRIDLABEL(String value) {
this.gridlabel = value;
}
/**
* Obtient la valeur de la propriété records.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getRECORDS() {
return records;
}
/**
* Définit la valeur de la propriété records.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setRECORDS(BigInteger value) {
this.records = value;
}
/**
* Obtient la valeur de la propriété currentcursorposition.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getCURRENTCURSORPOSITION() {
return currentcursorposition;
}
/**
* Définit la valeur de la propriété currentcursorposition.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setCURRENTCURSORPOSITION(BigInteger value) {
this.currentcursorposition = value;
}
/**
* Obtient la valeur de la propriété clientrows.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getCLIENTROWS() {
return clientrows;
}
/**
* Définit la valeur de la propriété clientrows.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setCLIENTROWS(BigInteger value) {
this.clientrows = value;
}
/**
* Obtient la valeur de la propriété gridid.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getGRIDID() {
return gridid;
}
/**
* Définit la valeur de la propriété gridid.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setGRIDID(BigInteger value) {
this.gridid = value;
}
/**
* Obtient la valeur de la propriété dataspyid.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getDATASPYID() {
return dataspyid;
}
/**
* Définit la valeur de la propriété dataspyid.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setDATASPYID(BigInteger value) {
this.dataspyid = value;
}
/**
* Obtient la valeur de la propriété servertimestamp.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getSERVERTIMESTAMP() {
return servertimestamp;
}
/**
* Définit la valeur de la propriété servertimestamp.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setSERVERTIMESTAMP(BigInteger value) {
this.servertimestamp = value;
}
/**
* Obtient la valeur de la propriété transtype.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTRANSTYPE() {
return transtype;
}
/**
* Définit la valeur de la propriété transtype.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTRANSTYPE(String value) {
this.transtype = value;
}
/**
* Obtient la valeur de la propriété targetpromptseq.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTARGETPROMPTSEQ() {
return targetpromptseq;
}
/**
* Définit la valeur de la propriété targetpromptseq.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTARGETPROMPTSEQ(BigInteger value) {
this.targetpromptseq = value;
}
/**
* Obtient la valeur de la propriété srcpromptseq.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getSRCPROMPTSEQ() {
return srcpromptseq;
}
/**
* Définit la valeur de la propriété srcpromptseq.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setSRCPROMPTSEQ(BigInteger value) {
this.srcpromptseq = value;
}
/**
* Obtient la valeur de la propriété gridtype.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGRIDTYPE() {
return gridtype;
}
/**
* Définit la valeur de la propriété gridtype.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGRIDTYPE(String value) {
this.gridtype = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy