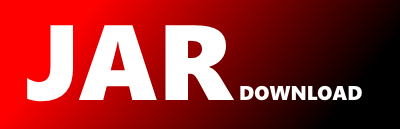
org.openapplications.oagis_segments.OPERAMT Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eam-wshub-proxyclient Show documentation
Show all versions of eam-wshub-proxyclient Show documentation
JAX-WS aisws aisws generated from Infor EAM Web Services Toolkit WSDLs.
package org.openapplications.oagis_segments;
import java.math.BigDecimal;
import java.math.BigInteger;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Classe Java pour OPERAMT complex type.
*
*
Le fragment de schéma suivant indique le contenu attendu figurant dans cette classe.
*
*
* <complexType name="OPERAMT">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.openapplications.org/oagis_fields}VALUE"/>
* <element ref="{http://www.openapplications.org/oagis_fields}NUMOFDEC"/>
* <element ref="{http://www.openapplications.org/oagis_fields}SIGN"/>
* <element ref="{http://www.openapplications.org/oagis_fields}CURRENCY"/>
* <element ref="{http://www.openapplications.org/oagis_fields}UOMVALUE"/>
* <element ref="{http://www.openapplications.org/oagis_fields}UOMNUMDEC"/>
* <element ref="{http://www.openapplications.org/oagis_fields}UOM"/>
* </sequence>
* <attribute name="qualifier" use="required">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}NMTOKEN">
* <enumeration value="COST"/>
* <enumeration value="EXTENDED"/>
* <enumeration value="FREIGHT"/>
* <enumeration value="UNIT"/>
* <enumeration value="OTHER"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="type" use="required">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}NMTOKEN">
* <enumeration value="T"/>
* <enumeration value="F"/>
* <enumeration value="OTHER"/>
* </restriction>
* </simpleType>
* </attribute>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "OPERAMT", propOrder = {
"value",
"numofdec",
"sign",
"currency",
"uomvalue",
"uomnumdec",
"uom"
})
public class OPERAMT {
@XmlElement(name = "VALUE", namespace = "http://www.openapplications.org/oagis_fields", required = true)
protected BigDecimal value;
@XmlElement(name = "NUMOFDEC", namespace = "http://www.openapplications.org/oagis_fields", required = true)
protected BigInteger numofdec;
@XmlElement(name = "SIGN", namespace = "http://www.openapplications.org/oagis_fields", required = true)
protected String sign;
@XmlElement(name = "CURRENCY", namespace = "http://www.openapplications.org/oagis_fields", required = true)
protected String currency;
@XmlElement(name = "UOMVALUE", namespace = "http://www.openapplications.org/oagis_fields", required = true)
protected String uomvalue;
@XmlElement(name = "UOMNUMDEC", namespace = "http://www.openapplications.org/oagis_fields", required = true)
protected BigInteger uomnumdec;
@XmlElement(name = "UOM", namespace = "http://www.openapplications.org/oagis_fields", required = true)
protected String uom;
@XmlAttribute(name = "qualifier", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String qualifier;
@XmlAttribute(name = "type", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String type;
/**
* Obtient la valeur de la propriété value.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getVALUE() {
return value;
}
/**
* Définit la valeur de la propriété value.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setVALUE(BigDecimal value) {
this.value = value;
}
/**
* Obtient la valeur de la propriété numofdec.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getNUMOFDEC() {
return numofdec;
}
/**
* Définit la valeur de la propriété numofdec.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setNUMOFDEC(BigInteger value) {
this.numofdec = value;
}
/**
* Obtient la valeur de la propriété sign.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSIGN() {
return sign;
}
/**
* Définit la valeur de la propriété sign.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSIGN(String value) {
this.sign = value;
}
/**
* Obtient la valeur de la propriété currency.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCURRENCY() {
return currency;
}
/**
* Définit la valeur de la propriété currency.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCURRENCY(String value) {
this.currency = value;
}
/**
* Obtient la valeur de la propriété uomvalue.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUOMVALUE() {
return uomvalue;
}
/**
* Définit la valeur de la propriété uomvalue.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUOMVALUE(String value) {
this.uomvalue = value;
}
/**
* Obtient la valeur de la propriété uomnumdec.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getUOMNUMDEC() {
return uomnumdec;
}
/**
* Définit la valeur de la propriété uomnumdec.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setUOMNUMDEC(BigInteger value) {
this.uomnumdec = value;
}
/**
* Obtient la valeur de la propriété uom.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUOM() {
return uom;
}
/**
* Définit la valeur de la propriété uom.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUOM(String value) {
this.uom = value;
}
/**
* Obtient la valeur de la propriété qualifier.
*
* @return
* possible object is
* {@link String }
*
*/
public String getQualifier() {
return qualifier;
}
/**
* Définit la valeur de la propriété qualifier.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setQualifier(String value) {
this.qualifier = value;
}
/**
* Obtient la valeur de la propriété type.
*
* @return
* possible object is
* {@link String }
*
*/
public String getType() {
return type;
}
/**
* Définit la valeur de la propriété type.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setType(String value) {
this.type = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy