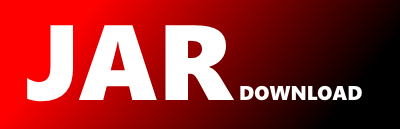
net.datastream.schemas.mp_entities.addresses_001.Addresses Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eam-wshub-proxyclient Show documentation
Show all versions of eam-wshub-proxyclient Show documentation
JAX-WS aisws aisws generated from Infor EAM Web Services Toolkit WSDLs.
package net.datastream.schemas.mp_entities.addresses_001;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import net.datastream.schemas.mp_fields.ADDRESSID;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://schemas.datastream.net/MP_fields}ADDRESSID"/>
* <element name="TEXT" type="{http://schemas.datastream.net/MP_fields}CODE1000_Type" minOccurs="0"/>
* <element name="ADDRESS1" type="{http://schemas.datastream.net/MP_fields}CODE64_Type" minOccurs="0"/>
* <element name="ADDRESS2" type="{http://schemas.datastream.net/MP_fields}CODE64_Type" minOccurs="0"/>
* <element name="ADDRESS3" type="{http://schemas.datastream.net/MP_fields}CODE64_Type" minOccurs="0"/>
* <element name="CITY" type="{http://schemas.datastream.net/MP_fields}CODE20_Type" minOccurs="0"/>
* <element name="STATE" type="{http://schemas.datastream.net/MP_fields}CODE30_Type" minOccurs="0"/>
* <element name="ZIP" type="{http://schemas.datastream.net/MP_fields}CODE10_Type" minOccurs="0"/>
* <element name="COUNTRY" type="{http://schemas.datastream.net/MP_fields}CODE30_Type" minOccurs="0"/>
* <element name="PHONE" type="{http://schemas.datastream.net/MP_fields}CODE50_Type" minOccurs="0"/>
* <element name="PHONEEXT" type="{http://schemas.datastream.net/MP_fields}CODE50_Type" minOccurs="0"/>
* <element name="FAX" type="{http://schemas.datastream.net/MP_fields}CODE50_Type" minOccurs="0"/>
* <element name="EMAIL" type="{http://schemas.datastream.net/MP_fields}CODE180_Type" minOccurs="0"/>
* </sequence>
* <attribute name="updatecount" type="{http://www.w3.org/2001/XMLSchema}long" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"addressid",
"text",
"address1",
"address2",
"address3",
"city",
"state",
"zip",
"country",
"phone",
"phoneext",
"fax",
"email"
})
@XmlRootElement(name = "Addresses")
public class Addresses {
@XmlElement(name = "ADDRESSID", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected ADDRESSID addressid;
@XmlElement(name = "TEXT")
protected String text;
@XmlElement(name = "ADDRESS1")
protected String address1;
@XmlElement(name = "ADDRESS2")
protected String address2;
@XmlElement(name = "ADDRESS3")
protected String address3;
@XmlElement(name = "CITY")
protected String city;
@XmlElement(name = "STATE")
protected String state;
@XmlElement(name = "ZIP")
protected String zip;
@XmlElement(name = "COUNTRY")
protected String country;
@XmlElement(name = "PHONE")
protected String phone;
@XmlElement(name = "PHONEEXT")
protected String phoneext;
@XmlElement(name = "FAX")
protected String fax;
@XmlElement(name = "EMAIL")
protected String email;
@XmlAttribute(name = "updatecount")
protected Long updatecount;
/**
* Gets the value of the addressid property.
*
* @return
* possible object is
* {@link ADDRESSID }
*
*/
public ADDRESSID getADDRESSID() {
return addressid;
}
/**
* Sets the value of the addressid property.
*
* @param value
* allowed object is
* {@link ADDRESSID }
*
*/
public void setADDRESSID(ADDRESSID value) {
this.addressid = value;
}
/**
* Gets the value of the text property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTEXT() {
return text;
}
/**
* Sets the value of the text property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTEXT(String value) {
this.text = value;
}
/**
* Gets the value of the address1 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getADDRESS1() {
return address1;
}
/**
* Sets the value of the address1 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setADDRESS1(String value) {
this.address1 = value;
}
/**
* Gets the value of the address2 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getADDRESS2() {
return address2;
}
/**
* Sets the value of the address2 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setADDRESS2(String value) {
this.address2 = value;
}
/**
* Gets the value of the address3 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getADDRESS3() {
return address3;
}
/**
* Sets the value of the address3 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setADDRESS3(String value) {
this.address3 = value;
}
/**
* Gets the value of the city property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCITY() {
return city;
}
/**
* Sets the value of the city property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCITY(String value) {
this.city = value;
}
/**
* Gets the value of the state property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSTATE() {
return state;
}
/**
* Sets the value of the state property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSTATE(String value) {
this.state = value;
}
/**
* Gets the value of the zip property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getZIP() {
return zip;
}
/**
* Sets the value of the zip property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setZIP(String value) {
this.zip = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCOUNTRY() {
return country;
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCOUNTRY(String value) {
this.country = value;
}
/**
* Gets the value of the phone property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPHONE() {
return phone;
}
/**
* Sets the value of the phone property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPHONE(String value) {
this.phone = value;
}
/**
* Gets the value of the phoneext property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPHONEEXT() {
return phoneext;
}
/**
* Sets the value of the phoneext property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPHONEEXT(String value) {
this.phoneext = value;
}
/**
* Gets the value of the fax property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFAX() {
return fax;
}
/**
* Sets the value of the fax property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFAX(String value) {
this.fax = value;
}
/**
* Gets the value of the email property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEMAIL() {
return email;
}
/**
* Sets the value of the email property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEMAIL(String value) {
this.email = value;
}
/**
* Gets the value of the updatecount property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getUpdatecount() {
return updatecount;
}
/**
* Sets the value of the updatecount property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setUpdatecount(Long value) {
this.updatecount = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy