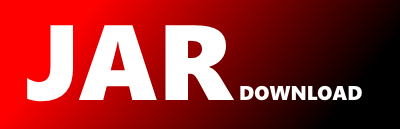
net.datastream.schemas.mp_entities.depreciationdefault_001.DepreciationDefault Maven / Gradle / Ivy
package net.datastream.schemas.mp_entities.depreciationdefault_001;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import net.datastream.schemas.mp_fields.DEPRECIATIONCATEGORYID_Type;
import net.datastream.schemas.mp_fields.STATUS_Type;
import org.openapplications.oagis_segments.AMOUNT;
import org.openapplications.oagis_segments.DATETIME;
import org.openapplications.oagis_segments.QUANTITY;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://schemas.datastream.net/MP_fields}DEPRECIATIONMETHOD"/>
* <element ref="{http://schemas.datastream.net/MP_fields}RSTATUS" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}FROMDATE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}RESIDUALVALUE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}DEPRECIATIONCATEGORYID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}ORIGINALVALUE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}ESTIMATEDOUTPUT" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_entities/DepreciationDefault_001}RemainingUsefulLife" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}RELATIVETOCOMMISSION" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}ORIGINALCOMPONENTVALUE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CHANGEVALUE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}TRANSFERDATE" minOccurs="0"/>
* </sequence>
* <attribute name="has_department_security" type="{http://schemas.datastream.net/MP_fields}FLAG_Type" />
* <attribute name="has_suspend_depreciation" type="{http://schemas.datastream.net/MP_fields}FLAG_Type" />
* <attribute name="has_unitsofoutput_depreciation" type="{http://schemas.datastream.net/MP_fields}FLAG_Type" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"depreciationmethod",
"rstatus",
"fromdate",
"residualvalue",
"depreciationcategoryid",
"originalvalue",
"estimatedoutput",
"remainingUsefulLife",
"relativetocommission",
"originalcomponentvalue",
"changevalue",
"transferdate"
})
@XmlRootElement(name = "DepreciationDefault")
public class DepreciationDefault {
@XmlElement(name = "DEPRECIATIONMETHOD", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected String depreciationmethod;
@XmlElement(name = "RSTATUS", namespace = "http://schemas.datastream.net/MP_fields")
protected STATUS_Type rstatus;
@XmlElement(name = "FROMDATE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME fromdate;
@XmlElement(name = "RESIDUALVALUE", namespace = "http://schemas.datastream.net/MP_fields")
protected AMOUNT residualvalue;
@XmlElement(name = "DEPRECIATIONCATEGORYID", namespace = "http://schemas.datastream.net/MP_fields")
protected DEPRECIATIONCATEGORYID_Type depreciationcategoryid;
@XmlElement(name = "ORIGINALVALUE", namespace = "http://schemas.datastream.net/MP_fields")
protected AMOUNT originalvalue;
@XmlElement(name = "ESTIMATEDOUTPUT", namespace = "http://schemas.datastream.net/MP_fields")
protected QUANTITY estimatedoutput;
@XmlElement(name = "RemainingUsefulLife")
protected RemainingUsefulLife remainingUsefulLife;
@XmlElement(name = "RELATIVETOCOMMISSION", namespace = "http://schemas.datastream.net/MP_fields", defaultValue = "false")
protected String relativetocommission;
@XmlElement(name = "ORIGINALCOMPONENTVALUE", namespace = "http://schemas.datastream.net/MP_fields")
protected AMOUNT originalcomponentvalue;
@XmlElement(name = "CHANGEVALUE", namespace = "http://schemas.datastream.net/MP_fields")
protected AMOUNT changevalue;
@XmlElement(name = "TRANSFERDATE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME transferdate;
@XmlAttribute(name = "has_department_security")
protected String has_Department_Security;
@XmlAttribute(name = "has_suspend_depreciation")
protected String has_Suspend_Depreciation;
@XmlAttribute(name = "has_unitsofoutput_depreciation")
protected String has_Unitsofoutput_Depreciation;
/**
* Gets the value of the depreciationmethod property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDEPRECIATIONMETHOD() {
return depreciationmethod;
}
/**
* Sets the value of the depreciationmethod property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDEPRECIATIONMETHOD(String value) {
this.depreciationmethod = value;
}
/**
* Gets the value of the rstatus property.
*
* @return
* possible object is
* {@link STATUS_Type }
*
*/
public STATUS_Type getRSTATUS() {
return rstatus;
}
/**
* Sets the value of the rstatus property.
*
* @param value
* allowed object is
* {@link STATUS_Type }
*
*/
public void setRSTATUS(STATUS_Type value) {
this.rstatus = value;
}
/**
* Gets the value of the fromdate property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getFROMDATE() {
return fromdate;
}
/**
* Sets the value of the fromdate property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setFROMDATE(DATETIME value) {
this.fromdate = value;
}
/**
* Gets the value of the residualvalue property.
*
* @return
* possible object is
* {@link AMOUNT }
*
*/
public AMOUNT getRESIDUALVALUE() {
return residualvalue;
}
/**
* Sets the value of the residualvalue property.
*
* @param value
* allowed object is
* {@link AMOUNT }
*
*/
public void setRESIDUALVALUE(AMOUNT value) {
this.residualvalue = value;
}
/**
* Gets the value of the depreciationcategoryid property.
*
* @return
* possible object is
* {@link DEPRECIATIONCATEGORYID_Type }
*
*/
public DEPRECIATIONCATEGORYID_Type getDEPRECIATIONCATEGORYID() {
return depreciationcategoryid;
}
/**
* Sets the value of the depreciationcategoryid property.
*
* @param value
* allowed object is
* {@link DEPRECIATIONCATEGORYID_Type }
*
*/
public void setDEPRECIATIONCATEGORYID(DEPRECIATIONCATEGORYID_Type value) {
this.depreciationcategoryid = value;
}
/**
* Gets the value of the originalvalue property.
*
* @return
* possible object is
* {@link AMOUNT }
*
*/
public AMOUNT getORIGINALVALUE() {
return originalvalue;
}
/**
* Sets the value of the originalvalue property.
*
* @param value
* allowed object is
* {@link AMOUNT }
*
*/
public void setORIGINALVALUE(AMOUNT value) {
this.originalvalue = value;
}
/**
* Gets the value of the estimatedoutput property.
*
* @return
* possible object is
* {@link QUANTITY }
*
*/
public QUANTITY getESTIMATEDOUTPUT() {
return estimatedoutput;
}
/**
* Sets the value of the estimatedoutput property.
*
* @param value
* allowed object is
* {@link QUANTITY }
*
*/
public void setESTIMATEDOUTPUT(QUANTITY value) {
this.estimatedoutput = value;
}
/**
* Gets the value of the remainingUsefulLife property.
*
* @return
* possible object is
* {@link RemainingUsefulLife }
*
*/
public RemainingUsefulLife getRemainingUsefulLife() {
return remainingUsefulLife;
}
/**
* Sets the value of the remainingUsefulLife property.
*
* @param value
* allowed object is
* {@link RemainingUsefulLife }
*
*/
public void setRemainingUsefulLife(RemainingUsefulLife value) {
this.remainingUsefulLife = value;
}
/**
* Gets the value of the relativetocommission property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRELATIVETOCOMMISSION() {
return relativetocommission;
}
/**
* Sets the value of the relativetocommission property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRELATIVETOCOMMISSION(String value) {
this.relativetocommission = value;
}
/**
* Gets the value of the originalcomponentvalue property.
*
* @return
* possible object is
* {@link AMOUNT }
*
*/
public AMOUNT getORIGINALCOMPONENTVALUE() {
return originalcomponentvalue;
}
/**
* Sets the value of the originalcomponentvalue property.
*
* @param value
* allowed object is
* {@link AMOUNT }
*
*/
public void setORIGINALCOMPONENTVALUE(AMOUNT value) {
this.originalcomponentvalue = value;
}
/**
* Gets the value of the changevalue property.
*
* @return
* possible object is
* {@link AMOUNT }
*
*/
public AMOUNT getCHANGEVALUE() {
return changevalue;
}
/**
* Sets the value of the changevalue property.
*
* @param value
* allowed object is
* {@link AMOUNT }
*
*/
public void setCHANGEVALUE(AMOUNT value) {
this.changevalue = value;
}
/**
* Gets the value of the transferdate property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getTRANSFERDATE() {
return transferdate;
}
/**
* Sets the value of the transferdate property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setTRANSFERDATE(DATETIME value) {
this.transferdate = value;
}
/**
* Gets the value of the has_Department_Security property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHas_Department_Security() {
return has_Department_Security;
}
/**
* Sets the value of the has_Department_Security property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHas_Department_Security(String value) {
this.has_Department_Security = value;
}
/**
* Gets the value of the has_Suspend_Depreciation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHas_Suspend_Depreciation() {
return has_Suspend_Depreciation;
}
/**
* Sets the value of the has_Suspend_Depreciation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHas_Suspend_Depreciation(String value) {
this.has_Suspend_Depreciation = value;
}
/**
* Gets the value of the has_Unitsofoutput_Depreciation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHas_Unitsofoutput_Depreciation() {
return has_Unitsofoutput_Depreciation;
}
/**
* Sets the value of the has_Unitsofoutput_Depreciation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHas_Unitsofoutput_Depreciation(String value) {
this.has_Unitsofoutput_Depreciation = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy