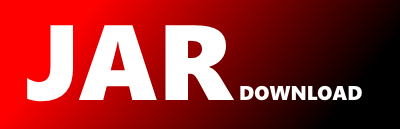
net.datastream.schemas.mp_entities.nonconformityobservation_001.OBTrackingDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eam-wshub-proxyclient Show documentation
Show all versions of eam-wshub-proxyclient Show documentation
JAX-WS aisws aisws generated from Infor EAM Web Services Toolkit WSDLs.
package net.datastream.schemas.mp_entities.nonconformityobservation_001;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import net.datastream.schemas.mp_fields.USERDEFINEDCODEID_Type;
import net.datastream.schemas.mp_fields.USERID_Type;
import net.datastream.schemas.mp_fields.WOID_Type;
import org.openapplications.oagis_segments.DATETIME;
import org.openapplications.oagis_segments.QUANTITY;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://schemas.datastream.net/MP_fields}CONDITIONSCORE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CONDITIONINDEX" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}NEXTINSPECTIONDATE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}NEXTINSPECTIONDATEOVERRIDE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}WORKORDERID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}NONCONFORMITYCODEBEFOREMERGE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CREATEDDATE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CREATEDBY" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}UPDATEDBY" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}DATEUPDATED" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"conditionscore",
"conditionindex",
"nextinspectiondate",
"nextinspectiondateoverride",
"workorderid",
"nonconformitycodebeforemerge",
"createddate",
"createdby",
"updatedby",
"dateupdated"
})
@XmlRootElement(name = "OBTrackingDetails")
public class OBTrackingDetails {
@XmlElement(name = "CONDITIONSCORE", namespace = "http://schemas.datastream.net/MP_fields")
protected QUANTITY conditionscore;
@XmlElement(name = "CONDITIONINDEX", namespace = "http://schemas.datastream.net/MP_fields")
protected USERDEFINEDCODEID_Type conditionindex;
@XmlElement(name = "NEXTINSPECTIONDATE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME nextinspectiondate;
@XmlElement(name = "NEXTINSPECTIONDATEOVERRIDE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME nextinspectiondateoverride;
@XmlElement(name = "WORKORDERID", namespace = "http://schemas.datastream.net/MP_fields")
protected WOID_Type workorderid;
@XmlElement(name = "NONCONFORMITYCODEBEFOREMERGE", namespace = "http://schemas.datastream.net/MP_fields")
protected String nonconformitycodebeforemerge;
@XmlElement(name = "CREATEDDATE", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME createddate;
@XmlElement(name = "CREATEDBY", namespace = "http://schemas.datastream.net/MP_fields")
protected USERID_Type createdby;
@XmlElement(name = "UPDATEDBY", namespace = "http://schemas.datastream.net/MP_fields")
protected USERID_Type updatedby;
@XmlElement(name = "DATEUPDATED", namespace = "http://schemas.datastream.net/MP_fields")
protected DATETIME dateupdated;
/**
* Gets the value of the conditionscore property.
*
* @return
* possible object is
* {@link QUANTITY }
*
*/
public QUANTITY getCONDITIONSCORE() {
return conditionscore;
}
/**
* Sets the value of the conditionscore property.
*
* @param value
* allowed object is
* {@link QUANTITY }
*
*/
public void setCONDITIONSCORE(QUANTITY value) {
this.conditionscore = value;
}
/**
* Gets the value of the conditionindex property.
*
* @return
* possible object is
* {@link USERDEFINEDCODEID_Type }
*
*/
public USERDEFINEDCODEID_Type getCONDITIONINDEX() {
return conditionindex;
}
/**
* Sets the value of the conditionindex property.
*
* @param value
* allowed object is
* {@link USERDEFINEDCODEID_Type }
*
*/
public void setCONDITIONINDEX(USERDEFINEDCODEID_Type value) {
this.conditionindex = value;
}
/**
* Gets the value of the nextinspectiondate property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getNEXTINSPECTIONDATE() {
return nextinspectiondate;
}
/**
* Sets the value of the nextinspectiondate property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setNEXTINSPECTIONDATE(DATETIME value) {
this.nextinspectiondate = value;
}
/**
* Gets the value of the nextinspectiondateoverride property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getNEXTINSPECTIONDATEOVERRIDE() {
return nextinspectiondateoverride;
}
/**
* Sets the value of the nextinspectiondateoverride property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setNEXTINSPECTIONDATEOVERRIDE(DATETIME value) {
this.nextinspectiondateoverride = value;
}
/**
* Gets the value of the workorderid property.
*
* @return
* possible object is
* {@link WOID_Type }
*
*/
public WOID_Type getWORKORDERID() {
return workorderid;
}
/**
* Sets the value of the workorderid property.
*
* @param value
* allowed object is
* {@link WOID_Type }
*
*/
public void setWORKORDERID(WOID_Type value) {
this.workorderid = value;
}
/**
* Gets the value of the nonconformitycodebeforemerge property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNONCONFORMITYCODEBEFOREMERGE() {
return nonconformitycodebeforemerge;
}
/**
* Sets the value of the nonconformitycodebeforemerge property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNONCONFORMITYCODEBEFOREMERGE(String value) {
this.nonconformitycodebeforemerge = value;
}
/**
* Gets the value of the createddate property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getCREATEDDATE() {
return createddate;
}
/**
* Sets the value of the createddate property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setCREATEDDATE(DATETIME value) {
this.createddate = value;
}
/**
* Gets the value of the createdby property.
*
* @return
* possible object is
* {@link USERID_Type }
*
*/
public USERID_Type getCREATEDBY() {
return createdby;
}
/**
* Sets the value of the createdby property.
*
* @param value
* allowed object is
* {@link USERID_Type }
*
*/
public void setCREATEDBY(USERID_Type value) {
this.createdby = value;
}
/**
* Gets the value of the updatedby property.
*
* @return
* possible object is
* {@link USERID_Type }
*
*/
public USERID_Type getUPDATEDBY() {
return updatedby;
}
/**
* Sets the value of the updatedby property.
*
* @param value
* allowed object is
* {@link USERID_Type }
*
*/
public void setUPDATEDBY(USERID_Type value) {
this.updatedby = value;
}
/**
* Gets the value of the dateupdated property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getDATEUPDATED() {
return dateupdated;
}
/**
* Sets the value of the dateupdated property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setDATEUPDATED(DATETIME value) {
this.dateupdated = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy