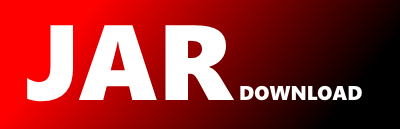
net.datastream.schemas.mp_entities.profile_001.Profile Maven / Gradle / Ivy
package net.datastream.schemas.mp_entities.profile_001;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import net.datastream.schemas.mp_fields.CATEGORYID;
import net.datastream.schemas.mp_fields.CLASSID_Type;
import net.datastream.schemas.mp_fields.COSTCODEID_Type;
import net.datastream.schemas.mp_fields.DEPARTMENTID_Type;
import net.datastream.schemas.mp_fields.OBJECT_Type;
import net.datastream.schemas.mp_fields.PARTID_Type;
import net.datastream.schemas.mp_fields.STATUS_Type;
import net.datastream.schemas.mp_fields.STOREID_Type;
import net.datastream.schemas.mp_fields.TYPE_Type;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://schemas.datastream.net/MP_fields}PROFILEID"/>
* <element ref="{http://schemas.datastream.net/MP_fields}TYPE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CLASSID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}STATUS" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}DEPARTMENTID"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CATEGORYID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}COSTCODEID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}STOREID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}PARTID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}METERUNIT" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_entities/Profile_001}ManufacturerInfo" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CNNUMBER" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_entities/Profile_001}Variables" minOccurs="0"/>
* </sequence>
* <attribute name="recordid" type="{http://www.w3.org/2001/XMLSchema}long" />
* <attribute name="user_entity" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="system_entity" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"profileid",
"type",
"classid",
"status",
"departmentid",
"categoryid",
"costcodeid",
"storeid",
"partid",
"meterunit",
"manufacturerInfo",
"cnnumber",
"variables"
})
@XmlRootElement(name = "Profile")
public class Profile {
@XmlElement(name = "PROFILEID", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected OBJECT_Type profileid;
@XmlElement(name = "TYPE", namespace = "http://schemas.datastream.net/MP_fields")
protected TYPE_Type type;
@XmlElement(name = "CLASSID", namespace = "http://schemas.datastream.net/MP_fields")
protected CLASSID_Type classid;
@XmlElement(name = "STATUS", namespace = "http://schemas.datastream.net/MP_fields")
protected STATUS_Type status;
@XmlElement(name = "DEPARTMENTID", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected DEPARTMENTID_Type departmentid;
@XmlElement(name = "CATEGORYID", namespace = "http://schemas.datastream.net/MP_fields")
protected CATEGORYID categoryid;
@XmlElement(name = "COSTCODEID", namespace = "http://schemas.datastream.net/MP_fields")
protected COSTCODEID_Type costcodeid;
@XmlElement(name = "STOREID", namespace = "http://schemas.datastream.net/MP_fields")
protected STOREID_Type storeid;
@XmlElement(name = "PARTID", namespace = "http://schemas.datastream.net/MP_fields")
protected PARTID_Type partid;
@XmlElement(name = "METERUNIT", namespace = "http://schemas.datastream.net/MP_fields")
protected String meterunit;
@XmlElement(name = "ManufacturerInfo")
protected ManufacturerInfo manufacturerInfo;
@XmlElement(name = "CNNUMBER", namespace = "http://schemas.datastream.net/MP_fields")
protected String cnnumber;
@XmlElement(name = "Variables")
protected Variables variables;
@XmlAttribute(name = "recordid")
protected Long recordid;
@XmlAttribute(name = "user_entity")
protected String user_Entity;
@XmlAttribute(name = "system_entity")
protected String system_Entity;
/**
* Gets the value of the profileid property.
*
* @return
* possible object is
* {@link OBJECT_Type }
*
*/
public OBJECT_Type getPROFILEID() {
return profileid;
}
/**
* Sets the value of the profileid property.
*
* @param value
* allowed object is
* {@link OBJECT_Type }
*
*/
public void setPROFILEID(OBJECT_Type value) {
this.profileid = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link TYPE_Type }
*
*/
public TYPE_Type getTYPE() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link TYPE_Type }
*
*/
public void setTYPE(TYPE_Type value) {
this.type = value;
}
/**
* Gets the value of the classid property.
*
* @return
* possible object is
* {@link CLASSID_Type }
*
*/
public CLASSID_Type getCLASSID() {
return classid;
}
/**
* Sets the value of the classid property.
*
* @param value
* allowed object is
* {@link CLASSID_Type }
*
*/
public void setCLASSID(CLASSID_Type value) {
this.classid = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link STATUS_Type }
*
*/
public STATUS_Type getSTATUS() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link STATUS_Type }
*
*/
public void setSTATUS(STATUS_Type value) {
this.status = value;
}
/**
* Gets the value of the departmentid property.
*
* @return
* possible object is
* {@link DEPARTMENTID_Type }
*
*/
public DEPARTMENTID_Type getDEPARTMENTID() {
return departmentid;
}
/**
* Sets the value of the departmentid property.
*
* @param value
* allowed object is
* {@link DEPARTMENTID_Type }
*
*/
public void setDEPARTMENTID(DEPARTMENTID_Type value) {
this.departmentid = value;
}
/**
* Gets the value of the categoryid property.
*
* @return
* possible object is
* {@link CATEGORYID }
*
*/
public CATEGORYID getCATEGORYID() {
return categoryid;
}
/**
* Sets the value of the categoryid property.
*
* @param value
* allowed object is
* {@link CATEGORYID }
*
*/
public void setCATEGORYID(CATEGORYID value) {
this.categoryid = value;
}
/**
* Gets the value of the costcodeid property.
*
* @return
* possible object is
* {@link COSTCODEID_Type }
*
*/
public COSTCODEID_Type getCOSTCODEID() {
return costcodeid;
}
/**
* Sets the value of the costcodeid property.
*
* @param value
* allowed object is
* {@link COSTCODEID_Type }
*
*/
public void setCOSTCODEID(COSTCODEID_Type value) {
this.costcodeid = value;
}
/**
* Gets the value of the storeid property.
*
* @return
* possible object is
* {@link STOREID_Type }
*
*/
public STOREID_Type getSTOREID() {
return storeid;
}
/**
* Sets the value of the storeid property.
*
* @param value
* allowed object is
* {@link STOREID_Type }
*
*/
public void setSTOREID(STOREID_Type value) {
this.storeid = value;
}
/**
* Gets the value of the partid property.
*
* @return
* possible object is
* {@link PARTID_Type }
*
*/
public PARTID_Type getPARTID() {
return partid;
}
/**
* Sets the value of the partid property.
*
* @param value
* allowed object is
* {@link PARTID_Type }
*
*/
public void setPARTID(PARTID_Type value) {
this.partid = value;
}
/**
* Gets the value of the meterunit property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMETERUNIT() {
return meterunit;
}
/**
* Sets the value of the meterunit property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMETERUNIT(String value) {
this.meterunit = value;
}
/**
* Gets the value of the manufacturerInfo property.
*
* @return
* possible object is
* {@link ManufacturerInfo }
*
*/
public ManufacturerInfo getManufacturerInfo() {
return manufacturerInfo;
}
/**
* Sets the value of the manufacturerInfo property.
*
* @param value
* allowed object is
* {@link ManufacturerInfo }
*
*/
public void setManufacturerInfo(ManufacturerInfo value) {
this.manufacturerInfo = value;
}
/**
* Gets the value of the cnnumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCNNUMBER() {
return cnnumber;
}
/**
* Sets the value of the cnnumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCNNUMBER(String value) {
this.cnnumber = value;
}
/**
* Gets the value of the variables property.
*
* @return
* possible object is
* {@link Variables }
*
*/
public Variables getVariables() {
return variables;
}
/**
* Sets the value of the variables property.
*
* @param value
* allowed object is
* {@link Variables }
*
*/
public void setVariables(Variables value) {
this.variables = value;
}
/**
* Gets the value of the recordid property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getRecordid() {
return recordid;
}
/**
* Sets the value of the recordid property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setRecordid(Long value) {
this.recordid = value;
}
/**
* Gets the value of the user_Entity property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUser_Entity() {
return user_Entity;
}
/**
* Sets the value of the user_Entity property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUser_Entity(String value) {
this.user_Entity = value;
}
/**
* Gets the value of the system_Entity property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSystem_Entity() {
return system_Entity;
}
/**
* Sets the value of the system_Entity property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSystem_Entity(String value) {
this.system_Entity = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy