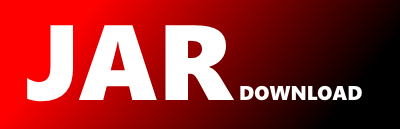
net.datastream.schemas.mp_entities.systemequipment_001.ChildSystem Maven / Gradle / Ivy
Show all versions of eam-wshub-proxyclient Show documentation
package net.datastream.schemas.mp_entities.systemequipment_001;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import net.datastream.schemas.mp_entities.assetequipment_001.ChildAsset;
import net.datastream.schemas.mp_entities.positionequipment_001.ChildPosition;
import net.datastream.schemas.mp_fields.DEPARTMENTID_Type;
import net.datastream.schemas.mp_fields.EQUIPMENTID_Type;
import net.datastream.schemas.mp_fields.TYPE_Type;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://schemas.datastream.net/MP_fields}SYSTEMID"/>
* <element ref="{http://schemas.datastream.net/MP_fields}TYPE"/>
* <element ref="{http://schemas.datastream.net/MP_fields}COSTROLLUP"/>
* <element ref="{http://schemas.datastream.net/MP_fields}DEPENDENTON"/>
* <element ref="{http://schemas.datastream.net/MP_fields}DEPARTMENTID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}OUTOFSERVICE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_entities/SystemEquipment_001}ChildSystem" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_entities/PositionEquipment_001}ChildPosition" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_entities/AssetEquipment_001}ChildAsset" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="recordid" type="{http://www.w3.org/2001/XMLSchema}long" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"systemid",
"type",
"costrollup",
"dependenton",
"departmentid",
"outofservice",
"childSystem",
"childPosition",
"childAsset"
})
@XmlRootElement(name = "ChildSystem")
public class ChildSystem {
@XmlElement(name = "SYSTEMID", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected EQUIPMENTID_Type systemid;
@XmlElement(name = "TYPE", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected TYPE_Type type;
@XmlElement(name = "COSTROLLUP", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected String costrollup;
@XmlElement(name = "DEPENDENTON", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected String dependenton;
@XmlElement(name = "DEPARTMENTID", namespace = "http://schemas.datastream.net/MP_fields")
protected DEPARTMENTID_Type departmentid;
@XmlElement(name = "OUTOFSERVICE", namespace = "http://schemas.datastream.net/MP_fields", defaultValue = "false")
protected String outofservice;
@XmlElement(name = "ChildSystem")
protected List childSystem;
@XmlElement(name = "ChildPosition", namespace = "http://schemas.datastream.net/MP_entities/PositionEquipment_001")
protected List childPosition;
@XmlElement(name = "ChildAsset", namespace = "http://schemas.datastream.net/MP_entities/AssetEquipment_001")
protected List childAsset;
@XmlAttribute(name = "recordid")
protected Long recordid;
/**
* Gets the value of the systemid property.
*
* @return
* possible object is
* {@link EQUIPMENTID_Type }
*
*/
public EQUIPMENTID_Type getSYSTEMID() {
return systemid;
}
/**
* Sets the value of the systemid property.
*
* @param value
* allowed object is
* {@link EQUIPMENTID_Type }
*
*/
public void setSYSTEMID(EQUIPMENTID_Type value) {
this.systemid = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link TYPE_Type }
*
*/
public TYPE_Type getTYPE() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link TYPE_Type }
*
*/
public void setTYPE(TYPE_Type value) {
this.type = value;
}
/**
* Gets the value of the costrollup property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCOSTROLLUP() {
return costrollup;
}
/**
* Sets the value of the costrollup property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCOSTROLLUP(String value) {
this.costrollup = value;
}
/**
* Gets the value of the dependenton property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDEPENDENTON() {
return dependenton;
}
/**
* Sets the value of the dependenton property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDEPENDENTON(String value) {
this.dependenton = value;
}
/**
* Gets the value of the departmentid property.
*
* @return
* possible object is
* {@link DEPARTMENTID_Type }
*
*/
public DEPARTMENTID_Type getDEPARTMENTID() {
return departmentid;
}
/**
* Sets the value of the departmentid property.
*
* @param value
* allowed object is
* {@link DEPARTMENTID_Type }
*
*/
public void setDEPARTMENTID(DEPARTMENTID_Type value) {
this.departmentid = value;
}
/**
* Gets the value of the outofservice property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOUTOFSERVICE() {
return outofservice;
}
/**
* Sets the value of the outofservice property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOUTOFSERVICE(String value) {
this.outofservice = value;
}
/**
* Gets the value of the childSystem property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the childSystem property.
*
*
* For example, to add a new item, do as follows:
*
* getChildSystem().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ChildSystem }
*
*
*/
public List getChildSystem() {
if (childSystem == null) {
childSystem = new ArrayList();
}
return this.childSystem;
}
/**
* Gets the value of the childPosition property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the childPosition property.
*
*
* For example, to add a new item, do as follows:
*
* getChildPosition().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ChildPosition }
*
*
*/
public List getChildPosition() {
if (childPosition == null) {
childPosition = new ArrayList();
}
return this.childPosition;
}
/**
* Gets the value of the childAsset property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the childAsset property.
*
*
* For example, to add a new item, do as follows:
*
* getChildAsset().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ChildAsset }
*
*
*/
public List getChildAsset() {
if (childAsset == null) {
childAsset = new ArrayList();
}
return this.childAsset;
}
/**
* Gets the value of the recordid property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getRecordid() {
return recordid;
}
/**
* Sets the value of the recordid property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setRecordid(Long value) {
this.recordid = value;
}
}