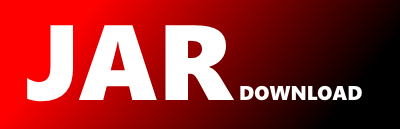
net.datastream.schemas.mp_results.mp0026_001.ResultData Maven / Gradle / Ivy
package net.datastream.schemas.mp_results.mp0026_001;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import net.datastream.schemas.mp_entities.workorder_001.ComplianceDetail;
import net.datastream.schemas.mp_entities.workorder_001.IncidentTracking;
import net.datastream.schemas.mp_fields.ORGANIZATIONID_Type;
import net.datastream.schemas.mp_fields.PERSONID_Type;
import net.datastream.schemas.mp_fields.STATUS_Type;
import net.datastream.schemas.mp_fields.TYPE_Type;
import net.datastream.schemas.mp_fields.WOTYPECATEGORYID_Type;
import org.openapplications.oagis_segments.DATETIME;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://schemas.datastream.net/MP_results/MP0026_001}JOBNUM"/>
* <element ref="{http://schemas.datastream.net/MP_fields}ORGANIZATIONID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}STATUS" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}TYPE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}ENTEREDBY"/>
* <element ref="{http://schemas.datastream.net/MP_fields}REQUESTEDBY" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}SHIFTCODE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}REPORTED"/>
* <element ref="{http://schemas.datastream.net/MP_fields}TARGETDATE"/>
* <element ref="{http://schemas.datastream.net/MP_fields}SCHEDEND"/>
* <element ref="{http://schemas.datastream.net/MP_fields}WORKDAY" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}PERTYPE" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CURRENCYCODE" minOccurs="0"/>
* <element name="GROUP" type="{http://schemas.datastream.net/MP_fields}CODE12_Type" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}CCTRSPCVALIDATION" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_fields}WOTYPECATEGORYID" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_entities/WorkOrder_001}ComplianceDetail" minOccurs="0"/>
* <element ref="{http://schemas.datastream.net/MP_entities/WorkOrder_001}IncidentTracking" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"jobnum",
"organizationid",
"status",
"type",
"enteredby",
"requestedby",
"shiftcode",
"reported",
"targetdate",
"schedend",
"workday",
"pertype",
"currencycode",
"group",
"cctrspcvalidation",
"wotypecategoryid",
"complianceDetail",
"incidentTracking"
})
@XmlRootElement(name = "ResultData")
public class ResultData {
@XmlElement(name = "JOBNUM", required = true)
protected String jobnum;
@XmlElement(name = "ORGANIZATIONID", namespace = "http://schemas.datastream.net/MP_fields")
protected ORGANIZATIONID_Type organizationid;
@XmlElement(name = "STATUS", namespace = "http://schemas.datastream.net/MP_fields")
protected STATUS_Type status;
@XmlElement(name = "TYPE", namespace = "http://schemas.datastream.net/MP_fields")
protected TYPE_Type type;
@XmlElement(name = "ENTEREDBY", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected String enteredby;
@XmlElement(name = "REQUESTEDBY", namespace = "http://schemas.datastream.net/MP_fields")
protected PERSONID_Type requestedby;
@XmlElement(name = "SHIFTCODE", namespace = "http://schemas.datastream.net/MP_fields")
protected String shiftcode;
@XmlElement(name = "REPORTED", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected DATETIME reported;
@XmlElement(name = "TARGETDATE", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected DATETIME targetdate;
@XmlElement(name = "SCHEDEND", namespace = "http://schemas.datastream.net/MP_fields", required = true)
protected DATETIME schedend;
@XmlElement(name = "WORKDAY", namespace = "http://schemas.datastream.net/MP_fields")
protected String workday;
@XmlElement(name = "PERTYPE", namespace = "http://schemas.datastream.net/MP_fields")
protected String pertype;
@XmlElement(name = "CURRENCYCODE", namespace = "http://schemas.datastream.net/MP_fields")
protected String currencycode;
@XmlElement(name = "GROUP")
protected String group;
@XmlElement(name = "CCTRSPCVALIDATION", namespace = "http://schemas.datastream.net/MP_fields")
protected String cctrspcvalidation;
@XmlElement(name = "WOTYPECATEGORYID", namespace = "http://schemas.datastream.net/MP_fields")
protected WOTYPECATEGORYID_Type wotypecategoryid;
@XmlElement(name = "ComplianceDetail", namespace = "http://schemas.datastream.net/MP_entities/WorkOrder_001")
protected ComplianceDetail complianceDetail;
@XmlElement(name = "IncidentTracking", namespace = "http://schemas.datastream.net/MP_entities/WorkOrder_001")
protected IncidentTracking incidentTracking;
/**
* Gets the value of the jobnum property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJOBNUM() {
return jobnum;
}
/**
* Sets the value of the jobnum property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJOBNUM(String value) {
this.jobnum = value;
}
/**
* Gets the value of the organizationid property.
*
* @return
* possible object is
* {@link ORGANIZATIONID_Type }
*
*/
public ORGANIZATIONID_Type getORGANIZATIONID() {
return organizationid;
}
/**
* Sets the value of the organizationid property.
*
* @param value
* allowed object is
* {@link ORGANIZATIONID_Type }
*
*/
public void setORGANIZATIONID(ORGANIZATIONID_Type value) {
this.organizationid = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link STATUS_Type }
*
*/
public STATUS_Type getSTATUS() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link STATUS_Type }
*
*/
public void setSTATUS(STATUS_Type value) {
this.status = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link TYPE_Type }
*
*/
public TYPE_Type getTYPE() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link TYPE_Type }
*
*/
public void setTYPE(TYPE_Type value) {
this.type = value;
}
/**
* Gets the value of the enteredby property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getENTEREDBY() {
return enteredby;
}
/**
* Sets the value of the enteredby property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setENTEREDBY(String value) {
this.enteredby = value;
}
/**
* Gets the value of the requestedby property.
*
* @return
* possible object is
* {@link PERSONID_Type }
*
*/
public PERSONID_Type getREQUESTEDBY() {
return requestedby;
}
/**
* Sets the value of the requestedby property.
*
* @param value
* allowed object is
* {@link PERSONID_Type }
*
*/
public void setREQUESTEDBY(PERSONID_Type value) {
this.requestedby = value;
}
/**
* Gets the value of the shiftcode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSHIFTCODE() {
return shiftcode;
}
/**
* Sets the value of the shiftcode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSHIFTCODE(String value) {
this.shiftcode = value;
}
/**
* Gets the value of the reported property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getREPORTED() {
return reported;
}
/**
* Sets the value of the reported property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setREPORTED(DATETIME value) {
this.reported = value;
}
/**
* Gets the value of the targetdate property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getTARGETDATE() {
return targetdate;
}
/**
* Sets the value of the targetdate property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setTARGETDATE(DATETIME value) {
this.targetdate = value;
}
/**
* Gets the value of the schedend property.
*
* @return
* possible object is
* {@link DATETIME }
*
*/
public DATETIME getSCHEDEND() {
return schedend;
}
/**
* Sets the value of the schedend property.
*
* @param value
* allowed object is
* {@link DATETIME }
*
*/
public void setSCHEDEND(DATETIME value) {
this.schedend = value;
}
/**
* Gets the value of the workday property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWORKDAY() {
return workday;
}
/**
* Sets the value of the workday property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWORKDAY(String value) {
this.workday = value;
}
/**
* Gets the value of the pertype property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPERTYPE() {
return pertype;
}
/**
* Sets the value of the pertype property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPERTYPE(String value) {
this.pertype = value;
}
/**
* Gets the value of the currencycode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCURRENCYCODE() {
return currencycode;
}
/**
* Sets the value of the currencycode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCURRENCYCODE(String value) {
this.currencycode = value;
}
/**
* Gets the value of the group property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGROUP() {
return group;
}
/**
* Sets the value of the group property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGROUP(String value) {
this.group = value;
}
/**
* Gets the value of the cctrspcvalidation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCCTRSPCVALIDATION() {
return cctrspcvalidation;
}
/**
* Sets the value of the cctrspcvalidation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCCTRSPCVALIDATION(String value) {
this.cctrspcvalidation = value;
}
/**
* Gets the value of the wotypecategoryid property.
*
* @return
* possible object is
* {@link WOTYPECATEGORYID_Type }
*
*/
public WOTYPECATEGORYID_Type getWOTYPECATEGORYID() {
return wotypecategoryid;
}
/**
* Sets the value of the wotypecategoryid property.
*
* @param value
* allowed object is
* {@link WOTYPECATEGORYID_Type }
*
*/
public void setWOTYPECATEGORYID(WOTYPECATEGORYID_Type value) {
this.wotypecategoryid = value;
}
/**
* Gets the value of the complianceDetail property.
*
* @return
* possible object is
* {@link ComplianceDetail }
*
*/
public ComplianceDetail getComplianceDetail() {
return complianceDetail;
}
/**
* Sets the value of the complianceDetail property.
*
* @param value
* allowed object is
* {@link ComplianceDetail }
*
*/
public void setComplianceDetail(ComplianceDetail value) {
this.complianceDetail = value;
}
/**
* Gets the value of the incidentTracking property.
*
* @return
* possible object is
* {@link IncidentTracking }
*
*/
public IncidentTracking getIncidentTracking() {
return incidentTracking;
}
/**
* Sets the value of the incidentTracking property.
*
* @param value
* allowed object is
* {@link IncidentTracking }
*
*/
public void setIncidentTracking(IncidentTracking value) {
this.incidentTracking = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy