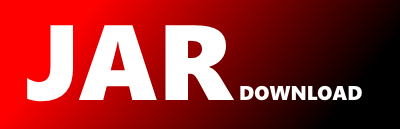
ch.cern.ZKPattern Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cerndb-sw-zkpolicy Show documentation
Show all versions of cerndb-sw-zkpolicy Show documentation
zkpolicy - ZooKeeper Policy Auditing Tool
/*
* Copyright © 2020, CERN
* This software is distributed under the terms of the MIT Licence,
* copied verbatim in the file 'LICENSE'. In applying this licence,
* CERN does not waive the privileges and immunities
* granted to it by virtue of its status as an Intergovernmental Organization
* or submit itself to any jurisdiction.
*/
package ch.cern;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Pattern;
public class ZKPattern {
/**
* Create a list of Pattern objects from a list of glob expression strings.
*
* @param globStringList Glob expression List
* @return List of Pattern objects
*/
public static List createGlobPatternList(List globStringList) {
List queryPatternList = new ArrayList();
for (String queryGlob : globStringList) {
String regexFromGlob = globToRegex(queryGlob);
Pattern patternRegex = Pattern.compile(regexFromGlob);
queryPatternList.add(patternRegex);
}
return queryPatternList;
}
/**
* Create a list of Pattern objects from a list of regular expression strings.
*
* @param regexStringList
* @return List of Pattern objects
*/
public static List createRegexPatternList(List regexStringList) {
List queryPatternList = new ArrayList();
for (String queryRegEx : regexStringList) {
Pattern patternRegex = Pattern.compile(queryRegEx);
queryPatternList.add(patternRegex);
}
return queryPatternList;
}
private static String globToRegex(String pattern) {
StringBuilder sb = new StringBuilder(pattern.length());
int inGroup = 0;
int inClass = 0;
int firstIndexInClass = -1;
char[] arr = pattern.toCharArray();
for (int i = 0; i < arr.length; i++) {
char ch = arr[i];
switch (ch) {
case '\\':
if (++i >= arr.length) {
sb.append('\\');
} else {
char next = arr[i];
switch (next) {
case ',':
// escape not needed
break;
case 'Q':
case 'E':
// extra escape needed
sb.append('\\');
sb.append('\\');
break;
default:
sb.append('\\');
}
sb.append(next);
}
break;
case '*':
if (inClass == 0)
sb.append(".*");
else
sb.append('*');
break;
case '?':
if (inClass == 0)
sb.append('.');
else
sb.append('?');
break;
case '[':
inClass++;
firstIndexInClass = i + 1;
sb.append('[');
break;
case ']':
inClass--;
sb.append(']');
break;
case '.':
case '(':
case ')':
case '+':
case '|':
case '^':
case '$':
case '@':
case '%':
if (inClass == 0 || firstIndexInClass == i && ch == '^')
sb.append('\\');
sb.append(ch);
break;
case '!':
if (firstIndexInClass == i)
sb.append('^');
else
sb.append('!');
break;
case '{':
inGroup++;
sb.append('(');
break;
case '}':
inGroup--;
sb.append(')');
break;
case ',':
if (inGroup > 0)
sb.append('|');
else
sb.append(',');
break;
default:
sb.append(ch);
}
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy