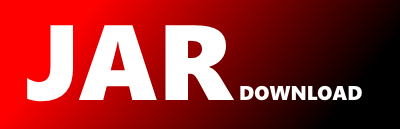
ch.cern.ZKPolicyUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cerndb-sw-zkpolicy Show documentation
Show all versions of cerndb-sw-zkpolicy Show documentation
zkpolicy - ZooKeeper Policy Auditing Tool
/*
* Copyright © 2020, CERN
* This software is distributed under the terms of the MIT Licence,
* copied verbatim in the file 'LICENSE'. In applying this licence,
* CERN does not waive the privileges and immunities
* granted to it by virtue of its status as an Intergovernmental Organization
* or submit itself to any jurisdiction.
*/
package ch.cern;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
import java.util.concurrent.CountDownLatch;
import org.apache.zookeeper.WatchedEvent;
import org.apache.zookeeper.Watcher;
import org.apache.zookeeper.Watcher.Event.KeeperState;
public class ZKPolicyUtils {
/**
* Construct output buffer for a list of query elements.
*
* @param queryElements Queries to be executed
* @return Output buffer
*/
public static Hashtable> getQueryOutputBuffer(List queryElements) {
Hashtable> returnOutput = new Hashtable>();
for (ZKQueryElement queryElement : queryElements) {
// Construct the output buffer HashMap
returnOutput.put(queryElement.hashCode(), new ArrayList());
}
return returnOutput;
}
/**
* Construct output buffer for a list of check elements.
*
* @param checkElements Checks to be executed
* @return Output buffer
*/
public static Hashtable> getChecksOutputBuffer(List checkElements) {
Hashtable> returnOutput = new Hashtable>();
for (ZKCheckElement checkElement : checkElements) {
// Construct the output buffer HashMap
returnOutput.put(checkElement.hashCode(), new ArrayList());
}
return returnOutput;
}
/**
* Watcher for ZooKeeper client connection that blocks waiting for succesful
* connection.
*/
public static class ConnectedWatcher implements Watcher {
private CountDownLatch connectedLatch;
ConnectedWatcher(CountDownLatch connectedLatch) {
this.connectedLatch = connectedLatch;
}
@Override
public void process(WatchedEvent event) {
if (event.getState() == KeeperState.SyncConnected) {
connectedLatch.countDown();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy