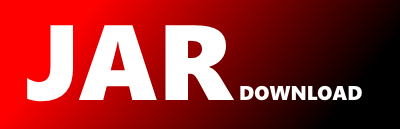
ch.codeblock.qrinvoice.boofcv.BoofQrCodeReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qrinvoice-boofcv Show documentation
Show all versions of qrinvoice-boofcv Show documentation
BoofCV module contains a BoofCV based implementation for detecting/decoding QR codes
The newest version!
package ch.codeblock.qrinvoice.boofcv;
import boofcv.abst.fiducial.QrCodeDetector;
import boofcv.alg.fiducial.qrcode.QrCode;
import boofcv.factory.fiducial.ConfigQrCode;
import boofcv.factory.fiducial.FactoryFiducial;
import boofcv.io.image.ConvertBufferedImage;
import boofcv.struct.image.GrayU8;
import ch.codeblock.qrinvoice.qrcode.IQrCodeReader;
import ch.codeblock.qrinvoice.qrcode.SwissQrCode;
import ch.codeblock.qrinvoice.qrcode.SwissQrCodeFilter;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.awt.image.BufferedImage;
import java.util.List;
import java.util.Optional;
import static java.util.stream.Collectors.toList;
public class BoofQrCodeReader implements IQrCodeReader {
private static final Logger LOGGER = LoggerFactory.getLogger(BoofQrCodeReader.class);
private final ConfigQrCode config;
public BoofQrCodeReader() {
this.config = new ConfigQrCode();
config.versionMinimum = SwissQrCode.QR_CODE_MIN_VERSION;
config.versionMaximum = SwissQrCode.QR_CODE_MAX_VERSION;
}
public Optional readSingleSwissPaymentsCode(final BufferedImage image) {
return readAllInternally(image).stream().findFirst();
}
public List readAllSwissPaymentCodes(final BufferedImage image) {
return readAllInternally(image);
}
private List readAllInternally(BufferedImage image) {
final long start = System.currentTimeMillis();
final QrCodeDetector detector = FactoryFiducial.qrcode(config, GrayU8.class);
detector.process(ConvertBufferedImage.convertFrom(image, (GrayU8) null));
if (LOGGER.isDebugEnabled()) {
for (QrCode failure : detector.getFailures()) {
LOGGER.debug("QR Code detection failures, failureCause={}", failure.failureCause);
}
}
// Gets a list of all the qr codes it could successfully detect and decode
final List detections = detector.getDetections();
final long end = System.currentTimeMillis();
LOGGER.debug("took {} ms to readAll image w={} h={}", (end - start), image.getWidth(), image.getHeight());
return detections.stream()
.map(d -> d.message)
.filter(SwissQrCodeFilter::filter)
.distinct()
.collect(toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy