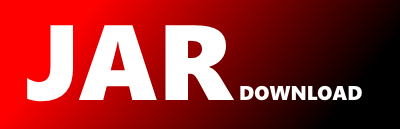
ch.codeblock.qrinvoice.pdf.PdfMerger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qrinvoice-openpdf Show documentation
Show all versions of qrinvoice-openpdf Show documentation
Provides an OpenPDF specific payment part receipt writer
/*-
* #%L
* QR Invoice Solutions
* %%
* Copyright (C) 2017 - 2022 Codeblock GmbH
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
* -
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
* -
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
* -
* Other licenses:
* -----------------------------------------------------------------------------
* Commercial licenses are available for this software. These replace the above
* AGPLv3 terms and offer support, maintenance and allow the use in commercial /
* proprietary products.
* -
* More information on commercial licenses are available at the following page:
* https://www.qr-invoice.ch/licenses/
* #L%
*/
package ch.codeblock.qrinvoice.pdf;
import ch.codeblock.qrinvoice.TechnicalException;
import com.lowagie.text.Document;
import com.lowagie.text.DocumentException;
import com.lowagie.text.pdf.PdfCopy;
import com.lowagie.text.pdf.PdfReader;
import com.lowagie.text.pdf.PdfSmartCopy;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class PdfMerger extends AbstractPdfMerger implements AutoCloseable {
public static PdfMerger create() {
return new PdfMerger();
}
private final ByteArrayOutputStream baos;
final Document document;
final PdfCopy copy;
public PdfMerger() {
baos = new ByteArrayOutputStream();
document = new Document();
copy = new PdfSmartCopy(document, baos);
document.open();
}
@Override
public void close() {
copy.close();
if (document.isOpen()) {
document.close();
}
}
public PdfMerger addPdf(final byte[] data) {
return addPdf(data, false);
}
// TODO implement test
public PdfMerger addPdf(final byte[] data, boolean exceptLastPage) {
try {
final PdfReader pdfReaderOne = new PdfReader(data);
int numberOfPages = pdfReaderOne.getNumberOfPages();
if (exceptLastPage) {
numberOfPages--;
}
importPdf(pdfReaderOne, copy, numberOfPages).freeReader(pdfReaderOne);
pdfReaderOne.close();
} catch (DocumentException | IOException e) {
throw new TechnicalException("Unable to append PDF", e);
}
return this;
}
public byte[] getPdf() {
close();
return baos.toByteArray();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy