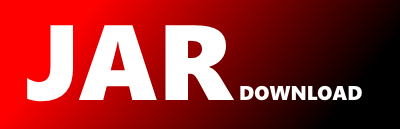
ch.codeblock.qrinvoice.qrcode.IText4QrCodeWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qrinvoice-openpdf Show documentation
Show all versions of qrinvoice-openpdf Show documentation
Provides an OpenPDF specific payment part receipt writer
/*-
* #%L
* QR Invoice Solutions
* %%
* Copyright (C) 2017 - 2022 Codeblock GmbH
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
* -
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
* -
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
* -
* Other licenses:
* -----------------------------------------------------------------------------
* Commercial licenses are available for this software. These replace the above
* AGPLv3 terms and offer support, maintenance and allow the use in commercial /
* proprietary products.
* -
* More information on commercial licenses are available at the following page:
* https://www.qr-invoice.ch/licenses/
* #L%
*/
package ch.codeblock.qrinvoice.qrcode;
import ch.codeblock.qrinvoice.OutputFormat;
import ch.codeblock.qrinvoice.TechnicalException;
import ch.codeblock.qrinvoice.layout.DimensionUnitUtils;
import ch.codeblock.qrinvoice.layout.Rect;
import ch.codeblock.qrinvoice.output.QrCode;
import com.lowagie.text.Document;
import com.lowagie.text.DocumentException;
import com.lowagie.text.Image;
import com.lowagie.text.Rectangle;
import com.lowagie.text.pdf.PdfContentByte;
import com.lowagie.text.pdf.PdfWriter;
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import static ch.codeblock.qrinvoice.layout.DimensionUnitUtils.millimetersToPoints;
public class IText4QrCodeWriter implements IQrCodeWriter {
private static final Rect QR_CODE_RECTANGLE;
static {
QR_CODE_RECTANGLE = Rect.create(0F, 0F, millimetersToPoints(SwissQrCode.QR_CODE_SIZE.getWidth()), millimetersToPoints(SwissQrCode.QR_CODE_SIZE.getHeight()));
}
private final QrCodeWriterOptions options;
public IText4QrCodeWriter(final QrCodeWriterOptions options) {
this.options = options;
}
@Override
public int getQrCodeImageSize() {
final int dpi = options.getOutputResolution().getDpi();
final float qrCodeMillimeters = SwissQrCode.QR_CODE_SIZE.getWidth();
// millimeters to pixels
return DimensionUnitUtils.millimetersToPointsRounded(qrCodeMillimeters, dpi);
}
@Override
public QrCode write(final String qrCodeString) {
final QrCodeWriterOptions qrCodeWriterOptions = new QrCodeWriterOptions(options.getOutputFormat(), getQrCodeImageSize());
final BufferedImage qrCodeImage = JavaGraphicsQrCodeWriter.create(qrCodeWriterOptions).writeBufferedImage(qrCodeString);
try {
final ByteArrayOutputStream baos = new ByteArrayOutputStream(25 * 1024);
final Rectangle pageCanvas = new Rectangle(QR_CODE_RECTANGLE.getWidth(), QR_CODE_RECTANGLE.getHeight());
final Document document = new Document(pageCanvas, 0, 0, 0, 0);
final PdfWriter writer = PdfWriter.getInstance(document, baos);
document.open();
addQrCodeImage(writer, qrCodeImage);
document.close();
return new QrCode(OutputFormat.PDF, baos.toByteArray(), null, null);
} catch (DocumentException | IOException e) {
throw new TechnicalException("Unexpected exception occurred during the creation of the qr code", e);
}
}
private void addQrCodeImage(final PdfWriter writer, final BufferedImage qrCodeImage) throws IOException, DocumentException {
final Image image = Image.getInstance(qrCodeImage, null);
image.setAbsolutePosition(QR_CODE_RECTANGLE.getLowerLeftX(), QR_CODE_RECTANGLE.getLowerLeftY());
final float size = QR_CODE_RECTANGLE.getWidth();
image.scaleToFit(size, size);
final PdfContentByte canvas = writer.getDirectContent();
canvas.addImage(image);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy