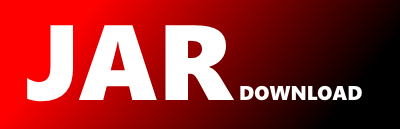
ch.codeblock.qrinvoice.pdf.QrPdfMerger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qrinvoice-openpdf Show documentation
Show all versions of qrinvoice-openpdf Show documentation
Provides an OpenPDF specific payment part receipt writer
/*-
* #%L
* QR Invoice Solutions
* %%
* Copyright (C) 2017 - 2023 Codeblock GmbH
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
* -
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
* -
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
* -
* Other licenses:
* -----------------------------------------------------------------------------
* Commercial licenses are available for this software. These replace the above
* AGPLv3 terms and offer support, maintenance and allow the use in commercial /
* proprietary products.
* -
* More information on commercial licenses are available at the following page:
* https://www.qr-invoice.ch/licenses/
* #L%
*/
package ch.codeblock.qrinvoice.pdf;
import ch.codeblock.qrinvoice.TechnicalException;
import com.lowagie.text.Document;
import com.lowagie.text.DocumentException;
import com.lowagie.text.Rectangle;
import com.lowagie.text.pdf.*;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.List;
public class QrPdfMerger extends AbstractPdfMerger {
public static QrPdfMerger create() {
return new QrPdfMerger();
}
public byte[] mergePdfs(byte[] inputPdf, byte[] paymentPartReceiptPdf) throws IOException, TechnicalException {
return mergePdfs(inputPdf, paymentPartReceiptPdf, 1);
}
public byte[] mergePdfs(byte[] inputPdf, byte[] paymentPartReceiptPdf, int onPage) throws IOException, TechnicalException {
final PdfReader reader = new PdfReader(inputPdf);
final ByteArrayOutputStream baos = new ByteArrayOutputStream();
final PdfStamper stamper;
try {
stamper = new PdfStamper(reader, baos);
final PdfContentByte canvas = stamper.getOverContent(onPage);
final Rectangle basePage = reader.getPageSize(1);
final PdfReader r = new PdfReader(paymentPartReceiptPdf);
final PdfImportedPage paymentPartPage = stamper.getImportedPage(r, 1);
final float x = basePage.getWidth() - paymentPartPage.getWidth();
canvas.addTemplate(paymentPartPage, x, 0);
stamper.getWriter().freeReader(r);
r.close();
stamper.close();
} catch (DocumentException e) {
throw new TechnicalException("Unable to merge PDFs", e);
}
return baos.toByteArray();
}
public byte[] appendPdfs(List data) throws IOException, TechnicalException {
final ByteArrayOutputStream baos = new ByteArrayOutputStream();
final Document document = new Document();
final PdfCopy copy;
try {
copy = new PdfSmartCopy(document, baos);
document.open();
for (byte[] dataLoop : data) {
final PdfReader pdfReaderOne = new PdfReader(dataLoop);
importPdf(pdfReaderOne, copy).freeReader(pdfReaderOne);
pdfReaderOne.close();
}
document.close();
} catch (DocumentException e) {
throw new TechnicalException("Unable to append PDFs", e);
}
return baos.toByteArray();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy