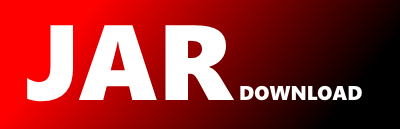
ch.dkitc.ridioc.DIConstructor Maven / Gradle / Ivy
package ch.dkitc.ridioc;
import java.lang.annotation.Annotation;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import com.thoughtworks.paranamer.AdaptiveParanamer;
import com.thoughtworks.paranamer.Paranamer;
public class DIConstructor {
private final Constructor> constructor;
private final Paranamer paranamer = new AdaptiveParanamer();
private final Map, Class>> wrappedPrimitiveTypeMap;
public DIConstructor(Constructor> constructor, Map, Class>> wrappedPrimitiveTypeMap) {
this.constructor = constructor;
this.wrappedPrimitiveTypeMap = wrappedPrimitiveTypeMap;
}
public String getName() {
return constructor.getDeclaringClass().getName();
}
public boolean matchesParams(Object ... givenParams) {
List> givenParamTypes = new ArrayList>();
for (Object givenParam : givenParams) {
if (givenParam == null) {
throw new IllegalArgumentException("Given param must NOT be null");
}
givenParamTypes.add(givenParam.getClass());
}
return matchesParamTypes(givenParamTypes.toArray(new Class[givenParamTypes.size()]));
}
public boolean matchesParamTypes(Class>... givenParamTypes) {
Class>[] constructorParamTypesArray = constructor.getParameterTypes();
if (givenParamTypes.length != constructorParamTypesArray.length) {
// leave early
return false;
}
for (int i=0; i paramType = constructorParamTypesArray[i];
Class> wrappedParamType;
if (paramType.isPrimitive()) {
wrappedParamType = wrappedPrimitiveTypeMap.get(paramType);
if (wrappedParamType == null) {
throw new IllegalStateException("there is no wrapped type available for primitive type '" + paramType + '"');
}
} else {
wrappedParamType = paramType;
}
if (!wrappedParamType.isAssignableFrom(givenParamTypes[i])) {
return false;
}
}
// if we're here, everything is alrite!
return true;
}
public T newInstance(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy