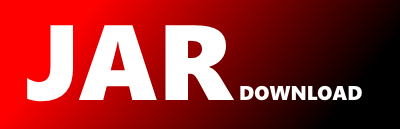
ch.inftec.ju.util.context.GenericContextUtils Maven / Gradle / Ivy
package ch.inftec.ju.util.context;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import ch.inftec.ju.util.JuStringUtils;
/**
* GenericContextUtils contains utility methods related to the GenericContext interface.
*
* @author tgdmemae
*
*/
public final class GenericContextUtils {
/**
* Don't instantiate.
*/
private GenericContextUtils() {
throw new AssertionError("use only statically");
}
/**
* Provides a builder to construct GenericContext instances.
* @return GenericContext instance
*/
public static GenericContextBuilder builder() {
return new GenericContextBuilder();
}
/**
* Wraps the specified context in a GenericContextX instance. If the
* context is already an instance of GenericContextX, the same reference
* is returned.
* @param context GenericContextX wrapper around the context
* @return GenericContextX working on the specified context.
*/
public static GenericContextX asX(GenericContext context) {
if (context instanceof GenericContextX) return (GenericContextX)context;
else return new GenericContextX(context);
}
/**
* Implementation of the GenericContext interface using Object lists and
* ObjectEvaluators.
*
* @author Martin
*
*/
private static final class GenericContextImpl implements GenericContext {
/**
* HashMap containing the object lists for the class types.
*/
HashMap, ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy