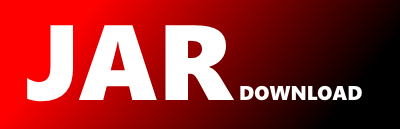
ch.poole.osm.presetutils.MultiHashMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of preset-utils Show documentation
Show all versions of preset-utils Show documentation
Assorted utils for JOSM style presets
package ch.poole.osm.presetutils;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.TreeSet;
/**
* This data structure is a combination of a Map and Set.
* Each key can be assigned not one, but multiple values.
* Sorted map/set implementations are used to guarantee (case-sensitive) alphabetical sorting of entries.
* @author Jan
*
* @param Key type
* @param Type of the values to be associated with the keys
*/
public class MultiHashMap implements Serializable {
/**
*
*/
private static final long serialVersionUID = 1L;
private Map> map;
private boolean sorted;
/**
* Creates a regular, unsorted MultiHashMap
*/
public MultiHashMap() {
this(false);
}
/**
* Creates a MultiHashMap.
*
* @param sorted if true, Tree maps/sets will be used, if false, regular HashMap/HashSets will be used.
*/
public MultiHashMap(boolean sorted) {
this.sorted = sorted;
if (sorted) {
map = new TreeMap<>();
} else {
map = new HashMap<>();
}
}
/**
* Check for key in map
*
* @param key the key we are looking for
* @return true if key exists in map
*/
public boolean containsKey(K key) {
return map.containsKey(key);
}
/**
* Adds item to the set of values associated with the key (null items are not added)
*
* @param key key to add
* @param item item to add
* @return true if the element was added, false if it was already in the set or null
*/
public boolean add(K key, V item) {
Set values = map.get(key);
if (values == null) {
values = (sorted ? new TreeSet() : new HashSet());
map.put(key, values);
}
if (item == null) return false;
return values.add(item);
}
/**
* Adds all items to the set of values associated with the key
*
* @param key the key
* @param items an array containing the items
*/
public void add(K key, V[] items) {
Set values = map.get(key);
if (values == null) {
values = (sorted ? new TreeSet() : new HashSet());
map.put(key, values);
}
values.addAll(Arrays.asList(items));
}
/**
* Adds all items to the set of values associated with the key
*
* @param key the key
* @param items a set containing the items
*/
public void add(K key, Set items) {
Set values = map.get(key);
if (values == null) {
values = (sorted ? new TreeSet() : new HashSet());
map.put(key, values);
}
values.addAll(items);
}
/**
* Removes the item from the set associated with the given key
*
* @param key the key of the item to remove
* @param item the item to remove
* @return true if the item was in the set
*/
public boolean removeItem(K key, V item) {
Set values = map.get(key);
if (values != null) return values.remove(item);
return false;
}
/**
* Completely removes all values associated with a key
*
* @param key key to remove all items for
*/
public void removeKey(K key) {
map.remove(key);
}
/**
* Gets the list of items associated with a key.
*
* @param key key we want the values for
* @return a unmodifiable list of the items associated with the key, may be empty but never null
*/
public Set get(K key) {
Set values = map.get(key);
if (values == null) return Collections.emptySet();
return Collections.unmodifiableSet(values);
}
/**
* Guess what.
*/
public void clear() {
map.clear();
}
/**
* Get all keys as a Set
*
* @return a Set containing the keys
*/
public Set getKeys() {
return map.keySet();
}
/**
* Return all values
*
* @return a Set of all values
*/
public Set getValues() {
Set retval = new LinkedHashSet<>();
for (K key: getKeys()) {
retval.addAll(get(key));
}
return retval;
}
/**
* Add all key/values from source to this Map
*
* @param source add all entries from thiw MultiHashMap
*/
public void addAll(MultiHashMap source) {
for (K key:source.getKeys()) {
add(key,source.get(key));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy