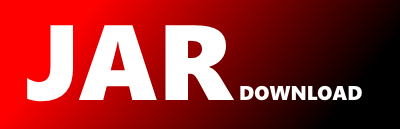
ch.poole.osm.presetutils.Preset2Html Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of preset-utils Show documentation
Show all versions of preset-utils Show documentation
Assorted utils for JOSM style presets
package ch.poole.osm.presetutils;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.Map;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.HelpFormatter;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
/**
* Make a (semi-)nice HTML page out of the presets
*
* Licence Apache 2.0
*
* @author Simon Poole
*
*/
public class Preset2Html {
private static final String TRUE = "true";
private static final String LIST_ENTRY_ELEMENT = "list_entry";
private static final String REFERENCE_ELEMENT = "reference";
private static final String ROLE_ELEMENT = "role";
private static final String PRESET_LINK_ELEMENT = "preset_link";
private static final String TEXT_ELEMENT = "text";
private static final String CHECK_ELEMENT = "check";
private static final String COMBO_ELEMENT = "combo";
private static final String MULTISELECT_ELEMENT = "multiselect";
private static final String KEY_ELEMENT = "key";
private static final String OPTIONAL_ELEMENT = "optional";
private static final String LABEL_ELEMENT = "label";
private static final String SEPARATOR_ELEMENT = "separator";
private static final String PRESETS_ELEMENT = "presets";
private static final String GROUP_ELEMENT = "group";
private static final String CHUNK_ELEMENT = "chunk";
private static final String ITEM_ELEMENT = "item";
private static final String DEPRECATED_ATTRIBUTE = "deprecated";
private static final String SHORTDESCRIPTION_ATTRIBUTE = "shortdescription";
private static final String ID_ATTRIBUTE = "id";
private static final String ICON_ATTRIBUTE = "icon";
private static final String REF_ATTRIBUTE = "ref";
private static final String KEY_ATTRIBUTE = "key";
private static final String NAME_ATTRIBUTE = "name";
private static final String PRESET_NAME_ATTRIBUTE = "preset_name";
private static final String VALUE_ATTRIBUTE = "value";
private static final String REGIONS_ATTRIBUTE = "regions";
private static final String EXCLUDE_REGIONS_ATTRIBUTE = "exclude_regions";
private static final int GROUP_INDENT = 30;
HashMap> msgs = new HashMap<>();
String inputFilename;
String vespucciLink = null;
String josmLink = null;
int groupCount = 0;
void parseXML(final InputStream input, final PrintWriter pw) throws ParserConfigurationException, SAXException, IOException {
SAXParser saxParser = SAXParserFactory.newInstance().newSAXParser();
pw.write(
"");
pw.write("");
pw.write("");
pw.write("");
pw.write("");
pw.write("");
saxParser.parse(input, new DefaultHandler() {
String group = null;
String preset = null;
String regions = null;
String chunk = null;
Map chunkKeys = new HashMap<>();
Map chunkOptionalKeys = new HashMap<>();
Map chunkLinks = new HashMap<>();
Map chunkRoles = new HashMap<>();
String icon = null;
String icon2 = null;
String keys = null;
String optionalKeys = null;
String links = null;
String roles = null;
boolean optional = false;
StringBuilder buffer = new StringBuilder();
boolean deprecated = false;
boolean separator = false;
/**
* ${@inheritDoc}.
*/
@Override
public void startElement(String uri, String localName, String name, Attributes attr) throws SAXException {
switch (name) {
case PRESETS_ELEMENT:
String shortdescription = attr.getValue(SHORTDESCRIPTION_ATTRIBUTE);
if (shortdescription == null) {
pw.write("Presets from File " + inputFilename + "
\n");
} else {
pw.write("" + shortdescription + "
\n");
}
if (vespucciLink != null) {
try {
pw.write("Download link for Vespucci
\n");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
if (josmLink != null) {
pw.write("\n");
}
pw.write("");
break;
case GROUP_ELEMENT:
groupCount++;
group = attr.getValue(NAME_ATTRIBUTE);
buffer.append("");
String groupIcon = attr.getValue(ICON_ATTRIBUTE);
if (groupIcon != null && !"".equals(groupIcon)) {
String groupIcon2 = groupIcon.replace("${ICONPATH}", "icons/png/");
groupIcon2 = groupIcon2.replace("${ICONTYPE}", "png");
if (!groupIcon.equals(groupIcon2)) {
buffer.append("
");
}
}
buffer.append("" + group);
String groupRegions = getRegions(attr, true);
if (groupRegions != null) {
buffer.append(" " + groupRegions);
}
buffer.append(" \n");
if (groupCount == 1) {
pw.write("" + group + getRegions(attr, true) + " ");
}
break;
case ITEM_ELEMENT:
preset = attr.getValue(NAME_ATTRIBUTE);
regions = getRegions(attr, true);
String deprecatedStr = attr.getValue(DEPRECATED_ATTRIBUTE);
deprecated = deprecatedStr != null && TRUE.equals(deprecatedStr);
icon = attr.getValue(ICON_ATTRIBUTE);
if (icon != null && !"".equals(icon)) {
icon2 = icon.replace("${ICONPATH}", "icons/png/");
icon2 = icon2.replace("${ICONTYPE}", "png");
}
break;
case CHUNK_ELEMENT:
chunk = attr.getValue(ID_ATTRIBUTE);
keys = "";
break;
case SEPARATOR_ELEMENT:
break;
case LABEL_ELEMENT:
break;
case OPTIONAL_ELEMENT:
optional = true;
break;
case KEY_ELEMENT:
case MULTISELECT_ELEMENT:
case COMBO_ELEMENT:
case CHECK_ELEMENT:
case TEXT_ELEMENT:
if (!optional) {
keys = addTags(keys, attr);
} else {
optionalKeys = addTags(optionalKeys, attr);
}
break;
case PRESET_LINK_ELEMENT:
String link = attr.getValue(PRESET_NAME_ATTRIBUTE);
if (link != null) {
if (links == null) {
links = link;
} else {
links = links + "
" + link;
}
}
break;
case ROLE_ELEMENT:
String role = attr.getValue(KEY_ATTRIBUTE);
if (role != null && !"".equals(role)) {
if (roles == null) {
roles = role;
} else {
roles = roles + "
" + role;
}
}
break;
case REFERENCE_ELEMENT:
String ref = attr.getValue(REF_ATTRIBUTE);
String refKeys = chunkKeys.get(ref);
if (refKeys != null) {
if (!optional) {
if (keys != null) {
keys = keys + refKeys;
} else {
keys = refKeys;
}
} else {
if (optionalKeys != null) {
optionalKeys = optionalKeys + refKeys;
} else {
optionalKeys = refKeys;
}
}
}
String refOptionalKeys = chunkOptionalKeys.get(ref);
if (refOptionalKeys != null) {
if (optionalKeys != null) {
optionalKeys = optionalKeys + refOptionalKeys;
} else {
optionalKeys = refOptionalKeys;
}
}
String refLinks = chunkLinks.get(ref);
if (refLinks != null) {
if (links != null) {
links = links + refLinks;
} else {
links = refLinks;
}
}
String refRoles = chunkRoles.get(ref);
if (refRoles != null) {
if (roles != null) {
roles = roles + refLinks;
} else {
roles = refRoles;
}
}
if ((refKeys == null || "".equals(refKeys)) && (refOptionalKeys == null || "".equals(refOptionalKeys))
&& (refLinks == null || "".equals(refLinks)) && (refRoles == null || "".equals(refRoles))) {
System.err.println(ref + " was not found for preset " + preset);
}
break;
case LIST_ENTRY_ELEMENT:
default:
// nothing
}
}
private String addTags(String result, Attributes attr) {
String key = attr.getValue(KEY_ATTRIBUTE);
String value = attr.getValue(VALUE_ATTRIBUTE);
if (key != null && !"".equals(key)) {
String deprecatedStr = attr.getValue(DEPRECATED_ATTRIBUTE);
boolean fieldDeprecated = deprecatedStr != null && TRUE.equals(deprecatedStr);
String tag = "" + key + "=" + (value != null ? value : "*") + getRegions(attr, false)
+ (fieldDeprecated ? " deprecated" : "") + "";
if (result != null) {
result = result + tag;
} else {
result = tag;
}
}
return result;
}
private String getRegions(Attributes attr, boolean withDiv) {
String reg = attr.getValue(REGIONS_ATTRIBUTE);
if (reg != null) {
String result = "[" + reg + "]";
if (TRUE.equals(attr.getValue(EXCLUDE_REGIONS_ATTRIBUTE))) {
result = "!" + result;
}
if (withDiv) {
return " " + result + "";
}
return " " + result;
}
return "";
}
@Override
public void endElement(String uri, String localMame, String name) throws SAXException {
switch (name) {
case GROUP_ELEMENT:
group = null;
buffer.append("\n");
groupCount--;
break;
case OPTIONAL_ELEMENT:
optional = false;
break;
case ITEM_ELEMENT:
if (preset != null) {
if (separator) {
buffer.append("");
separator = false;
} else {
buffer.append("");
}
if (icon != null && !"".equals(icon)) {
if (!icon2.equals(icon)) {
buffer.append("
" + preset.replace("/", " / "));
} else {
buffer.append("" + preset.replace("/", " / "));
}
} else {
buffer.append("" + preset.replace("/", " / "));
}
buffer.append(" " + regions);
buffer.append(isDeprecated());
buffer.append("");
appendKeys();
buffer.append("");
preset = null;
}
keys = null;
optionalKeys = null;
links = null;
break;
case CHUNK_ELEMENT:
if (chunk != null) {
if (keys != null) {
chunkKeys.put(chunk, keys);
}
if (optionalKeys != null) {
chunkOptionalKeys.put(chunk, optionalKeys);
}
if (links != null) {
chunkLinks.put(chunk, links);
}
if (roles != null) {
chunkRoles.put(chunk, roles);
}
} else {
System.err.println("chunk null");
}
keys = null;
optionalKeys = null;
chunk = null;
links = null;
break;
case SEPARATOR_ELEMENT:
separator = true;
break;
case COMBO_ELEMENT:
case MULTISELECT_ELEMENT:
default:
// nothing
}
}
private String isDeprecated() {
return deprecated ? "deprecated" : "";
}
private void appendKeys() {
if (keys != null) {
buffer.append("
" + keys);
if (optionalKeys != null) {
buffer.append("Optional:
");
buffer.append(optionalKeys);
}
if (links != null) {
buffer.append("Links:
");
buffer.append(links);
}
buffer.append("");
}
}
@Override
public void endDocument() {
pw.write(buffer.toString());
pw.write("
© 2015 - 2024 Weber Informatics LLC | Privacy Policy