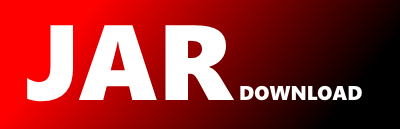
ch.racic.testing.cm.AggregatedResourceBundle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of test-configuration-manager Show documentation
Show all versions of test-configuration-manager Show documentation
A configuration manager tailored for functional testing with TestNG.
The newest version!
/*
* Copyleft (c) 2015. This code is for learning purposes only.
* Do whatever you like with it but don't take it as perfect code.
* //Michel Racic (http://rac.su/+)//
*/
package ch.racic.testing.cm;
import java.util.*;
/**
* A {@link java.util.ResourceBundle} whose content is aggregated from multiple source bundles. Code taken from
* hibernate-validator source
*
* @author Gunnar Morling
*/
public class AggregatedResourceBundle extends ResourceBundle {
private final Map contents = new HashMap();
/**
* Creates a new AggregatedResourceBundle.
*
* @param bundles A list of source bundles, which shall be merged into one aggregated bundle. The newly created
* bundle will contain all keys from all source bundles. In case a key occurs in multiple source
* bundles, the value will be taken from the first bundle containing the key.
*/
public AggregatedResourceBundle(List bundles) {
if (bundles != null) {
for (ResourceBundle bundle : bundles) {
mergeOverride(bundle);
}
}
}
public AggregatedResourceBundle() {
}
public AggregatedResourceBundle(AggregatedResourceBundle toCopy) {
if (toCopy!= null && toCopy.contents!=null) {
this.contents.putAll(toCopy.contents);
}
}
/**
* Merge the new bundle into the existing while overriding already existing keys.
*
* @param bundle
*/
public synchronized void mergeOverride(ResourceBundle bundle) {
Enumeration keys = bundle.getKeys();
while (keys.hasMoreElements()) {
String oneKey = keys.nextElement();
contents.put(oneKey, bundle.getObject(oneKey));
}
}
@Override
public Enumeration getKeys() {
return new IteratorEnumeration(contents.keySet().iterator());
}
@Override
public boolean containsKey(String key){
return contents.containsKey(key);
}
@Override
public Set keySet(){
return contents.keySet();
}
/**
* Get the full map of properties
*
* @return
*/
public Map getMap() {
return contents;
}
@Override
protected Object handleGetObject(String key) {
return contents.get(key);
}
/**
* Merge the new bundle into the existing while overriding already existing keys.
*
* @param params
*/
public synchronized void mergeOverride(Map params) {
contents.putAll(params);
}
public synchronized void mergeOverride(Properties props) {
for (String key : props.stringPropertyNames()) {
contents.put(key, props.getProperty(key));
}
}
/**
* An {@link java.util.Enumeration} implementation, that wraps an {@link java.util.Iterator}. Can be used to
* integrate older APIs working with enumerations with iterators.
*
* @param The enumerated type.
* @author Gunnar Morling
*/
private static class IteratorEnumeration implements Enumeration {
private final Iterator source;
/**
* Creates a new IterationEnumeration.
*
* @param source The source iterator. Must not be null.
*/
public IteratorEnumeration(Iterator source) {
if (source == null) {
throw new IllegalArgumentException("Source must not be null");
}
this.source = source;
}
public boolean hasMoreElements() {
return source.hasNext();
}
public T nextElement() {
return source.next();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy