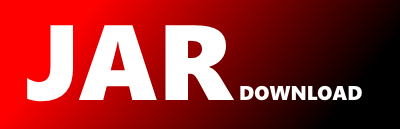
ch.raffael.doclets.pegdown.package-info Maven / Gradle / Ivy
/*
* Copyright 2013 Raffael Herzog
*
* This file is part of pegdown-doclet.
*
* pegdown-doclet is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* pegdown-doclet is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with pegdown-doclet. If not, see .
*/
/**
* A Doclet that allows the use of Markdown in JavaDoc and
* [PlantUML](http://plantuml.sourceforge.net/) comments. It uses
* [Pegdown](http://www.pegdown.org/) as Markdown processor. It's a simple preprocessor to
* the standard Doclet: It processes all JavaDoc comments in the documentation tree and
* then forwards the result to the standard Doclet.
*
* This Doclet is released under the
* [GPL 3.0](http://www.gnu.org/licenses/gpl-3.0-standalone.html).
*
*
* Leading Spaces
* --------------
*
* Sometimes, leading whitespaces are significant in Markdown. Because of the way we
* usually write JavaDoc comments and the way JavaDoc is implemented, this may lead to
* some problems:
*
* ```
* /**
* * Title
* * =====
* *
* * Text
* *{@literal /}
* ```
*
* In this example, each line has one leading space. Because of this, the title won't be
* recognised as such by Pegdown. To work around this problem, the Doclet uses a simple
* trick: The first leading space character (the *actual* space character, i.e. `\\u0020`)
* will be cut off, if it exists.
*
* This may be important e.g. for code blocks, which should be indented by 4 spaces: Well,
* it's 5 spaces now. ;)
*
* *Note:** If an `overview.md` file is specified, leading spaces will be treated normally
* in this file. The first space will *not* be ignored.
*
* This behaviour is currently *not* customisable.
*
*
* Javadoc Tags
* ------------
*
* The following known tags handled by Pegdown so you can use Markup with them:
*
* * `@author`
* * `@version`
* * `@return`
* * `@deprecated`
* * `@since`
* * `@param`
* * `@throws`
*
* ### `@see` Tags
*
* The `@see` tag is a special case, as there are several variants of this tag. These two
* variants will remain unchanged:
*
* * Javadoc-Links: `@see Foo#bar()`
* * Links: `@see Example`
*
* The third variant however, which is originally meant to refer to a printed book, may
* also contain Markdown-style links:
*
* * `@see "[Example](http://www.example.com/)"`
* * `@see " "`
* * `@see "Example "`
* * `@see "[[http://www.example.com/]]"`
* * `@see "[[http://www.example.com/ Example]]"`
*
* These are all rendered as `@see LABEL`, where
* LABEL falls back to the link's URL, if no label is given.
*
* **Warning:** Version 1.2 of this doclet will redefine Wiki-Style links (see
* [issue #7](https://github.com/Abnaxos/pegdown-doclet/issues/7)). It's recommended not
* to use them for now.
*
* ### Custom Tag Handling
*
* Tag handling can be customised by implementing your own `TagRenderer`s and registering
* them with the PegdownDoclet. You'll have to write your own Doclet, though, there's
* currently no way to do this using the command line. See the JavaDocs and sources for
* details on this.
*
* This currently only works for block tags.
*
* ### Inline Tags
*
* Inline tags will be removed before processing the Markdown source and re-inserted
* afterwards. Therefore, markup within inline tags won't work.
*
* There's currently no way to customise this behaviour or customize the way inline tags
* are rendered back into the processed doc comment.
*
*
* PlantUML
* --------
*
* This Doclet has built-in support for PlantUML. Just use the `@uml` tag:
*
* ```
* /**
* * Description.
* *
* * 
* *
* * @uml example.png
* * Alice -> Bob: Authentication Request
* * Bob --> Alice: Authentication Response
* *{@literal /}
* ```
*
* It's also possible to use `@startuml` and `@enduml` instead, as usual. `@startuml` is
* simply a synonym for `@uml` and `@enduml` will be ignored entirely. Use this for
* compatibility with other tools, like e.g. the
* [PlantUML IDEA Plugin](https://github.com/esteinberg/plantuml4idea).
*
*
* Syntax Highlighting
* -------------------
*
* The Pegdown Doclet integrates
* [highlight.js](http://softwaremaniacs.org/soft/highlight/en/) to enable syntax
* highlighting in code examples. See "Fenced code blocks" below for details.
*
*
* Invoking
* --------
*
* Specify the Doclet on JavaDoc's command line:
*
* ```
* javadoc -doclet ch.raffael.doclets.pegdown.PegdownDoclet -docletpath /path/to/pegdown-doclet-1.1-all.jar
* ```
*
* A prebuilt version can be downloaded from http://maven.raffael.ch/ch/raffael/pegdown-doclet/pegdown-doclet/
* (use the JAR with the suffix "-all" for a JAR file that includes all dependencies).
*
* It supports all options the standard Doclet supports and some additional options:
*
* `-extensions `
* : Specify the Pegdown extensions. The extensions list a comma
* separated list of constants as specified in
* [org.pegdown.Extensions](http://www.decodified.com/pegdown/api/org/pegdown/Extensions.html),
* converted to upper case and '-' replaced by '_'. The default is
* `autolinks,definitions,smartypants,tables,wikilinks`.
*
* `-overview `
* : Specify an overview page. This is basically the same as with the
* standard Doclet, however, the specified page will be rendered using Pegdown.
*
* `-plantuml-config `
* : A configuration file that will be included before each diagram.
*
* `-highlight-style