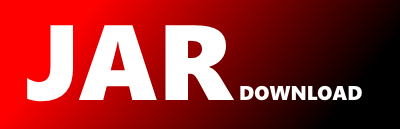
org.jhotdraw8.application.AbstractApplication Maven / Gradle / Ivy
/*
* @(#)AbstractApplication.java
* Copyright © 2023 The authors and contributors of JHotDraw. MIT License.
*/
package org.jhotdraw8.application;
import javafx.beans.binding.Bindings;
import javafx.beans.property.IntegerProperty;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.ReadOnlyBooleanProperty;
import javafx.beans.property.ReadOnlyBooleanWrapper;
import javafx.beans.property.ReadOnlyListProperty;
import javafx.beans.property.ReadOnlyListWrapper;
import javafx.beans.property.ReadOnlyMapProperty;
import javafx.beans.property.ReadOnlyMapWrapper;
import javafx.beans.property.ReadOnlySetProperty;
import javafx.beans.property.ReadOnlySetWrapper;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.property.SimpleObjectProperty;
import javafx.collections.FXCollections;
import javafx.collections.MapChangeListener;
import javafx.collections.ObservableMap;
import javafx.collections.ObservableSet;
import javafx.scene.control.MenuBar;
import javafx.scene.input.DataFormat;
import org.jhotdraw8.annotation.NonNull;
import org.jhotdraw8.application.action.Action;
import org.jhotdraw8.application.resources.EmptyResources;
import org.jhotdraw8.application.resources.Resources;
import org.jhotdraw8.fxbase.beans.NonNullObjectProperty;
import org.jhotdraw8.fxcollection.typesafekey.Key;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.concurrent.Executor;
import java.util.concurrent.ForkJoinPool;
import java.util.function.Supplier;
import java.util.logging.Level;
import java.util.logging.Logger;
import java.util.prefs.Preferences;
/**
* AbstractApplication.
*
* @author Werner Randelshofer
*/
public abstract class AbstractApplication extends javafx.application.Application implements org.jhotdraw8.application.Application {
private static final @NonNull String RECENT_URIS = ".recentUriFormats";
/**
* Holds the disabled state.
*/
private final @NonNull ReadOnlyBooleanProperty disabled;
/**
* Holds the disablers.
*/
private final @NonNull ObservableSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy