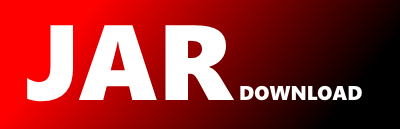
org.jhotdraw8.application.Application Maven / Gradle / Ivy
/*
* @(#)Application.java
* Copyright © 2023 The authors and contributors of JHotDraw. MIT License.
*/
package org.jhotdraw8.application;
import javafx.beans.property.IntegerProperty;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.ReadOnlyListProperty;
import javafx.beans.property.ReadOnlyMapProperty;
import javafx.beans.property.ReadOnlyObjectProperty;
import javafx.beans.property.ReadOnlySetProperty;
import javafx.collections.ObservableList;
import javafx.collections.ObservableMap;
import javafx.collections.ObservableSet;
import javafx.scene.Node;
import javafx.scene.control.MenuBar;
import javafx.scene.input.DataFormat;
import org.jhotdraw8.annotation.NonNull;
import org.jhotdraw8.annotation.Nullable;
import org.jhotdraw8.application.action.Action;
import org.jhotdraw8.application.resources.Resources;
import org.jhotdraw8.fxbase.beans.NonNullObjectProperty;
import org.jhotdraw8.fxbase.beans.PropertyBean;
import org.jhotdraw8.fxbase.concurrent.FXWorker;
import org.jhotdraw8.fxbase.control.Disableable;
import org.jhotdraw8.fxcollection.typesafekey.Key;
import org.jhotdraw8.fxcollection.typesafekey.NullableObjectKey;
import java.net.URI;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Executor;
import java.util.function.Supplier;
import java.util.prefs.Preferences;
/**
* An {@code Application} handles the life-cycle of {@link Activity} objects and
* provides windows to present them on screen.
*
* @author Werner Randelshofer
*/
public interface Application extends Disableable, PropertyBean {
String ACTIONS_PROPERTY = "actions";
String ACTIVITIES_PROPERTY = "activities";
String ACTIVITY_FACTORY_PROPERTY = "activityFactory";
String RESOURCE_BUNDLE_PROPERTY = "resourceBundle";
String MENU_BAR_FACTORY_PROPERTY = "menuBarFactory";
String RECENT_URIS_PROPERTY = "recentUris";
String PREFERENCES_PROPERTY = "preferences";
String MAX_NUMBER_OF_RECENT_URIS_PROPERTY = "maxNumberOfRecentUris";
String STYLESHEETS_PROPERTY = "stylesheets";
Key NAME_KEY = new NullableObjectKey<>("name", String.class);
Key VERSION_KEY = new NullableObjectKey<>("version", String.class);
Key COPYRIGHT_KEY = new NullableObjectKey<>("copyright", String.class);
Key LICENSE_KEY = new NullableObjectKey<>("license", String.class);
/**
* Contains all {@link Activity} objects that are managed by this
* {@link Application}.
*
* @return the activities
*/
@NonNull ReadOnlySetProperty activitiesProperty();
Executor getExecutor();
@NonNull NonNullObjectProperty preferencesProperty();
/**
* Contains all {@link Action} objects that are managed by this
* {@link Application}.
*
* @return the activities
*/
@NonNull ReadOnlyMapProperty actionsProperty();
default @NonNull ObservableMap getActions() {
return actionsProperty().get();
}
default @NonNull Preferences getPreferences() {
return preferencesProperty().get();
}
default void setPreferences(@NonNull Preferences preferences) {
preferencesProperty().set(preferences);
}
/**
* The set of recent URIs. The set must be ordered by most recently used
* first. Only the first items as specified in
* {@link #maxNumberOfRecentUrisProperty} of the set are used and persisted
* in user preferences.
*
* @return the recent Uris
*/
ReadOnlyMapProperty recentUrisProperty();
default @NonNull ObservableMap getRecentUris() {
return recentUrisProperty().get();
}
/**
* The maximal number of recent URIs. Specifies how many items of
* {@link #recentUrisProperty} are used and persisted in user preferences.
* This number is also persisted in user preferences.
*
* @return the number of recent Uris
*/
@NonNull IntegerProperty maxNumberOfRecentUrisProperty();
// Convenience method
default @NonNull ObservableSet getActivities() {
return activitiesProperty().get();
}
/**
* Provides the currently active activities. This is the last activities which was
* focus owner. Returns null, if the application has no views.
*
* @return The active activities.
*/
ReadOnlyObjectProperty activeActivityProperty();
// Convenience method
default @Nullable Activity getActiveActivity() {
return activeActivityProperty().get();
}
/**
* Exits the application.
*/
void exit();
/**
* Returns the application node.
*
* @return the node
*/
default @Nullable Node getNode() {
return null;
}
/**
* Creates a new activity, initializes it, then invokes the callback.
*
* @return A callback.
*/
default CompletionStage createActivity() {
return FXWorker.supply(() -> {
Supplier factory = getActivityFactory();
if (factory == null) {
throw new IllegalStateException("Could not create a new Activity, because no activityFactory has been set.");
}
return factory.get();
});
}
default int getMaxNumberOfRecentUris() {
return maxNumberOfRecentUrisProperty().get();
}
default void setMaxNumberOfRecentUris(int newValue) {
maxNumberOfRecentUrisProperty().set(newValue);
}
@NonNull ObjectProperty> activityFactoryProperty();
default Supplier getActivityFactory() {
return activityFactoryProperty().get();
}
default void setActivityFactory(Supplier newValue) {
activityFactoryProperty().set(newValue);
}
@NonNull ObjectProperty> menuBarFactoryProperty();
@NonNull NonNullObjectProperty resourcesProperty();
default @Nullable Supplier getMenuBarFactory() {
return menuBarFactoryProperty().get();
}
@NonNull ReadOnlyListProperty stylesheetsProperty();
default @NonNull ObservableList getStylesheets() {
return stylesheetsProperty().get();
}
default void setMenuBarFactory(@Nullable Supplier newValue) {
menuBarFactoryProperty().set(newValue);
}
default @NonNull Resources getResources() {
return resourcesProperty().get();
}
default void setResources(@NonNull Resources newValue) {
resourcesProperty().set(newValue);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy