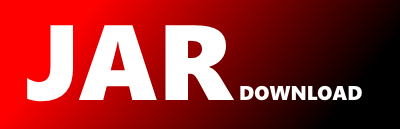
org.jhotdraw8.collection.pair.OrderedPair Maven / Gradle / Ivy
package org.jhotdraw8.collection.pair;
import org.jspecify.annotations.Nullable;
import java.util.Objects;
/**
* An ordered pair.
*
* This is a value-type.
*
* @param the type of the first element of the pair
* @param the type of the second element of the pair
* @author Werner Randelshofer
*/
public interface OrderedPair {
U first();
V second();
/**
* Checks if a given ordered pair is equal to a given object.
*
* @param pair an ordered pair
* @param obj an object
* @param the type of the first element of the pair
* @param the type of the second element of the pair
* @return true if equal
*/
static boolean orderedPairEquals(OrderedPair pair, @Nullable Object obj) {
if (pair == obj) {
return true;
}
if (obj == null) {
return false;
}
if (pair.getClass() != obj.getClass()) {
return false;
}
final OrderedPair, ?> other = (OrderedPair, ?>) obj;
if (!Objects.equals(pair.first(), other.first())) {
return false;
}
return Objects.equals(pair.second(), other.second());
}
/**
* Computes a hash code for an ordered pair.
* The hash code is guaranteed to be non-zero.
*
* @param pair an ordered pair
* @param the type of the first element of the pair
* @param the type of the second element of the pair
* @return the hash code
*/
static int orderedPairHashCode(OrderedPair pair) {
int hash = 3;
hash = 59 * hash + Objects.hashCode(pair.first());
hash = 59 * hash + Objects.hashCode(pair.second());
return hash == 0 ? -89 : hash;
}
}