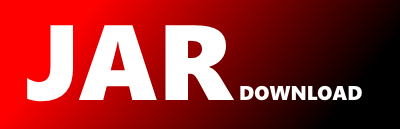
org.jhotdraw8.icollection.facade.ImmutableSetFacade Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jhotdraw8.icollection Show documentation
Show all versions of org.jhotdraw8.icollection Show documentation
JHotDraw8 Immutable Collections
The newest version!
/*
* @(#)ImmutableSetFacade.java
* Copyright © 2023 The authors and contributors of JHotDraw. MIT License.
*/
package org.jhotdraw8.icollection.facade;
import org.jhotdraw8.icollection.immutable.ImmutableSet;
import org.jhotdraw8.icollection.impl.iteration.IteratorSpliterator;
import org.jhotdraw8.icollection.impl.iteration.Iterators;
import org.jhotdraw8.icollection.readonly.AbstractReadOnlySet;
import org.jhotdraw8.icollection.readonly.ReadOnlyCollection;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.Set;
import java.util.Spliterator;
import java.util.function.Function;
/**
* Provides a {@link ImmutableSet} facade to a set of {@code ImmutableSet} functions.
*
* @param the element type
*/
public class ImmutableSetFacade extends AbstractReadOnlySet implements ImmutableSet {
private final Set target;
private final Function, Set> cloneFunction;
public ImmutableSetFacade(Set target, Function, Set> cloneFunction) {
this.target = target;
this.cloneFunction = cloneFunction;
}
@SuppressWarnings("unchecked")
@Override
public ImmutableSet empty() {
return new ImmutableSetFacade<>(new LinkedHashSet<>(), k -> (Set) ((LinkedHashSet>) k).clone());
}
@Override
public ImmutableSet add(E element) {
Set clone = cloneFunction.apply(target);
return clone.add(element) ? new ImmutableSetFacade<>(clone, cloneFunction) : this;
}
@Override
public ImmutableSet addAll(Iterable extends E> c) {
Set clone = cloneFunction.apply(target);
boolean changed = false;
for (E e : c) {
changed |= clone.add(e);
}
return changed ? new ImmutableSetFacade<>(clone, cloneFunction) : this;
}
@Override
public ImmutableSet remove(E element) {
Set clone = cloneFunction.apply(target);
return clone.remove(element) ? new ImmutableSetFacade<>(clone, cloneFunction) : this;
}
@Override
public ImmutableSet removeAll(Iterable> c) {
Set clone = cloneFunction.apply(target);
boolean changed = false;
for (Object e : c) {
changed |= clone.remove(e);
}
return changed ? new ImmutableSetFacade<>(clone, cloneFunction) : this;
}
@SuppressWarnings("unchecked")
@Override
public ImmutableSet retainAll(Iterable> c) {
if (isEmpty()) {
return this;
}
Set clone = cloneFunction.apply(target);
Collection collection;
if (c instanceof ReadOnlyCollection> rc) {
collection = (Collection) rc.asCollection();
} else if (c instanceof Collection> cc) {
collection = (Collection) cc;
} else {
collection = new HashSet<>();
c.forEach(e -> collection.add((E) e));
}
return clone.retainAll(collection) ? new ImmutableSetFacade<>(clone, cloneFunction) : this;
}
@Override
public int size() {
return target.size();
}
@Override
public boolean contains(Object o) {
return target.contains(o);
}
@Override
public int maxSize() {
return Integer.MAX_VALUE;
}
@Override
public Iterator iterator() {
return Iterators.unmodifiableIterator(target.iterator());
}
@Override
public Spliterator spliterator() {
return new IteratorSpliterator<>(iterator(), size(), characteristics(), null);
}
@Override
public Set toMutable() {
return cloneFunction.apply(target);
}
@Override
public int characteristics() {
return Spliterator.IMMUTABLE | super.characteristics();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy