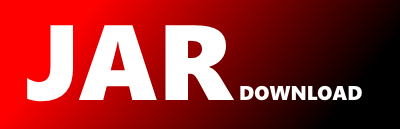
org.jhotdraw8.icollection.facade.ListFacade Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jhotdraw8.icollection Show documentation
Show all versions of org.jhotdraw8.icollection Show documentation
JHotDraw8 Immutable Collections
The newest version!
/*
* @(#)ListFacade.java
* Copyright © 2023 The authors and contributors of JHotDraw. MIT License.
*/
package org.jhotdraw8.icollection.facade;
import org.jhotdraw8.icollection.readonly.ReadOnlyList;
import org.jspecify.annotations.Nullable;
import java.util.AbstractList;
import java.util.List;
import java.util.SequencedCollection;
import java.util.Spliterator;
import java.util.function.BiConsumer;
import java.util.function.IntFunction;
import java.util.function.IntSupplier;
import java.util.stream.Stream;
/**
* Provides a {@link List} facade to a set of {@code List} functions.
*
* @param the element type
* @author Werner Randelshofer
*/
public class ListFacade extends AbstractList
implements SequencedCollection {
private final IntSupplier sizeFunction;
private final IntFunction getFunction;
private final BiConsumer addFunction;
private final IntFunction removeFunction;
private final Runnable clearFunction;
public ListFacade(ReadOnlyList backingList) {
this(backingList::size, backingList::get, null, null, null);
}
public ListFacade(List backingList) {
this(backingList::size, backingList::get, backingList::clear,
backingList::add, backingList::remove);
}
public ListFacade(IntSupplier sizeFunction,
IntFunction getFunction
) {
this(sizeFunction, getFunction, null, null, null);
}
public ListFacade(IntSupplier sizeFunction,
IntFunction getFunction,
@Nullable Runnable clearFunction,
@Nullable BiConsumer addFunction,
@Nullable IntFunction removeFunction
) {
this.sizeFunction = sizeFunction;
this.getFunction = getFunction;
this.addFunction = addFunction == null ? (i, e) -> {
throw new UnsupportedOperationException();
} : addFunction;
this.clearFunction = clearFunction == null ? () -> {
throw new UnsupportedOperationException();
} : clearFunction;
this.removeFunction = removeFunction == null ? (i) -> {
throw new UnsupportedOperationException();
} : removeFunction;
}
@Override
public E get(int index) {
return getFunction.apply(index);
}
@Override
public Spliterator spliterator() {
return super.spliterator();
}
@Override
public E remove(int index) {
return removeFunction.apply(index);
}
@Override
public int size() {
return sizeFunction.getAsInt();
}
@Override
public void addFirst(E e) {
addFunction.accept(0, e);
}
@Override
public void addLast(E e) {
addFunction.accept(size(), e);
}
@Override
public Stream stream() {
return super.stream();
}
@Override
public List reversed() {
return new ListFacade<>(
sizeFunction,
i -> get(size() - i - 1),
clearFunction,
(i, e) -> add(size() - i, e),
(i) -> remove(size() - i - i)
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy