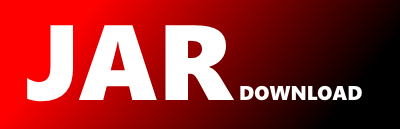
org.jhotdraw8.icollection.immutable.ImmutableList Maven / Gradle / Ivy
Show all versions of org.jhotdraw8.icollection Show documentation
/*
* @(#)ImmutableList.java
* Copyright © 2023 The authors and contributors of JHotDraw. MIT License.
*/
package org.jhotdraw8.icollection.immutable;
import org.jhotdraw8.icollection.readonly.ReadOnlyList;
import org.jspecify.annotations.Nullable;
import java.util.List;
/**
* An interface to an immutable list; the implementation guarantees that the state of the collection does not change.
*
* An interface to an immutable list provides methods for creating a new immutable list with
* added or removed elements, without changing the original immutable list.
*
* @param the element type
*/
public interface ImmutableList extends ReadOnlyList, ImmutableSequencedCollection {
/**
* Returns a copy of this list that is empty.
*
* @return this list instance if it is already empty, or a different list
* instance that is empty.
*/
@SuppressWarnings("unchecked")
@Override
ImmutableList empty();
@Override
ImmutableList addFirst(@Nullable final E element);
@Override
ImmutableList addLast(@Nullable final E element);
@Override
default ImmutableList removeFirst() {
return (ImmutableList) ImmutableSequencedCollection.super.removeFirst();
}
@Override
default ImmutableList removeLast() {
return (ImmutableList) ImmutableSequencedCollection.super.removeLast();
}
/**
* Returns a copy of this list that contains all elements
* of this list and the specified element appended to the
* end of the list.
*
* @param element an element
* @return a different list instance with the element added
*/
@Override
ImmutableList add(E element);
/**
* Returns a copy of this list that contains all elements
* of this list and the specified element appended to the
* end of the list.
*
* @param index the insertion index
* @param element an element
* @return a different list instance with the element added
*/
ImmutableList add(int index, E element);
/**
* Returns a copy of this list that contains all elements
* of this list and all elements of the specified
* collection appended.
*
* @param c a collection to be added to this list
* @return a different list instance with the elements added
*/
@Override
ImmutableList addAll(Iterable extends E> c);
/**
* Returns a copy of this list that contains all elements
* of this list and all elements of the specified
* collection appended.
*
* @param index the insertion index
* @param c a collection to be added to this list
* @return a different list instance with the elements added
*/
ImmutableList addAll(int index, Iterable extends E> c);
/**
* Returns a copy of this list that contains all elements
* of this list except the specified element.
*
* @param element an element
* @return this list instance if it already does not contain the element, or
* a different list instance with the element removed
*/
@Override
ImmutableList remove(E element);
/**
* Returns a copy of this list that contains all elements
* of this list except the element at the specified index
*
* @param index an index
* @return a different list instance with the element removed
*/
ImmutableList removeAt(int index);
/**
* Returns a copy of this list that contains all elements
* of this list except the elements in the specified range.
*
* @param fromIndex from index (inclusive) of the sub-list
* @param toIndex to index (exclusive) of the sub-list
* @return a different list instance with the element removed
*/
ImmutableList removeRange(int fromIndex, int toIndex);
/**
* Returns a copy of this list that contains all elements
* of this list except the elements of the specified
* collection.
*
* @param c a collection with elements to be removed from this set
* @return this list instance if it already does not contain the elements, or
* a different list instance with the elements removed
*/
@Override
ImmutableList removeAll(Iterable> c);
/**
* Returns a copy of this list that contains only elements
* that are in this list and in the specified collection.
*
* @param c a collection with elements to be retained in this set
* @return this list instance if it has not changed, or
* a different list instance with elements removed
*/
@Override
ImmutableList retainAll(Iterable> c);
/**
* Returns a reversed copy of this list.
*
* This operation may be implemented in O(N).
*
* Use {@link #readOnlyReversed()} if you only
* need to iterate in the reversed sequence over this list.
*
* @return a reversed copy of this list.
*/
ImmutableList reverse();
/**
* Returns a copy of this list that contains all elements
* of this list and the specified element replaced.
*
* @param element an element
* @return this list instance if it has not changed, or
* a different list instance with the element changed
*/
ImmutableList set(int index, E element);
/**
* Returns a copy of this list that contains only
* the elements in the given index range.
*
* @param fromIndex from index (inclusive) of the sub-list
* @param toIndex to index (exclusive) of the sub-list
* @return this list instance if it has not changed, or
* a different list instance with the element changed
*/
@Override
ImmutableList readOnlySubList(int fromIndex, int toIndex);
/**
* Returns a mutable copy of this list.
*
* @return a mutable copy.
*/
List toMutable();
}