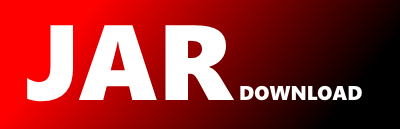
org.jhotdraw8.icollection.immutable.ImmutableSequencedSet Maven / Gradle / Ivy
Show all versions of org.jhotdraw8.icollection Show documentation
/*
* @(#)ImmutableSequencedSet.java
* Copyright © 2023 The authors and contributors of JHotDraw. MIT License.
*/
package org.jhotdraw8.icollection.immutable;
import org.jspecify.annotations.Nullable;
import org.jhotdraw8.icollection.readonly.ReadOnlySequencedSet;
import java.util.NoSuchElementException;
import java.util.SequencedSet;
/**
* An interface to an immutable set with a well-defined iteration order; the
* implementation guarantees that the state of the collection does not change.
*
* An interface to an immutable sequenced set provides methods for creating a new immutable sequenced set with
* added or removed elements, without changing the original immutable sequenced set.
*
* @param the element type
*/
public interface ImmutableSequencedSet extends ImmutableSet, ReadOnlySequencedSet, ImmutableSequencedCollection {
@Override
ImmutableSequencedSet add(E element);
@Override
default ImmutableSequencedSet addAll(Iterable extends E> c) {
return (ImmutableSequencedSet) ImmutableSet.super.addAll(c);
}
@Override
ImmutableSequencedSet addFirst(final @Nullable E element);
@Override
ImmutableSequencedSet addLast(final @Nullable E element);
@Override
ImmutableSequencedSet empty();
@Override
ImmutableSequencedSet remove(E element);
@Override
default ImmutableSequencedSet removeAll(Iterable> c) {
return (ImmutableSequencedSet) ImmutableSet.super.removeAll(c);
}
/**
* Returns a copy of this set that contains all elements
* of this set except the first.
*
* @return a new set instance with the first element removed
* @throws NoSuchElementException if this set is empty
*/
@Override
default ImmutableSequencedSet removeFirst() {
return remove(getFirst());
}
/**
* Returns a copy of this set that contains all elements
* of this set except the last.
*
* @return a new set instance with the last element removed
* @throws NoSuchElementException if this set is empty
*/
@Override
default ImmutableSequencedSet removeLast() {
return remove(getLast());
}
@Override
default ImmutableSequencedSet retainAll(Iterable> c) {
return (ImmutableSequencedSet) ImmutableSet.super.retainAll(c);
}
@Override
SequencedSet toMutable();
/**
* Returns a reversed copy of this set.
*
* This operation may be implemented in O(N).
*
* Use {@link #readOnlyReversed()} if you only
* need to iterate in the reversed sequence over this set.
*
* @return a reversed copy of this set.
*/
default ImmutableSequencedSet reverse() {
if (size() < 2) {
return this;
}
return this.empty().addAll(readOnlyReversed());
}
}