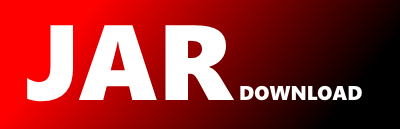
org.jhotdraw8.icollection.serialization.MapSerializationProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jhotdraw8.icollection Show documentation
Show all versions of org.jhotdraw8.icollection Show documentation
JHotDraw8 Immutable Collections
The newest version!
/*
* @(#)MapSerializationProxy.java
* Copyright © 2023 The authors and contributors of JHotDraw. MIT License.
*/
package org.jhotdraw8.icollection.serialization;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serial;
import java.io.Serializable;
import java.util.AbstractMap;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* A serialization proxy that serializes a map independently of its internal
* structure.
*
* Usage:
*
* class MyMap<K, V> implements Map<K, V>, Serializable {
* private final static long serialVersionUID = 0L;
*
* private Object writeReplace() throws ObjectStreamException {
* return new SerializationProxy<>(this);
* }
*
* static class SerializationProxy<K, V>
* extends MapSerializationProxy<K, V> {
* private final static long serialVersionUID = 0L;
* SerializationProxy(Map<K, V> target) {
* super(target);
* }
* {@literal @Override}
* protected Object readResolve() {
* return new MyMap<>(deserializedEntries);
* }
* }
* }
*
*
* References:
*
* - Java Object Serialization Specification: 2 - Object Output Classes,
* 2.5 The writeReplace Method
* - oracle.com
*
* - Java Object Serialization Specification: 3 - Object Input Classes,
* 3.7 The readResolve Method
* - oracle.com
*
*
* @param the key type
* @param the value type
*/
public abstract class MapSerializationProxy implements Serializable {
private final transient Map serialized;
protected transient List> deserializedEntries;
@Serial
private static final long serialVersionUID = 0L;
protected MapSerializationProxy(Map serialized) {
this.serialized = serialized;
}
@Serial
private void writeObject(ObjectOutputStream s)
throws IOException {
s.writeInt(serialized.size());
for (Map.Entry entry : serialized.entrySet()) {
s.writeObject(entry.getKey());
s.writeObject(entry.getValue());
}
}
@Serial
private void readObject(ObjectInputStream s)
throws IOException, ClassNotFoundException {
int n = s.readInt();
deserializedEntries = new ArrayList<>(n);
for (int i = 0; i < n; i++) {
@SuppressWarnings("unchecked")
K key = (K) s.readObject();
@SuppressWarnings("unchecked")
V value = (V) s.readObject();
deserializedEntries.add(new AbstractMap.SimpleImmutableEntry<>(key, value));
}
}
@SuppressWarnings({"serial", "RedundantSuppression"})
// We define this abstract method here, because require that subclasses have this method.
@Serial
protected abstract Object readResolve();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy