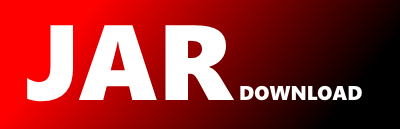
ch.rasc.bsoncodec.AnnotationHierarchyUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bsoncodec-apt Show documentation
Show all versions of bsoncodec-apt Show documentation
Java 8 Annotation Processor creating org.bson.codecs.Codec implementations for POJOs
/**
* Written by Michael Karneim
*
* Part of the project
* https://github.com/mkarneim/pojobuilder
*
* License:
* https://github.com/mkarneim/pojobuilder/blob/master/COPYING
*/
package ch.rasc.bsoncodec;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import javax.lang.model.element.AnnotationMirror;
import javax.lang.model.element.Element;
import javax.lang.model.element.ElementKind;
import javax.lang.model.element.TypeElement;
import javax.lang.model.type.DeclaredType;
import javax.lang.model.util.Types;
public class AnnotationHierarchyUtil {
private final Types types;
public AnnotationHierarchyUtil(Types types) {
this.types = types;
}
public Set filterTriggeringAnnotations(
Set extends TypeElement> aAnnotations, TypeElement bsonDocumentAnnotation) {
Set result = new HashSet<>();
for (TypeElement annoEl : aAnnotations) {
Set hierarchy = getAnnotationHierarchy(annoEl);
if (containsAnnotation(hierarchy, bsonDocumentAnnotation)) {
result.add(annoEl);
}
}
return result;
}
private Set getAnnotationHierarchy(TypeElement annoTypeEl) {
Set result = new HashSet<>();
getAnnotationHierarchy(annoTypeEl, result);
return result;
}
private void getAnnotationHierarchy(TypeElement annoTypeEl, Set result) {
if (result.add(annoTypeEl)) {
List extends AnnotationMirror> annos = annoTypeEl.getAnnotationMirrors();
for (AnnotationMirror anno : annos) {
DeclaredType annoDeclType = anno.getAnnotationType();
Element annoEl = annoDeclType.asElement();
if (annoEl.getKind() == ElementKind.ANNOTATION_TYPE) {
getAnnotationHierarchy((TypeElement) annoEl, result);
}
}
}
}
private boolean containsAnnotation(Set elems, TypeElement annoEl) {
for (TypeElement el : elems) {
if (this.types.isSameType(annoEl.asType(), el.asType())) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy