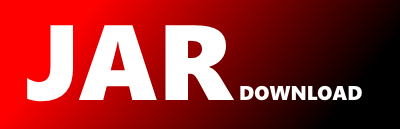
ch.rasc.bsoncodec.model.ImmutableIdModel Maven / Gradle / Ivy
Show all versions of bsoncodec-apt Show documentation
package ch.rasc.bsoncodec.model;
import ch.rasc.bsoncodec.annotation.Id;
import com.google.common.base.MoreObjects;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link IdModel}.
*
* Use the builder to create immutable instances:
* {@code ImmutableIdModel.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "IdModel"})
@Immutable
public final class ImmutableIdModel extends IdModel {
private final @Nullable String generatorName;
private final @Nullable Id.IdConversion conversion;
private final @Nullable String codecName;
private ImmutableIdModel(
@Nullable String generatorName,
@Nullable Id.IdConversion conversion,
@Nullable String codecName) {
this.generatorName = generatorName;
this.conversion = conversion;
this.codecName = codecName;
}
/**
* @return The value of the {@code generatorName} attribute
*/
@Override
public @Nullable String generatorName() {
return generatorName;
}
/**
* @return The value of the {@code conversion} attribute
*/
@Override
public @Nullable Id.IdConversion conversion() {
return conversion;
}
/**
* @return The value of the {@code codecName} attribute
*/
@Override
public @Nullable String codecName() {
return codecName;
}
/**
* Copy the current immutable object by setting a value for the {@link IdModel#generatorName() generatorName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param generatorName A new value for generatorName (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableIdModel withGeneratorName(@Nullable String generatorName) {
if (Objects.equal(this.generatorName, generatorName)) return this;
return new ImmutableIdModel(generatorName, this.conversion, this.codecName);
}
/**
* Copy the current immutable object by setting a value for the {@link IdModel#conversion() conversion} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param conversion A new value for conversion (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableIdModel withConversion(@Nullable Id.IdConversion conversion) {
if (this.conversion == conversion) return this;
return new ImmutableIdModel(this.generatorName, conversion, this.codecName);
}
/**
* Copy the current immutable object by setting a value for the {@link IdModel#codecName() codecName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param codecName A new value for codecName (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableIdModel withCodecName(@Nullable String codecName) {
if (Objects.equal(this.codecName, codecName)) return this;
return new ImmutableIdModel(this.generatorName, this.conversion, codecName);
}
/**
* This instance is equal to all instances of {@code ImmutableIdModel} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableIdModel
&& equalTo((ImmutableIdModel) another);
}
private boolean equalTo(ImmutableIdModel another) {
return Objects.equal(generatorName, another.generatorName)
&& Objects.equal(conversion, another.conversion)
&& Objects.equal(codecName, another.codecName);
}
/**
* Computes a hash code from attributes: {@code generatorName}, {@code conversion}, {@code codecName}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + Objects.hashCode(generatorName);
h = h * 17 + Objects.hashCode(conversion);
h = h * 17 + Objects.hashCode(codecName);
return h;
}
/**
* Prints the immutable value {@code IdModel} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("IdModel")
.omitNullValues()
.add("generatorName", generatorName)
.add("conversion", conversion)
.add("codecName", codecName)
.toString();
}
/**
* Creates an immutable copy of a {@link IdModel} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable IdModel instance
*/
public static ImmutableIdModel copyOf(IdModel instance) {
if (instance instanceof ImmutableIdModel) {
return (ImmutableIdModel) instance;
}
return ImmutableIdModel.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableIdModel ImmutableIdModel}.
* @return A new ImmutableIdModel builder
*/
public static ImmutableIdModel.Builder builder() {
return new ImmutableIdModel.Builder();
}
/**
* Builds instances of type {@link ImmutableIdModel ImmutableIdModel}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private @Nullable String generatorName;
private @Nullable Id.IdConversion conversion;
private @Nullable String codecName;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code IdModel} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(IdModel instance) {
Preconditions.checkNotNull(instance, "instance");
@Nullable String generatorNameValue = instance.generatorName();
if (generatorNameValue != null) {
generatorName(generatorNameValue);
}
@Nullable Id.IdConversion conversionValue = instance.conversion();
if (conversionValue != null) {
conversion(conversionValue);
}
@Nullable String codecNameValue = instance.codecName();
if (codecNameValue != null) {
codecName(codecNameValue);
}
return this;
}
/**
* Initializes the value for the {@link IdModel#generatorName() generatorName} attribute.
* @param generatorName The value for generatorName (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder generatorName(@Nullable String generatorName) {
this.generatorName = generatorName;
return this;
}
/**
* Initializes the value for the {@link IdModel#conversion() conversion} attribute.
* @param conversion The value for conversion (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder conversion(@Nullable Id.IdConversion conversion) {
this.conversion = conversion;
return this;
}
/**
* Initializes the value for the {@link IdModel#codecName() codecName} attribute.
* @param codecName The value for codecName (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder codecName(@Nullable String codecName) {
this.codecName = codecName;
return this;
}
/**
* Builds a new {@link ImmutableIdModel ImmutableIdModel}.
* @return An immutable instance of IdModel
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableIdModel build() {
return new ImmutableIdModel(generatorName, conversion, codecName);
}
}
}