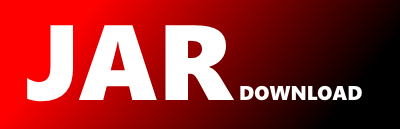
ch.rasc.bsoncodec.model.ImmutableCodecInfo Maven / Gradle / Ivy
package ch.rasc.bsoncodec.model;
import com.google.common.base.MoreObjects;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableSet;
import com.google.common.primitives.Booleans;
import com.squareup.javapoet.TypeName;
import java.util.Set;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.lang.model.element.TypeElement;
/**
* Immutable implementation of {@link CodecInfo}.
*
* Use the static factory method to create immutable instances:
* {@code ImmutableCodecInfo.of()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "CodecInfo"})
@Immutable
public final class ImmutableCodecInfo extends CodecInfo {
private final TypeElement valueType;
private final TypeName codecType;
private final ImmutableSet instanceFields;
private final boolean needRegistryField;
private ImmutableCodecInfo(
TypeElement valueType,
TypeName codecType,
Iterable extends InstanceField> instanceFields,
boolean needRegistryField) {
this.valueType = Preconditions.checkNotNull(valueType, "valueType");
this.codecType = Preconditions.checkNotNull(codecType, "codecType");
this.instanceFields = ImmutableSet.copyOf(instanceFields);
this.needRegistryField = needRegistryField;
}
/**
* @return The value of the {@code valueType} attribute
*/
@Override
public TypeElement valueType() {
return valueType;
}
/**
* @return The value of the {@code codecType} attribute
*/
@Override
public TypeName codecType() {
return codecType;
}
/**
* @return The value of the {@code instanceFields} attribute
*/
@Override
public ImmutableSet instanceFields() {
return instanceFields;
}
/**
* @return The value of the {@code needRegistryField} attribute
*/
@Override
public boolean needRegistryField() {
return needRegistryField;
}
/**
* This instance is equal to all instances of {@code ImmutableCodecInfo} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableCodecInfo
&& equalTo((ImmutableCodecInfo) another);
}
private boolean equalTo(ImmutableCodecInfo another) {
return valueType.equals(another.valueType)
&& codecType.equals(another.codecType)
&& instanceFields.equals(another.instanceFields)
&& needRegistryField == another.needRegistryField;
}
/**
* Computes a hash code from attributes: {@code valueType}, {@code codecType}, {@code instanceFields}, {@code needRegistryField}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + valueType.hashCode();
h = h * 17 + codecType.hashCode();
h = h * 17 + instanceFields.hashCode();
h = h * 17 + Booleans.hashCode(needRegistryField);
return h;
}
/**
* Prints the immutable value {@code CodecInfo} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("CodecInfo")
.omitNullValues()
.add("valueType", valueType)
.add("codecType", codecType)
.add("instanceFields", instanceFields)
.add("needRegistryField", needRegistryField)
.toString();
}
/**
* Construct a new immutable {@code CodecInfo} instance.
* @param valueType The value for the {@code valueType} attribute
* @param codecType The value for the {@code codecType} attribute
* @param instanceFields The value for the {@code instanceFields} attribute
* @param needRegistryField The value for the {@code needRegistryField} attribute
* @return An immutable CodecInfo instance
*/
public static ImmutableCodecInfo of(TypeElement valueType, TypeName codecType, Set instanceFields, boolean needRegistryField) {
return of(valueType, codecType, (Iterable extends InstanceField>) instanceFields, needRegistryField);
}
/**
* Construct a new immutable {@code CodecInfo} instance.
* @param valueType The value for the {@code valueType} attribute
* @param codecType The value for the {@code codecType} attribute
* @param instanceFields The value for the {@code instanceFields} attribute
* @param needRegistryField The value for the {@code needRegistryField} attribute
* @return An immutable CodecInfo instance
*/
public static ImmutableCodecInfo of(TypeElement valueType, TypeName codecType, Iterable extends InstanceField> instanceFields, boolean needRegistryField) {
return new ImmutableCodecInfo(valueType, codecType, instanceFields, needRegistryField);
}
}