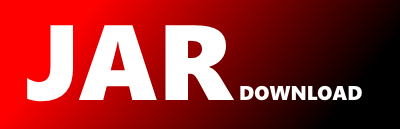
ch.rasc.bsoncodec.model.ImmutableFieldModel Maven / Gradle / Ivy
package ch.rasc.bsoncodec.model;
import ch.rasc.bsoncodec.codegen.CodeGen;
import com.google.common.base.MoreObjects;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import com.google.common.primitives.Booleans;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import javax.lang.model.element.VariableElement;
/**
* Immutable implementation of {@link FieldModel}.
*
* Use the builder to create immutable instances:
* {@code ImmutableFieldModel.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "FieldModel"})
@Immutable
public final class ImmutableFieldModel extends FieldModel {
private final VariableElement varEl;
private final String name;
private final int order;
private final @Nullable String customCodecName;
private final CodeGen codeGen;
private final boolean storeNullValue;
private final boolean storeEmptyCollection;
private final boolean disableEncodeNullCheck;
private final boolean disableDecodeNullCheck;
private final boolean disableSetNullStatement;
private final int fixedArray;
private final @Nullable IdModel idModel;
private final String methodNameSet;
private final String methodNameGet;
private ImmutableFieldModel(ImmutableFieldModel.Builder builder) {
this.varEl = builder.varEl;
this.order = builder.order;
this.customCodecName = builder.customCodecName;
this.codeGen = builder.codeGen;
this.idModel = builder.idModel;
this.methodNameSet = builder.methodNameSet;
this.methodNameGet = builder.methodNameGet;
if (builder.name != null) {
initShim.name(builder.name);
}
if (builder.storeNullValueIsSet()) {
initShim.storeNullValue(builder.storeNullValue);
}
if (builder.storeEmptyCollectionIsSet()) {
initShim.storeEmptyCollection(builder.storeEmptyCollection);
}
if (builder.disableEncodeNullCheckIsSet()) {
initShim.disableEncodeNullCheck(builder.disableEncodeNullCheck);
}
if (builder.disableDecodeNullCheckIsSet()) {
initShim.disableDecodeNullCheck(builder.disableDecodeNullCheck);
}
if (builder.disableSetNullStatementIsSet()) {
initShim.disableSetNullStatement(builder.disableSetNullStatement);
}
if (builder.fixedArrayIsSet()) {
initShim.fixedArray(builder.fixedArray);
}
this.name = initShim.name();
this.storeNullValue = initShim.storeNullValue();
this.storeEmptyCollection = initShim.storeEmptyCollection();
this.disableEncodeNullCheck = initShim.disableEncodeNullCheck();
this.disableDecodeNullCheck = initShim.disableDecodeNullCheck();
this.disableSetNullStatement = initShim.disableSetNullStatement();
this.fixedArray = initShim.fixedArray();
this.initShim = null;
}
private ImmutableFieldModel(
VariableElement varEl,
String name,
int order,
@Nullable String customCodecName,
CodeGen codeGen,
boolean storeNullValue,
boolean storeEmptyCollection,
boolean disableEncodeNullCheck,
boolean disableDecodeNullCheck,
boolean disableSetNullStatement,
int fixedArray,
@Nullable IdModel idModel,
String methodNameSet,
String methodNameGet) {
this.varEl = varEl;
this.name = name;
this.order = order;
this.customCodecName = customCodecName;
this.codeGen = codeGen;
this.storeNullValue = storeNullValue;
this.storeEmptyCollection = storeEmptyCollection;
this.disableEncodeNullCheck = disableEncodeNullCheck;
this.disableDecodeNullCheck = disableDecodeNullCheck;
this.disableSetNullStatement = disableSetNullStatement;
this.fixedArray = fixedArray;
this.idModel = idModel;
this.methodNameSet = methodNameSet;
this.methodNameGet = methodNameGet;
this.initShim = null;
}
private static final int STAGE_INITIALIZING = -1;
private static final int STAGE_UNINITIALIZED = 0;
private static final int STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
private final class InitShim {
private String name;
private int nameBuildStage;
String name() {
if (nameBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (nameBuildStage == STAGE_UNINITIALIZED) {
nameBuildStage = STAGE_INITIALIZING;
this.name = Preconditions.checkNotNull(ImmutableFieldModel.super.name(), "name");
nameBuildStage = STAGE_INITIALIZED;
}
return this.name;
}
void name(String name) {
this.name = name;
nameBuildStage = STAGE_INITIALIZED;
}
private boolean storeNullValue;
private int storeNullValueBuildStage;
boolean storeNullValue() {
if (storeNullValueBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (storeNullValueBuildStage == STAGE_UNINITIALIZED) {
storeNullValueBuildStage = STAGE_INITIALIZING;
this.storeNullValue = ImmutableFieldModel.super.storeNullValue();
storeNullValueBuildStage = STAGE_INITIALIZED;
}
return this.storeNullValue;
}
void storeNullValue(boolean storeNullValue) {
this.storeNullValue = storeNullValue;
storeNullValueBuildStage = STAGE_INITIALIZED;
}
private boolean storeEmptyCollection;
private int storeEmptyCollectionBuildStage;
boolean storeEmptyCollection() {
if (storeEmptyCollectionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (storeEmptyCollectionBuildStage == STAGE_UNINITIALIZED) {
storeEmptyCollectionBuildStage = STAGE_INITIALIZING;
this.storeEmptyCollection = ImmutableFieldModel.super.storeEmptyCollection();
storeEmptyCollectionBuildStage = STAGE_INITIALIZED;
}
return this.storeEmptyCollection;
}
void storeEmptyCollection(boolean storeEmptyCollection) {
this.storeEmptyCollection = storeEmptyCollection;
storeEmptyCollectionBuildStage = STAGE_INITIALIZED;
}
private boolean disableEncodeNullCheck;
private int disableEncodeNullCheckBuildStage;
boolean disableEncodeNullCheck() {
if (disableEncodeNullCheckBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (disableEncodeNullCheckBuildStage == STAGE_UNINITIALIZED) {
disableEncodeNullCheckBuildStage = STAGE_INITIALIZING;
this.disableEncodeNullCheck = ImmutableFieldModel.super.disableEncodeNullCheck();
disableEncodeNullCheckBuildStage = STAGE_INITIALIZED;
}
return this.disableEncodeNullCheck;
}
void disableEncodeNullCheck(boolean disableEncodeNullCheck) {
this.disableEncodeNullCheck = disableEncodeNullCheck;
disableEncodeNullCheckBuildStage = STAGE_INITIALIZED;
}
private boolean disableDecodeNullCheck;
private int disableDecodeNullCheckBuildStage;
boolean disableDecodeNullCheck() {
if (disableDecodeNullCheckBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (disableDecodeNullCheckBuildStage == STAGE_UNINITIALIZED) {
disableDecodeNullCheckBuildStage = STAGE_INITIALIZING;
this.disableDecodeNullCheck = ImmutableFieldModel.super.disableDecodeNullCheck();
disableDecodeNullCheckBuildStage = STAGE_INITIALIZED;
}
return this.disableDecodeNullCheck;
}
void disableDecodeNullCheck(boolean disableDecodeNullCheck) {
this.disableDecodeNullCheck = disableDecodeNullCheck;
disableDecodeNullCheckBuildStage = STAGE_INITIALIZED;
}
private boolean disableSetNullStatement;
private int disableSetNullStatementBuildStage;
boolean disableSetNullStatement() {
if (disableSetNullStatementBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (disableSetNullStatementBuildStage == STAGE_UNINITIALIZED) {
disableSetNullStatementBuildStage = STAGE_INITIALIZING;
this.disableSetNullStatement = ImmutableFieldModel.super.disableSetNullStatement();
disableSetNullStatementBuildStage = STAGE_INITIALIZED;
}
return this.disableSetNullStatement;
}
void disableSetNullStatement(boolean disableSetNullStatement) {
this.disableSetNullStatement = disableSetNullStatement;
disableSetNullStatementBuildStage = STAGE_INITIALIZED;
}
private int fixedArray;
private int fixedArrayBuildStage;
int fixedArray() {
if (fixedArrayBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (fixedArrayBuildStage == STAGE_UNINITIALIZED) {
fixedArrayBuildStage = STAGE_INITIALIZING;
this.fixedArray = ImmutableFieldModel.super.fixedArray();
fixedArrayBuildStage = STAGE_INITIALIZED;
}
return this.fixedArray;
}
void fixedArray(int fixedArray) {
this.fixedArray = fixedArray;
fixedArrayBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
ArrayList attributes = Lists.newArrayList();
if (nameBuildStage == STAGE_INITIALIZING) attributes.add("name");
if (storeNullValueBuildStage == STAGE_INITIALIZING) attributes.add("storeNullValue");
if (storeEmptyCollectionBuildStage == STAGE_INITIALIZING) attributes.add("storeEmptyCollection");
if (disableEncodeNullCheckBuildStage == STAGE_INITIALIZING) attributes.add("disableEncodeNullCheck");
if (disableDecodeNullCheckBuildStage == STAGE_INITIALIZING) attributes.add("disableDecodeNullCheck");
if (disableSetNullStatementBuildStage == STAGE_INITIALIZING) attributes.add("disableSetNullStatement");
if (fixedArrayBuildStage == STAGE_INITIALIZING) attributes.add("fixedArray");
return "Cannot build FieldModel, attribute initializers form cycle" + attributes;
}
}
/**
* @return The value of the {@code varEl} attribute
*/
@Override
public VariableElement varEl() {
return varEl;
}
/**
* @return The value of the {@code name} attribute
*/
@Override
public String name() {
InitShim shim = this.initShim;
return shim != null
? shim.name()
: this.name;
}
/**
* @return The value of the {@code order} attribute
*/
@Override
public int order() {
return order;
}
/**
* @return The value of the {@code customCodecName} attribute
*/
@Override
public @Nullable String customCodecName() {
return customCodecName;
}
/**
* @return The value of the {@code codeGen} attribute
*/
@Override
public CodeGen codeGen() {
return codeGen;
}
/**
* @return The value of the {@code storeNullValue} attribute
*/
@Override
public boolean storeNullValue() {
InitShim shim = this.initShim;
return shim != null
? shim.storeNullValue()
: this.storeNullValue;
}
/**
* @return The value of the {@code storeEmptyCollection} attribute
*/
@Override
public boolean storeEmptyCollection() {
InitShim shim = this.initShim;
return shim != null
? shim.storeEmptyCollection()
: this.storeEmptyCollection;
}
/**
* @return The value of the {@code disableEncodeNullCheck} attribute
*/
@Override
public boolean disableEncodeNullCheck() {
InitShim shim = this.initShim;
return shim != null
? shim.disableEncodeNullCheck()
: this.disableEncodeNullCheck;
}
/**
* @return The value of the {@code disableDecodeNullCheck} attribute
*/
@Override
public boolean disableDecodeNullCheck() {
InitShim shim = this.initShim;
return shim != null
? shim.disableDecodeNullCheck()
: this.disableDecodeNullCheck;
}
/**
* @return The value of the {@code disableSetNullStatement} attribute
*/
@Override
public boolean disableSetNullStatement() {
InitShim shim = this.initShim;
return shim != null
? shim.disableSetNullStatement()
: this.disableSetNullStatement;
}
/**
* @return The value of the {@code fixedArray} attribute
*/
@Override
public int fixedArray() {
InitShim shim = this.initShim;
return shim != null
? shim.fixedArray()
: this.fixedArray;
}
/**
* @return The value of the {@code idModel} attribute
*/
@Override
public @Nullable IdModel idModel() {
return idModel;
}
/**
* @return The value of the {@code methodNameSet} attribute
*/
@Override
public String methodNameSet() {
return methodNameSet;
}
/**
* @return The value of the {@code methodNameGet} attribute
*/
@Override
public String methodNameGet() {
return methodNameGet;
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#varEl() varEl} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for varEl
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withVarEl(VariableElement value) {
if (this.varEl == value) return this;
VariableElement newValue = Preconditions.checkNotNull(value, "varEl");
return new ImmutableFieldModel(
newValue,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#name() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withName(String value) {
if (this.name.equals(value)) return this;
String newValue = Preconditions.checkNotNull(value, "name");
return new ImmutableFieldModel(
this.varEl,
newValue,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#order() order} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for order
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withOrder(int value) {
if (this.order == value) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
value,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#customCodecName() customCodecName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for customCodecName (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withCustomCodecName(@Nullable String value) {
if (Objects.equal(this.customCodecName, value)) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
value,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#codeGen() codeGen} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for codeGen
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withCodeGen(CodeGen value) {
if (this.codeGen == value) return this;
CodeGen newValue = Preconditions.checkNotNull(value, "codeGen");
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
newValue,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#storeNullValue() storeNullValue} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for storeNullValue
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withStoreNullValue(boolean value) {
if (this.storeNullValue == value) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
value,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#storeEmptyCollection() storeEmptyCollection} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for storeEmptyCollection
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withStoreEmptyCollection(boolean value) {
if (this.storeEmptyCollection == value) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
value,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#disableEncodeNullCheck() disableEncodeNullCheck} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for disableEncodeNullCheck
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withDisableEncodeNullCheck(boolean value) {
if (this.disableEncodeNullCheck == value) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
value,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#disableDecodeNullCheck() disableDecodeNullCheck} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for disableDecodeNullCheck
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withDisableDecodeNullCheck(boolean value) {
if (this.disableDecodeNullCheck == value) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
value,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#disableSetNullStatement() disableSetNullStatement} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for disableSetNullStatement
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withDisableSetNullStatement(boolean value) {
if (this.disableSetNullStatement == value) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
value,
this.fixedArray,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#fixedArray() fixedArray} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for fixedArray
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withFixedArray(int value) {
if (this.fixedArray == value) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
value,
this.idModel,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#idModel() idModel} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for idModel (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withIdModel(@Nullable IdModel value) {
if (this.idModel == value) return this;
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
value,
this.methodNameSet,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#methodNameSet() methodNameSet} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for methodNameSet
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withMethodNameSet(String value) {
if (this.methodNameSet.equals(value)) return this;
String newValue = Preconditions.checkNotNull(value, "methodNameSet");
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
newValue,
this.methodNameGet);
}
/**
* Copy the current immutable object by setting a value for the {@link FieldModel#methodNameGet() methodNameGet} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for methodNameGet
* @return A modified copy of the {@code this} object
*/
public final ImmutableFieldModel withMethodNameGet(String value) {
if (this.methodNameGet.equals(value)) return this;
String newValue = Preconditions.checkNotNull(value, "methodNameGet");
return new ImmutableFieldModel(
this.varEl,
this.name,
this.order,
this.customCodecName,
this.codeGen,
this.storeNullValue,
this.storeEmptyCollection,
this.disableEncodeNullCheck,
this.disableDecodeNullCheck,
this.disableSetNullStatement,
this.fixedArray,
this.idModel,
this.methodNameSet,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableFieldModel} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableFieldModel
&& equalTo((ImmutableFieldModel) another);
}
private boolean equalTo(ImmutableFieldModel another) {
return varEl.equals(another.varEl)
&& name.equals(another.name)
&& order == another.order
&& Objects.equal(customCodecName, another.customCodecName)
&& codeGen.equals(another.codeGen)
&& storeNullValue == another.storeNullValue
&& storeEmptyCollection == another.storeEmptyCollection
&& disableEncodeNullCheck == another.disableEncodeNullCheck
&& disableDecodeNullCheck == another.disableDecodeNullCheck
&& disableSetNullStatement == another.disableSetNullStatement
&& fixedArray == another.fixedArray
&& Objects.equal(idModel, another.idModel)
&& methodNameSet.equals(another.methodNameSet)
&& methodNameGet.equals(another.methodNameGet);
}
/**
* Computes a hash code from attributes: {@code varEl}, {@code name}, {@code order}, {@code customCodecName}, {@code codeGen}, {@code storeNullValue}, {@code storeEmptyCollection}, {@code disableEncodeNullCheck}, {@code disableDecodeNullCheck}, {@code disableSetNullStatement}, {@code fixedArray}, {@code idModel}, {@code methodNameSet}, {@code methodNameGet}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + varEl.hashCode();
h = h * 17 + name.hashCode();
h = h * 17 + order;
h = h * 17 + Objects.hashCode(customCodecName);
h = h * 17 + codeGen.hashCode();
h = h * 17 + Booleans.hashCode(storeNullValue);
h = h * 17 + Booleans.hashCode(storeEmptyCollection);
h = h * 17 + Booleans.hashCode(disableEncodeNullCheck);
h = h * 17 + Booleans.hashCode(disableDecodeNullCheck);
h = h * 17 + Booleans.hashCode(disableSetNullStatement);
h = h * 17 + fixedArray;
h = h * 17 + Objects.hashCode(idModel);
h = h * 17 + methodNameSet.hashCode();
h = h * 17 + methodNameGet.hashCode();
return h;
}
/**
* Prints the immutable value {@code FieldModel} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("FieldModel")
.omitNullValues()
.add("varEl", varEl)
.add("name", name)
.add("order", order)
.add("customCodecName", customCodecName)
.add("codeGen", codeGen)
.add("storeNullValue", storeNullValue)
.add("storeEmptyCollection", storeEmptyCollection)
.add("disableEncodeNullCheck", disableEncodeNullCheck)
.add("disableDecodeNullCheck", disableDecodeNullCheck)
.add("disableSetNullStatement", disableSetNullStatement)
.add("fixedArray", fixedArray)
.add("idModel", idModel)
.add("methodNameSet", methodNameSet)
.add("methodNameGet", methodNameGet)
.toString();
}
/**
* Creates an immutable copy of a {@link FieldModel} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable FieldModel instance
*/
public static ImmutableFieldModel copyOf(FieldModel instance) {
if (instance instanceof ImmutableFieldModel) {
return (ImmutableFieldModel) instance;
}
return ImmutableFieldModel.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableFieldModel ImmutableFieldModel}.
* @return A new ImmutableFieldModel builder
*/
public static ImmutableFieldModel.Builder builder() {
return new ImmutableFieldModel.Builder();
}
/**
* Builds instances of type {@link ImmutableFieldModel ImmutableFieldModel}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_VAR_EL = 0x1L;
private static final long INIT_BIT_ORDER = 0x2L;
private static final long INIT_BIT_CODE_GEN = 0x4L;
private static final long INIT_BIT_METHOD_NAME_SET = 0x8L;
private static final long INIT_BIT_METHOD_NAME_GET = 0x10L;
private static final long OPT_BIT_STORE_NULL_VALUE = 0x1L;
private static final long OPT_BIT_STORE_EMPTY_COLLECTION = 0x2L;
private static final long OPT_BIT_DISABLE_ENCODE_NULL_CHECK = 0x4L;
private static final long OPT_BIT_DISABLE_DECODE_NULL_CHECK = 0x8L;
private static final long OPT_BIT_DISABLE_SET_NULL_STATEMENT = 0x10L;
private static final long OPT_BIT_FIXED_ARRAY = 0x20L;
private long initBits = 0x1fL;
private long optBits;
private @Nullable VariableElement varEl;
private @Nullable String name;
private int order;
private @Nullable String customCodecName;
private @Nullable CodeGen codeGen;
private boolean storeNullValue;
private boolean storeEmptyCollection;
private boolean disableEncodeNullCheck;
private boolean disableDecodeNullCheck;
private boolean disableSetNullStatement;
private int fixedArray;
private @Nullable IdModel idModel;
private @Nullable String methodNameSet;
private @Nullable String methodNameGet;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code FieldModel} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(FieldModel instance) {
Preconditions.checkNotNull(instance, "instance");
varEl(instance.varEl());
name(instance.name());
order(instance.order());
@Nullable String customCodecNameValue = instance.customCodecName();
if (customCodecNameValue != null) {
customCodecName(customCodecNameValue);
}
codeGen(instance.codeGen());
storeNullValue(instance.storeNullValue());
storeEmptyCollection(instance.storeEmptyCollection());
disableEncodeNullCheck(instance.disableEncodeNullCheck());
disableDecodeNullCheck(instance.disableDecodeNullCheck());
disableSetNullStatement(instance.disableSetNullStatement());
fixedArray(instance.fixedArray());
@Nullable IdModel idModelValue = instance.idModel();
if (idModelValue != null) {
idModel(idModelValue);
}
methodNameSet(instance.methodNameSet());
methodNameGet(instance.methodNameGet());
return this;
}
/**
* Initializes the value for the {@link FieldModel#varEl() varEl} attribute.
* @param varEl The value for varEl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder varEl(VariableElement varEl) {
this.varEl = Preconditions.checkNotNull(varEl, "varEl");
initBits &= ~INIT_BIT_VAR_EL;
return this;
}
/**
* Initializes the value for the {@link FieldModel#name() name} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link FieldModel#name() name}.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder name(String name) {
this.name = Preconditions.checkNotNull(name, "name");
return this;
}
/**
* Initializes the value for the {@link FieldModel#order() order} attribute.
* @param order The value for order
* @return {@code this} builder for use in a chained invocation
*/
public final Builder order(int order) {
this.order = order;
initBits &= ~INIT_BIT_ORDER;
return this;
}
/**
* Initializes the value for the {@link FieldModel#customCodecName() customCodecName} attribute.
* @param customCodecName The value for customCodecName (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder customCodecName(@Nullable String customCodecName) {
this.customCodecName = customCodecName;
return this;
}
/**
* Initializes the value for the {@link FieldModel#codeGen() codeGen} attribute.
* @param codeGen The value for codeGen
* @return {@code this} builder for use in a chained invocation
*/
public final Builder codeGen(CodeGen codeGen) {
this.codeGen = Preconditions.checkNotNull(codeGen, "codeGen");
initBits &= ~INIT_BIT_CODE_GEN;
return this;
}
/**
* Initializes the value for the {@link FieldModel#storeNullValue() storeNullValue} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link FieldModel#storeNullValue() storeNullValue}.
* @param storeNullValue The value for storeNullValue
* @return {@code this} builder for use in a chained invocation
*/
public final Builder storeNullValue(boolean storeNullValue) {
this.storeNullValue = storeNullValue;
optBits |= OPT_BIT_STORE_NULL_VALUE;
return this;
}
/**
* Initializes the value for the {@link FieldModel#storeEmptyCollection() storeEmptyCollection} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link FieldModel#storeEmptyCollection() storeEmptyCollection}.
* @param storeEmptyCollection The value for storeEmptyCollection
* @return {@code this} builder for use in a chained invocation
*/
public final Builder storeEmptyCollection(boolean storeEmptyCollection) {
this.storeEmptyCollection = storeEmptyCollection;
optBits |= OPT_BIT_STORE_EMPTY_COLLECTION;
return this;
}
/**
* Initializes the value for the {@link FieldModel#disableEncodeNullCheck() disableEncodeNullCheck} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link FieldModel#disableEncodeNullCheck() disableEncodeNullCheck}.
* @param disableEncodeNullCheck The value for disableEncodeNullCheck
* @return {@code this} builder for use in a chained invocation
*/
public final Builder disableEncodeNullCheck(boolean disableEncodeNullCheck) {
this.disableEncodeNullCheck = disableEncodeNullCheck;
optBits |= OPT_BIT_DISABLE_ENCODE_NULL_CHECK;
return this;
}
/**
* Initializes the value for the {@link FieldModel#disableDecodeNullCheck() disableDecodeNullCheck} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link FieldModel#disableDecodeNullCheck() disableDecodeNullCheck}.
* @param disableDecodeNullCheck The value for disableDecodeNullCheck
* @return {@code this} builder for use in a chained invocation
*/
public final Builder disableDecodeNullCheck(boolean disableDecodeNullCheck) {
this.disableDecodeNullCheck = disableDecodeNullCheck;
optBits |= OPT_BIT_DISABLE_DECODE_NULL_CHECK;
return this;
}
/**
* Initializes the value for the {@link FieldModel#disableSetNullStatement() disableSetNullStatement} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link FieldModel#disableSetNullStatement() disableSetNullStatement}.
* @param disableSetNullStatement The value for disableSetNullStatement
* @return {@code this} builder for use in a chained invocation
*/
public final Builder disableSetNullStatement(boolean disableSetNullStatement) {
this.disableSetNullStatement = disableSetNullStatement;
optBits |= OPT_BIT_DISABLE_SET_NULL_STATEMENT;
return this;
}
/**
* Initializes the value for the {@link FieldModel#fixedArray() fixedArray} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link FieldModel#fixedArray() fixedArray}.
* @param fixedArray The value for fixedArray
* @return {@code this} builder for use in a chained invocation
*/
public final Builder fixedArray(int fixedArray) {
this.fixedArray = fixedArray;
optBits |= OPT_BIT_FIXED_ARRAY;
return this;
}
/**
* Initializes the value for the {@link FieldModel#idModel() idModel} attribute.
* @param idModel The value for idModel (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder idModel(@Nullable IdModel idModel) {
this.idModel = idModel;
return this;
}
/**
* Initializes the value for the {@link FieldModel#methodNameSet() methodNameSet} attribute.
* @param methodNameSet The value for methodNameSet
* @return {@code this} builder for use in a chained invocation
*/
public final Builder methodNameSet(String methodNameSet) {
this.methodNameSet = Preconditions.checkNotNull(methodNameSet, "methodNameSet");
initBits &= ~INIT_BIT_METHOD_NAME_SET;
return this;
}
/**
* Initializes the value for the {@link FieldModel#methodNameGet() methodNameGet} attribute.
* @param methodNameGet The value for methodNameGet
* @return {@code this} builder for use in a chained invocation
*/
public final Builder methodNameGet(String methodNameGet) {
this.methodNameGet = Preconditions.checkNotNull(methodNameGet, "methodNameGet");
initBits &= ~INIT_BIT_METHOD_NAME_GET;
return this;
}
/**
* Builds a new {@link ImmutableFieldModel ImmutableFieldModel}.
* @return An immutable instance of FieldModel
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableFieldModel build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableFieldModel(this);
}
private boolean storeNullValueIsSet() {
return (optBits & OPT_BIT_STORE_NULL_VALUE) != 0;
}
private boolean storeEmptyCollectionIsSet() {
return (optBits & OPT_BIT_STORE_EMPTY_COLLECTION) != 0;
}
private boolean disableEncodeNullCheckIsSet() {
return (optBits & OPT_BIT_DISABLE_ENCODE_NULL_CHECK) != 0;
}
private boolean disableDecodeNullCheckIsSet() {
return (optBits & OPT_BIT_DISABLE_DECODE_NULL_CHECK) != 0;
}
private boolean disableSetNullStatementIsSet() {
return (optBits & OPT_BIT_DISABLE_SET_NULL_STATEMENT) != 0;
}
private boolean fixedArrayIsSet() {
return (optBits & OPT_BIT_FIXED_ARRAY) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_VAR_EL) != 0) attributes.add("varEl");
if ((initBits & INIT_BIT_ORDER) != 0) attributes.add("order");
if ((initBits & INIT_BIT_CODE_GEN) != 0) attributes.add("codeGen");
if ((initBits & INIT_BIT_METHOD_NAME_SET) != 0) attributes.add("methodNameSet");
if ((initBits & INIT_BIT_METHOD_NAME_GET) != 0) attributes.add("methodNameGet");
return "Cannot build FieldModel, some of required attributes are not set " + attributes;
}
}
}