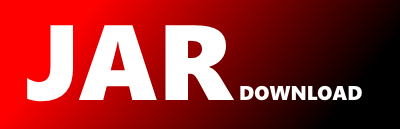
ch.rasc.wampspring.security.WampDestinationMessageMatcher Maven / Gradle / Ivy
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package ch.rasc.wampspring.security;
import org.springframework.messaging.Message;
import org.springframework.security.messaging.util.matcher.MessageMatcher;
import org.springframework.util.AntPathMatcher;
import org.springframework.util.Assert;
import org.springframework.util.PathMatcher;
import ch.rasc.wampspring.message.WampMessage;
import ch.rasc.wampspring.message.WampMessageType;
/**
*
* MessageMatcher which compares a pre-defined pattern against the destination of a
* {@link Message}. There is also support for optionally matching on a specified
* {@link WampMessageType}.
*
*
* @since 4.0
* @author Rob Winch
* @author Ralph Schaer
*/
public final class WampDestinationMessageMatcher implements MessageMatcher
© 2015 - 2025 Weber Informatics LLC | Privacy Policy