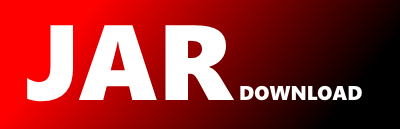
ch.sahits.game.openpatrician.engine.ClockTickTimer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of OpenPatricianEngine Show documentation
Show all versions of OpenPatricianEngine Show documentation
Engine part driving the models and the game
package ch.sahits.game.openpatrician.engine;
import ch.sahits.game.event.data.ClockTickDayChange;
import ch.sahits.game.event.data.IClockTick;
import ch.sahits.game.openpatrician.annotation.ClassCategory;
import ch.sahits.game.openpatrician.annotation.EClassCategory;
import ch.sahits.game.openpatrician.model.Date;
import ch.sahits.game.openpatrician.util.Timer;
import com.google.common.eventbus.AsyncEventBus;
import javafx.application.Platform;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
/**
* @author Andi Hotz, (c) Sahits GmbH, 2015
* Created on Sep 07, 2015
*/
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class ClockTickTimer extends Timer {
private final Logger logger = LogManager.getLogger(getClass());
@Autowired
private Date clock;
@Autowired
@Qualifier("serverEventBus")
private AsyncEventBus serverEventBus;
@Autowired
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
/** Lock for guaranteeing thread safety */
private static Object lock = new Object();
public ClockTickTimer(long interval, long duration) {
super(interval, duration);
}
@Override
protected void onTick() {
tick();
serverEventBus.post(new IClockTick(){});
}
@Override
protected void onFinish() {
}
/**
* Update the time by one tick.
*/
public void tick(){
synchronized (lock) {
int dayBefore = clock.getCurrentDate().getDayOfMonth();
clock.updateTime(clock.getCurrentDate().plusMinutes(clock.getTickUpdate()));
if (dayBefore!=clock.getCurrentDate().getDayOfMonth()){
Platform.runLater(() ->
clock.dayDateBinding().invalidate());
final ClockTickDayChange event = new ClockTickDayChange();
clientServerEventBus.post(event);
logger.info("Day change");
}
}
clientServerEventBus.post(new ch.sahits.game.event.data.ClockTick());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy