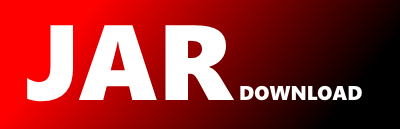
ch.sahits.game.openpatrician.engine.event.task.ShipBuildTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of OpenPatricianEngine Show documentation
Show all versions of OpenPatricianEngine Show documentation
Engine part driving the models and the game
package ch.sahits.game.openpatrician.engine.event.task;
import ch.sahits.game.openpatrician.annotation.ClassCategory;
import ch.sahits.game.openpatrician.annotation.EClassCategory;
import ch.sahits.game.openpatrician.annotation.Prototype;
import ch.sahits.game.openpatrician.model.DisplayMessage;
import ch.sahits.game.openpatrician.model.IPlayer;
import ch.sahits.game.openpatrician.model.city.impl.IShipBuildTask;
import ch.sahits.game.openpatrician.model.event.TimedTask;
import ch.sahits.game.openpatrician.model.ship.EShipType;
import ch.sahits.game.openpatrician.model.ship.IShip;
import ch.sahits.game.openpatrician.model.ship.ShipFactory;
import ch.sahits.game.openpatrician.util.RandomNameLoader;
import ch.sahits.game.openpatrician.util.l10n.Locale;
import com.google.common.eventbus.AsyncEventBus;
import com.thoughtworks.xstream.annotations.XStreamOmitField;
import org.joda.time.DateTime;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.MessageSource;
import javax.annotation.PostConstruct;
/**
* @author Andi Hotz, (c) Sahits GmbH, 2014
* Created on Feb 23, 2014
*/
@Prototype
@ClassCategory(EClassCategory.DEPENDS_ON_SERIALIZED_BEAN)
class ShipBuildTask extends TimedTask implements IShipBuildTask {
private final EShipType type;
private final IPlayer owner;
@Autowired
@XStreamOmitField
private ShipFactory shipUtility;
private final double x;
private IShip ship;
private static RandomNameLoader shipLoader = new RandomNameLoader("shipnames.properties");
@Autowired
@XStreamOmitField
private Locale locale;
@Autowired
@XStreamOmitField
private MessageSource messageSource;
@Autowired
@Qualifier("serverClientEventBus")
@XStreamOmitField
private AsyncEventBus clientServerEventBus;
public ShipBuildTask(DateTime executionTime, EShipType type, IPlayer owner, double westPosition) {
super();
setExecutionTime(executionTime);
this.owner = owner;
this.type = type;
x = westPosition;
}
@PostConstruct
void initializeShip() {
ship = createShip();
}
@Override
public void run() {
DisplayMessage msg = new DisplayMessage(messageSource.getMessage("ch.sahits.game.openpatrician.engine.event.task.ShipBuildTask.message", new Object[]{type, ship.getName()}, locale.getCurrentLocal()));
clientServerEventBus.post(msg);
}
/**
* Retrieve the ship that is to be built.
* @return
*/
@Override
public IShip getShipToBeBuilt(){
return ship;
}
/**
* Create the ship based on the ships type.
* @return
*/
private IShip createShip() {
IShip ship = null;
switch (type) {
case SNAIKKA:
ship = shipUtility.createSnaikka(shipLoader.getRandomName(), calculateShipCapacity());
break;
case CRAYER:
ship = shipUtility.createCrayer(shipLoader.getRandomName(), calculateShipCapacity());
break;
case COG:
ship = shipUtility.createCog(shipLoader.getRandomName(), calculateShipCapacity());
break;
case HOLK:
ship = shipUtility.createHolk(shipLoader.getRandomName(), calculateShipCapacity());
break;
}
ship.setOwner(owner);
return ship;
}
private int calculateShipCapacity() {
return shipUtility.calculateInitialCapacity(type, (int)x);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy