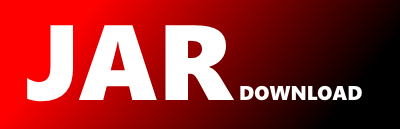
ch.sahits.game.openpatrician.engine.land.city.ChurchEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of OpenPatricianEngine Show documentation
Show all versions of OpenPatricianEngine Show documentation
Engine part driving the models and the game
package ch.sahits.game.openpatrician.engine.land.city;
import ch.sahits.game.event.data.PeriodicalTimeWeekEndUpdate;
import ch.sahits.game.openpatrician.annotation.ClassCategory;
import ch.sahits.game.openpatrician.annotation.EClassCategory;
import ch.sahits.game.openpatrician.annotation.LazySingleton;
import ch.sahits.game.openpatrician.engine.AbstractEngine;
import ch.sahits.game.openpatrician.engine.EngineFactory;
import ch.sahits.game.openpatrician.model.Date;
import ch.sahits.game.openpatrician.model.city.EChurchExtension;
import ch.sahits.game.openpatrician.model.city.IChurch;
import ch.sahits.game.openpatrician.model.city.ICity;
import ch.sahits.game.openpatrician.model.collection.CityChurchRegistry;
import ch.sahits.game.openpatrician.model.event.TimedTask;
import ch.sahits.game.openpatrician.model.event.TimedUpdatableTaskList;
import ch.sahits.game.openpatrician.model.factory.StateFactory;
import com.google.common.eventbus.AsyncEventBus;
import com.google.common.eventbus.Subscribe;
import org.joda.time.DateTime;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import java.util.Collections;
import java.util.List;
import java.util.Random;
/**
* @author Andi Hotz, (c) Sahits GmbH, 2015
* Created on Jun 21, 2015
*/
@LazySingleton
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class ChurchEngine extends AbstractEngine {
@Autowired
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
@Autowired
private Random rnd;
@Autowired
private Date date;
@Autowired
private TimedUpdatableTaskList timedTaskListener;
@Autowired
private EngineFactory engineFactory;
@Autowired
private StateFactory stateFactory;
@Autowired
private CityChurchRegistry churchMap;
@PostConstruct
private void init() {
clientServerEventBus.register(this);
}
@PreDestroy
private void unregister() {
clientServerEventBus.unregister(this);
}
@Override
public List getChildren() {
return Collections.EMPTY_LIST;
}
@Subscribe
public void handleWeeklyUpdate(PeriodicalTimeWeekEndUpdate event) {
// check if an upgrade can be made
for (ICity iCity : churchMap.keySet()) {
IChurch church = getChurch(iCity);
if (church.getExtensionLevel().getNextExtensionLevel().isPresent()) {
EChurchExtension next = church.getExtensionLevel().getNextExtensionLevel().get();
final int collectedDonationsForExtension = church.getCollectedDonationsForExtension();
if (collectedDonationsForExtension >= next.getRequiredCash()) {
// start upgrade
int delay = rnd.nextInt(30);
DateTime executionTime = date.getCurrentDate().plusDays(delay);
TimedTask task = new TimedTask() {
{
super.setExecutionTime(executionTime);
}
@Override
public void run() {
church.upgrade();
}
};
timedTaskListener.add(task);
}
}
}
}
public void establishChurch(ICity city) {
IChurch church = stateFactory.createChurch();
churchMap.put(city, church);
}
public IChurch getChurch(ICity city) {
return churchMap.get(city);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy