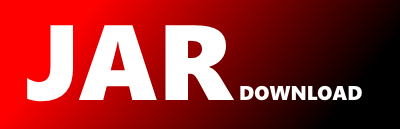
ch.sahits.game.openpatrician.engine.land.city.CityEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of OpenPatricianEngine Show documentation
Show all versions of OpenPatricianEngine Show documentation
Engine part driving the models and the game
package ch.sahits.game.openpatrician.engine.land.city;
import ch.sahits.game.event.data.ClockTick;
import ch.sahits.game.event.data.ClockTickIntervalChange;
import ch.sahits.game.openpatrician.annotation.ClassCategory;
import ch.sahits.game.openpatrician.annotation.DependentInitialisation;
import ch.sahits.game.openpatrician.annotation.EClassCategory;
import ch.sahits.game.openpatrician.engine.AbstractEngine;
import ch.sahits.game.openpatrician.engine.player.PlayerEngine;
import ch.sahits.game.openpatrician.model.Date;
import ch.sahits.game.openpatrician.model.IGame;
import ch.sahits.game.openpatrician.model.city.ICity;
import ch.sahits.game.openpatrician.model.city.impl.CitiesState;
import ch.sahits.game.openpatrician.model.city.impl.CityState;
import ch.sahits.game.openpatrician.util.MapInitializedBean;
import ch.sahits.game.openpatrician.util.javafx.IJavaFXApplicationThreadExecution;
import com.google.common.eventbus.AsyncEventBus;
import com.google.common.eventbus.Subscribe;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Component;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import static com.google.common.collect.Lists.newArrayList;
/**
* The CityEngine drives/generates the events that are based in the city.
* Mainly these are:
*
* - Consume of wares
* - Production of wares from workshops owned by the city
*
* @author Andi Hotz, (c) Sahits GmbH, 2011
* Created on Nov 28, 2011
*
*/
@Component
@Lazy
@DependentInitialisation(MapInitializedBean.class)
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class CityEngine extends AbstractEngine {
@Autowired
private Random rnd;
@Autowired
private ApplicationContext context;
@Autowired
private ShipyardEngine shipyardEngine;
/** Update the ware consumed and produced every 12h */
private final static int WARE_UPDATES_MINUTES = 12*60;
/** Number of ticks that need to waited untill {@link #WARE_UPDATES_MINUTES} */
private int numberOfTicks;
/** Counter that counts the ticks */
private int tickCounter = 0;
/** State indicating that the engine is not ready */
private final static byte STOPPED = 0x00;
/** State indicating that the engine is properly initialized */
private final static byte STARTED = 0x01;
/** State of the engine */
private byte state = STOPPED;
@Autowired
private Date date;
@Autowired
private CitiesState citiesState;
@Autowired
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
@Autowired
@Qualifier("serverEventBus")
private AsyncEventBus serverEventBus;
@Autowired
@Qualifier("javaFXApplicationThreadExecution")
private IJavaFXApplicationThreadExecution threadExecutor;
@Autowired
private AutomaticTradingEngine automaticTradingEngine;
@Autowired
private LoanerEngine loanerEngine;
@Autowired
private CityHallEngine cityHallEngine;
@Autowired
private PlayerEngine playerEngine;
@Autowired
private ChurchEngine churchEngine;
@Autowired
private TavernEngine tavernEngine;
@PostConstruct
public void initialize() {
numberOfTicks = WARE_UPDATES_MINUTES/date.getTickUpdate();
clientServerEventBus.register(this);
}
@PreDestroy
private void unregister() {
clientServerEventBus.unregister(this);
}
@Subscribe
public void handleClockTickIntervallChange(ClockTickIntervalChange event) {
if (event.getInterval() == 0) {
numberOfTicks = -1;
} else {
numberOfTicks = WARE_UPDATES_MINUTES / event.getInterval();
}
}
// for Test purposes
int getNumberOfTicks() {
return numberOfTicks;
}
@Subscribe
public void handleClockTicked(ClockTick event) {
tickCounter++;
if (tickCounter==numberOfTicks && state==STARTED){
tickCounter=0;
threadExecutor.execute(new Runnable() {
@Override
public void run() {
produceAndConsumeWares();
}
});
}
}
/**
* Compute the consume and production of wares of each city
*/
private void produceAndConsumeWares() {
for (CityState state : citiesState.getCityEngineStates()) {
state.consumeWares();
state.produceWares();
}
}
/**
* Add a new city to the engine
* @param city
*/
private void addCity(ICity city){
CityState cityState = city.getCityState();
tavernEngine.addCity(cityState.getTavernState(), city);
loanerEngine.addNewLoaner(city);
cityHallEngine.establishCityHall(city);
churchEngine.establishChurch(city);
}
/**
* Start the engine. This should be called after all cities were added
*/
public void start(IGame game){
for (ICity city : game.getMap().getCities()) {
addCity(city);
}
state=STARTED;
}
@Override
public List getChildren() {
ArrayList engines = newArrayList();
engines.add(tavernEngine);
engines.add(shipyardEngine);
engines.add(automaticTradingEngine);
engines.add(loanerEngine);
engines.add(cityHallEngine);
engines.add(playerEngine);
engines.add(churchEngine);
return engines;
}
public ShipyardEngine getShipyardEngine() {
return shipyardEngine;
}
/**
* Find the tafern engine for the matching city.
* @return
*/
public TavernEngine findTavernEngine() {
return tavernEngine;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy