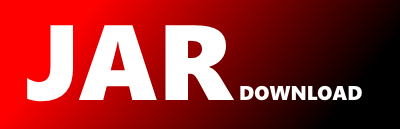
ch.sahits.game.openpatrician.engine.land.city.OutriggerService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of OpenPatricianEngine Show documentation
Show all versions of OpenPatricianEngine Show documentation
Engine part driving the models and the game
package ch.sahits.game.openpatrician.engine.land.city;
import ch.sahits.game.openpatrician.annotation.ClassCategory;
import ch.sahits.game.openpatrician.annotation.EClassCategory;
import ch.sahits.game.openpatrician.annotation.LazySingleton;
import ch.sahits.game.openpatrician.model.Date;
import ch.sahits.game.openpatrician.model.IMap;
import ch.sahits.game.openpatrician.model.city.ICity;
import ch.sahits.game.openpatrician.model.ship.IShip;
import ch.sahits.game.openpatrician.model.weapon.EWeapon;
import org.springframework.beans.factory.annotation.Autowired;
/**
* Service calculationg various values around a cities outrigger.
* @author Andi Hotz, (c) Sahits GmbH, 2015
* Created on Mar 21, 2015
*/
@LazySingleton
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class OutriggerService {
@Autowired
private Date date;
@Autowired
private IMap map;
/**
* Calculate the required weapons strength for a city.
* @param city
* @return
*/
public int getRequiredWeaponStrength(ICity city) {
double xLocation = city.getCoordinates().getX();
// 2 at the right border and 1 at the left border
double westwardFactor = 1 + (map.getDimension().getWidth() - xLocation) / map.getDimension().getWidth();
int currentYear = date.getCurrentDate().getYear();
if (currentYear <= 1200) {
return (int)westwardFactor;
}
int from1200 = currentYear - 1200;
double yearFactor = from1200*6.0/200;
return (int)(westwardFactor*yearFactor);
}
/**
* Calculate the weekly premium.
* @param city
* @return
*/
public int getWeeklyReund(ICity city) {
double xLocation = city.getCoordinates().getX();
// 2 at the right border and 1 at the left border
double westwardFactor = 1 + (map.getDimension().getWidth() - xLocation) / map.getDimension().getWidth();
int currentYear = date.getCurrentDate().getYear();
if (currentYear <= 1200) {
return (int)westwardFactor*30;
}
int from1200 = currentYear - 1200;
double yearFactor = from1200*6.0/200;
return (int)(westwardFactor*yearFactor)*30;
}
public int calculateShipsWeaponsStrength(IShip ship) {
int strength = 0;
strength += ship.getWeaponAmount(EWeapon.BALLISTA_BIG) * 2;
strength += ship.getWeaponAmount(EWeapon.TREBUCHET_BIG) * 2;
strength += ship.getWeaponAmount(EWeapon.CANNON) * 2;
strength += ship.getWeaponAmount(EWeapon.BOMBARD) * 2;
strength += ship.getWeaponAmount(EWeapon.BALLISTA_SMALL);
strength += ship.getWeaponAmount(EWeapon.TREBUCHET_SMALL);
return strength;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy