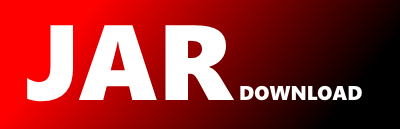
ch.sahits.game.openpatrician.engine.land.city.ShipyardEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of OpenPatricianEngine Show documentation
Show all versions of OpenPatricianEngine Show documentation
Engine part driving the models and the game
package ch.sahits.game.openpatrician.engine.land.city;
import ch.sahits.game.event.data.ShipyardOrderBuild;
import ch.sahits.game.event.data.ShipyardOrderRefit;
import ch.sahits.game.event.data.ShipyardOrderRepair;
import ch.sahits.game.openpatrician.annotation.ClassCategory;
import ch.sahits.game.openpatrician.annotation.DependentInitialisation;
import ch.sahits.game.openpatrician.annotation.EClassCategory;
import ch.sahits.game.openpatrician.client.ICityPlayerProxyJFX;
import ch.sahits.game.openpatrician.engine.AbstractEngine;
import ch.sahits.game.openpatrician.model.Date;
import ch.sahits.game.openpatrician.model.IPlayer;
import ch.sahits.game.openpatrician.model.city.IShipDueDate;
import ch.sahits.game.openpatrician.model.city.IShipyard;
import ch.sahits.game.openpatrician.model.city.impl.IShipBuildTask;
import ch.sahits.game.openpatrician.model.city.impl.ShipDueDate;
import ch.sahits.game.openpatrician.model.city.impl.ShipyardState;
import ch.sahits.game.openpatrician.model.event.TimedTask;
import ch.sahits.game.openpatrician.model.event.TimedUpdatableTaskList;
import ch.sahits.game.openpatrician.model.ship.EShipType;
import ch.sahits.game.openpatrician.model.ship.EShipUpgrade;
import ch.sahits.game.openpatrician.model.ship.IShip;
import ch.sahits.game.openpatrician.model.ship.ShipFactory;
import ch.sahits.game.openpatrician.engine.event.task.ServerSideTaskFactory;
import ch.sahits.game.openpatrician.util.StartNewGameBean;
import java.util.Optional;
import com.google.common.eventbus.AsyncEventBus;
import com.google.common.eventbus.Subscribe;
import org.joda.time.DateTime;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Service;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import static com.google.common.collect.Lists.newArrayList;
/**
* The shipyard engine handled the activities on a shipyard.
* This engine is stateless, so it requires the IShipyard to work on.
* @author Andi Hotz, (c) Sahits GmbH, 2014
* Created on Mar 01, 2014
*/
@Service
@Lazy
@DependentInitialisation(StartNewGameBean.class)
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class ShipyardEngine extends AbstractEngine {
@Autowired
private Date date;
@Autowired
private Random rnd;
@Autowired
private TimedUpdatableTaskList taskList;
@Autowired
private ShipFactory shipUtility;
@Autowired
private ServerSideTaskFactory taskFactory;
@Autowired
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
@PostConstruct
private void initializeEventBus() {
clientServerEventBus.register(this);
}
@PreDestroy
private void unregister() {
clientServerEventBus.unregister(this);
}
/**
* Order a ship of given type to be build. The costs are not deduced.
* This method is called when the ship building is actually ordered.
* @param type of the ship to build
* @param owner of the ship to be built
* @param shipyard reference to the state
* @return date when the ship will be finished.
*/
private DateTime orderShipBuilding(IShipyard shipyard, EShipType type, IPlayer owner) {
((ShipyardState)shipyard).cleanup();
int buildTime = shipyard.calculateBuildTime(type);
DateTime startDate = date.getCurrentDate();
for (Iterator iterator = shipyard.getShipBuildingList().iterator(); iterator.hasNext();) {
IShipDueDate dueDate = iterator.next();
if (dueDate.getDueDate().isAfter(startDate)) {
startDate = dueDate.getDueDate();
}
}
DateTime finished = startDate.plusDays(buildTime);
TimedTask task = (TimedTask) taskFactory.getShipBuildTask(finished, type, owner, shipyard.getCity().getCoordinates().getX());
IShipDueDate dueDate = new ShipDueDate(((IShipBuildTask)task).getShipToBeBuilt(), finished);
shipyard.addShipBuildingOrder(dueDate, Optional.of(task));
taskList.add(task);
return finished;
}
/**
* Order the repair of the ship. The costs for the repair not are deduced.
* With each repair order the crew gets more expierianced.
* @param ship to be repaired.
* @param shipyard reference to the state
*/
private void repair(IShipyard shipyard, IShip ship, ICityPlayerProxyJFX proxy) {
((ShipyardState)shipyard).cleanup();
int repairTime = shipyard.calculateBuildTime(ship.getShipType());
int damageInv = ship.getDamage();
repairTime = Math.max((int) (repairTime * Math.max(damageInv, 0.05)), 1);
ShipDueDate dueDate = new ShipDueDate(ship, date.getCurrentDate().plusDays(repairTime));
TimedTask task = taskFactory.getRepairTask(dueDate.getDueDate(), ship, proxy);
taskList.add(task);
ship.setAvailable(false);
shipyard.addShipRepairOrder(dueDate, Optional.of(task));
}
/**
* Refit the ship to the new level. The costs for the upgrade are not deduced.
* @param ship to be refitted
* @param upgradeLevel level to which the ship should be upgraded.
* @param shipyard reference to the state
*/
private void refit(IShipyard shipyard, IShip ship, EShipUpgrade upgradeLevel, ICityPlayerProxyJFX proxy) {
((ShipyardState)shipyard).cleanup();
int refitTime = shipyard.calculateRefitTime(ship.getShipType());
EShipUpgrade level = ship.getShipUpgradeLevel();
int indexStartLevel = 0;
int indexFinalLevel = 0;
for (int i = 0; i < EShipUpgrade.values().length; i++) {
if (EShipUpgrade.values()[i] == level) {
indexStartLevel = i;
}
if (EShipUpgrade.values()[i] == upgradeLevel) {
indexFinalLevel = i;
break; // the final level has to be larger than the start level
}
}
int nbLevelUpgrades = Math.max(indexFinalLevel-indexStartLevel,0);
ShipDueDate dueDate = new ShipDueDate(ship, date.getCurrentDate().plusDays(refitTime*nbLevelUpgrades));
TimedTask task = taskFactory.getRefitShipTask(dueDate.getDueDate(), ship, upgradeLevel, proxy);
taskList.add(task);
ship.setAvailable(false);
shipyard.addShipRefitOrder(dueDate, Optional.of(task));
}
@Override
public List getChildren() {
return newArrayList();
}
/**
* Recieve the event from the event bus and delegate it.
* @param event
*/
@Subscribe
public void handleOrderBuildEvent(ShipyardOrderBuild event) {
orderShipBuilding(event.getShipyard(), event.getShipType(), event.getOwner());
}
/**
* Recieve the event from the event bus and delegate it.
* @param event
*/
@Subscribe
public void handleOrderRepair(ShipyardOrderRepair event) {
repair(event.getShipyard(), event.getShip(), event.getProxy());
}
/**
* Recieve the event from the event bus and delegate it.
* @param event
*/
@Subscribe
public void handleORderRefit(ShipyardOrderRefit event) {
refit(event.getShipyard(), event.getShip(), event.getUpgradeLevel(), event.getProxy());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy