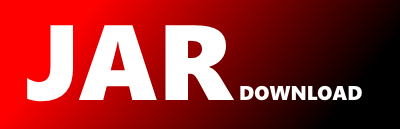
ch.sahits.game.openpatrician.engine.event.task.AIRefitTask Maven / Gradle / Ivy
package ch.sahits.game.openpatrician.engine.event.task;
import ch.sahits.game.openpatrician.clientserverinterface.service.ShipService;
import ch.sahits.game.openpatrician.event.data.RefitFinishedEvent;
import ch.sahits.game.openpatrician.model.city.IShipyard;
import ch.sahits.game.openpatrician.model.event.TimedTask;
import ch.sahits.game.openpatrician.model.ship.EShipUpgrade;
import ch.sahits.game.openpatrician.model.ship.IShip;
import ch.sahits.game.openpatrician.model.ship.IWeaponSlot;
import ch.sahits.game.openpatrician.model.ship.SecondaryLargeWeaponSlot;
import ch.sahits.game.openpatrician.model.ship.impl.ShipWeaponsLocationFactory;
import ch.sahits.game.openpatrician.model.weapon.EWeapon;
import ch.sahits.game.openpatrician.utilities.annotation.ClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.EClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.Prototype;
import com.google.common.eventbus.AsyncEventBus;
import com.thoughtworks.xstream.annotations.XStreamOmitField;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import java.time.LocalDateTime;
import java.util.List;
/**
* @author Andi Hotz, (c) Sahits GmbH, 2014
* Created on Feb 23, 2014
*/
@Prototype
@ClassCategory({EClassCategory.SERIALIZABLE_BEAN, EClassCategory.PROTOTYPE_BEAN})
class AIRefitTask extends TimedTask {
@XStreamOmitField
private static final Logger LOGGER = LogManager.getLogger(AIRefitTask.class);
@Autowired
@XStreamOmitField
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
@Autowired
@XStreamOmitField
private ShipWeaponsLocationFactory shipWeaponLocationFactory;
@Autowired
@XStreamOmitField
private ShipService shipService;
private final IShip ship;
private final EShipUpgrade level;
private final IShipyard shipyard;
public AIRefitTask(LocalDateTime executionTime, IShip ship, EShipUpgrade upgrade, IShipyard shipyard) {
super();
setExecutionTime(executionTime);
LOGGER.debug("Add AIRefitTask for {} to be done at {}", ship.getName(), executionTime);
this.ship = ship;
this.level = upgrade;
this.shipyard = shipyard;
}
@Override
public void run() {
EShipUpgrade currentLevel = ship.getShipUpgradeLevel();
while (currentLevel != level) {
ship.upgrade();
List oldWeaponSlots = ship.getWeaponSlots();
List newWeaponSlots = shipWeaponLocationFactory.getShipWeaponsLocation(ship.getShipType(), level);
ship.setWeaponSlots(newWeaponSlots);
for (IWeaponSlot slot : oldWeaponSlots) {
if (slot.getWeapon().isPresent()) {
EWeapon weapon = (EWeapon) slot.getWeapon().get();
if (slot instanceof SecondaryLargeWeaponSlot) {
if (!shipService.isLargeWeapon(weapon)) { // ignore large weapons in secondary slots
shipService.placeWeapon(weapon, ship);
}
} else {
// Not secondary slot
shipService.placeWeapon(weapon, ship);
}
}
}
currentLevel = ship.getShipUpgradeLevel();
}
ship.setAvailable(true);
shipyard.removeCompletedUpgrade(ship);
LOGGER.debug("Refited ship {}", ship.getName());
clientServerEventBus.post(new RefitFinishedEvent(ship));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy