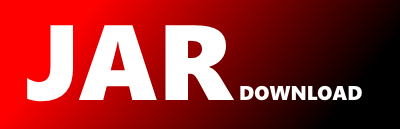
ch.sahits.game.openpatrician.engine.event.task.RepairPirateShipTask Maven / Gradle / Ivy
package ch.sahits.game.openpatrician.engine.event.task;
import ch.sahits.game.openpatrician.event.data.RepairFinishedEvent;
import ch.sahits.game.openpatrician.model.city.IShipyard;
import ch.sahits.game.openpatrician.model.event.TimedTask;
import ch.sahits.game.openpatrician.model.people.ISeaPirate;
import ch.sahits.game.openpatrician.model.ship.INavigableVessel;
import ch.sahits.game.openpatrician.model.ship.IShip;
import ch.sahits.game.openpatrician.model.ship.IShipGroup;
import ch.sahits.game.openpatrician.utilities.annotation.ClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.EClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.Prototype;
import com.google.common.base.Preconditions;
import com.google.common.eventbus.AsyncEventBus;
import com.thoughtworks.xstream.annotations.XStreamOmitField;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import java.time.LocalDateTime;
/**
* Special implementation for the repair task for pirate ships,
* which do not have a player as owner and may be ordered to
* repair as a whole group
*/
@Prototype
@ClassCategory({EClassCategory.SERIALIZABLE_BEAN, EClassCategory.PROTOTYPE_BEAN})
public class RepairPirateShipTask extends TimedTask {
@Autowired
@XStreamOmitField
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
private final INavigableVessel vessel;
private final IShipyard shipyard;
public RepairPirateShipTask(LocalDateTime executionTime, INavigableVessel vessel, ISeaPirate pirate, IShipyard shipyard) {
Preconditions.checkArgument(vessel instanceof IShip || vessel instanceof IShipGroup);
setExecutionTime(executionTime);
this.vessel = vessel;
this.shipyard = shipyard;
}
@Override
public void run() {
if (vessel instanceof IShip) {
IShip ship = (IShip) vessel;
executeRepair(ship);
} else {
for (IShip ship : ((IShipGroup)vessel).getShips()) {
executeRepair(ship);
}
}
clientServerEventBus.post(new RepairFinishedEvent(vessel));
}
private void executeRepair(IShip ship) {
ship.repair();
shipyard.removeCompletedRepair(ship);
ship.setAvailable(true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy